日期时间类
Date类用于表示日期时间,它位于Java.util包中。
创建Date类的对象
Date类最简单的构造方法就是默认的无参的Date()构造方法,它使用系统中当前日期和时间创建并初始化Date类的实例对象。
例如:Date now=new Date();
Date类的另一个构造方法是Date(long date),这个构造方法接收一个long类型的整数来初始化Date对象。System类的currenTimeMillis()方法可以获取系统当前时间距历元(1970年1月1日00:00:00开始)的豪秒数。
例如:long timeMillis=System.currentTimeMillis();
Date date=new Date(timeMillis);
比较Date对象
1. after()方法
该方法用于测试此日期对象是否在指定日期之后。
方法声明:public boolean after(Date when)
When:该参数是要比较的日期对象。
仅当此Date对象表示的时间比when表示的时间晚,才返回true;否则返回false。
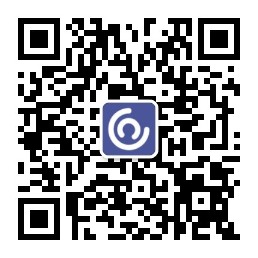
例 15.01 创建两个不同时间的Date对象,判断这个对象表示的时间哪个在前哪个在后。
import java.util.Date; public class Demo{ public static void main(String[] args){ //比当前时间快5秒 long timeMillis=System.currentTimeMillis()+5000; Date now=new Date();//当前时间 Date otherDate=new Date(timeMillis); if(otherDate.after(now)) System.out.println("otherDate在now之后"); else System.out.println("otherDate在now之前"); } } |
运行结果:
otherDate在now之后
2. Before()方法
该方法用于测试此日期对象是否在指定日期之前,它和after()方法正好相反。
方法声明:public boolean before(Date when)
When:该参数是要比较的日期对象
仅当此Date对象表示的时间比when表示的时间早,才返回true;否则返回false。
3. compareTo()方法
该方法用于比较两个日期对象的顺序,它常用于多个Date对象的排序。
方法声明:public int compareTo(Date anotherDate)
anotherDate:该参数是要比较其他日期对象
如果参数anotherDate表示的时间等于当前Date对象表示的时间,该方法返回值为0;如果当前Date对象表示的时间在anotherDate参数表示的时间前,则返回小于0的值;如果当前Date对象在anotherDate参数表示的时间之后,则返回大于0的值。
例 15.02 创建两个不同时间的Date对象,比较两个对象。
import java.util.Date; public class Demo{ public static void main(String[] args){ //比当前时间快5秒 long timeMillis=System.currentTimeMillis()-5000; Date now=new Date();//当前时间 Date otherDate=new Date(timeMillis); int compare=otherDate.compareTo(now); switch (compare) { case 0: System.out.println("两个日期对象表示的时间相等"); break; case 1: System.out.println("otherDate对象表示的时间大于now对象表示的时间"); break; case -1: System.out.println("otherDate对象表示的时间小于now对象表示的时间"); break; default: break; } } } |
运行结果:
otherDate对象表示的时间小于now对象表示的时间
更改Date对象
1. getTime()方法
该方法用于获取此Date对象的豪秒数值。
方法声明:public long getTime()
2. setTime()方法
该方法用于设置此Date对象。
方法声明:public void setTime(long time)
日期和时间格式化输出
String类的静态format()方法通过特殊转义符作为参数可以实现对日期和时间的格式化。
1. 日期格式化
对于日期的格式化,可以使用转义符,把Date类的实例化对象格式化。
例如:返回年月日中的日。
import java.util.Date; public class Demo{ public static void main(String[] args){ Date date=new Date();//创建Date对象 //通过format()方法对Date进行格式化 String day=String.format("%te", date); System.out.println("day="+day); } } |
运行结果:
day=21
例 15.03 日期格式化输出
import java.util.Date; public class Demo{ public static void main(String[] args){ Date date=new Date();//创建Date对象 //通过format()方法对Date进行格式化 String year=String.format("%tY", date); String month=String.format("%tB", date); String day=String.format("%td", date); System.out.println("今年是:"+year+"年"+",现在是:"+month+",今天是:"+day+"号。"); } } |
运行结果:
今年是:2017年,现在是:十二月,今天是:21号。
2. 时间格式化
使用format()方法不但可以完成日期的格式化,也可以实现时间的格式化。
例 15.04 实现时间格式化
import java.util.Date; public class Demo{ public static void main(String[] args){ Date date=new Date();//创建Date对象 //通过format()方法对Date进行格式化 String hour=String.format("%tH", date); String minute=String.format("%tM", date); String second=String.format("%tS", date); System.out.println("现在是:"+hour+"时"+minute+"分"+second+"秒。"); } } |
运行结果:
现在是:17时18分53秒。
3. 格式化常见的日期时间组合
例 15.05 实现当前日期时间的全部格式化信息
import java.util.Date; public class Demo{ public static void main(String[] args){ Date date=new Date();//创建Date对象 //通过format()方法对Date进行格式化 String time=String.format("%tc", date); String form=String.format("%tF", date); System.out.println("全部的时间信息是:"+time); System.out.println("年-月-日格式:"+form); } } |
运行结果:
全部的时间信息是:星期四 十二月 21 17:23:16 CST 2017
年-月-日格式:2017-12-21
日期格式化操作类:simpleDateformat
1.构造方法
public SimpleDateFormat(String pattern)
传入日期时间标记实例化对象
2.将日期格式化为字符串数据
public final String format(Date date)
import java.text.SimpleDateFormat; import java.util.Date; public class Demo{ public static void main(String[] args) throws Exception{ SimpleDateFormat fmt=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); Date date=new Date(); System.out.println(fmt.format(date)); } } |
运行结果:
2017-12-22 09:19:25
3.将字符串格式化日期数据
public Date parse(String source) throws ParseException
import java.text.SimpleDateFormat; import java.util.Date; public class Demo{ public static void main(String[] args) throws Exception{ SimpleDateFormat fmt=new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); String str="2017-12-22 09:37:45"; Date date=fmt.parse(str); System.out.println(date); } } |
运行结果:
Fri Dec 22 09:37:45 CST 2017