index.jsp(登录界面)
<%@ page language="java" import="java.util.*" pageEncoding="UTF-8"%> <%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%> <% String path = request.getContextPath(); String basePath = request.getScheme()+"://"+request.getServerName()+":"+request.getServerPort()+path+"/"; %> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"> <html> <head> <base href="<%=basePath%>"> <title>用户登录</title> <meta http-equiv="pragma" content="no-cache"> <meta http-equiv="cache-control" content="no-cache"> <meta http-equiv="expires" content="0"> <meta http-equiv="keywords" content="keyword1,keyword2,keyword3"> <meta http-equiv="description" content="This is my page"> <!-- <link rel="stylesheet" type="text/css" href="styles.css"> --> <style type="text/css"> </style> </head> <body> <div> <form action="loginServlet" method="post"> <table align="center" > <tr> <td>用户名:</td> <td><input type="text" name="username"> <font color="red"> ${requestScope.namemsg}${requestScope.nameError}</font></td> </tr> <tr> <td>密码:</td> <td><input type="password" name="password"> <font color="red"> ${requestScope.pwdError}${requestScope.pwdmsg}</font></td> </tr> <tr> <td></td> <td><input type="submit" value="登录"> <input type="reset" value="取消"></td> </tr> </table> </form> </div> </body> </html>
loginServlet(登录验证)
package com.dwx.servlet; import java.io.IOException; import java.sql.ResultSet; import java.sql.SQLException; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import com.dwx.bean.user; import com.dwx.bean.userDao; /** * 用户登录验证 */ @WebServlet("/loginServlet") public class loginServlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ public loginServlet() { super(); // TODO Auto-generated constructor stub } /** * @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response) */ protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { doPost(request, response); } /** * @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response) */ protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String username=request.getParameter("username"); String password=request.getParameter("password"); //判断用户名、密码是否为空 if(username==""||username.length()==0){ request.setAttribute("namemsg","用户名为空!"); request.getRequestDispatcher("index.jsp").forward(request, response); } if(password==""||password.length()==0){ request.setAttribute("pwdmsg","密码为空!"); request.getRequestDispatcher("index.jsp").forward(request, response); } //判断用户名、密码是否正确 ResultSet rst=userDao.selectUser(username, password); try { if(rst.next()){ if(password.equals(rst.getString("password"))){ request.setAttribute("username",username); request.getRequestDispatcher("items.jsp").forward(request, response); }else{ request.setAttribute("pwdError","密码不正确!"); request.getRequestDispatcher("index.jsp").forward(request, response); } }else{ request.setAttribute("nameError","用户名不存在!"); request.getRequestDispatcher("index.jsp").forward(request, response); } } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
userDao.java(查询用户)
package com.dwx.bean; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.util.ArrayList; import java.util.List; import com.dwx.util.dao; public class userDao { public static ResultSet selectUser(String username,String password){ Connection conn=dao.getConnection(); String sql="select * from user where username=?"; ResultSet rst=null; try{ PreparedStatement prst=conn.prepareStatement(sql); prst.setString(1, username); rst=prst.executeQuery(); }catch(Exception ex){ ex.printStackTrace(); } return rst; } }
dao.java(建立数据库连接)
package com.dwx.util; import java.sql.Connection; import java.sql.DriverManager; public class dao { public static Connection getConnection(){ Connection conn=null; try{ Class.forName("com.mysql.jdbc.Driver"); //加载驱动 String url="jdbc:mysql://localhost:3306/db_item"; String username="root"; String password="root"; //创建数据库连接 conn=DriverManager.getConnection(url,username,password); }catch(Exception ex){ ex.printStackTrace(); } return conn; } }
效果演示:
1、用户名为空
2、密码为空
3、用户名不存在
扫描二维码关注公众号,回复:
905560 查看本文章
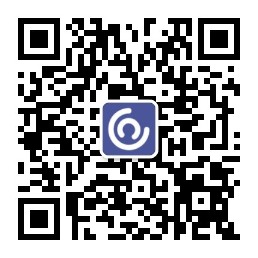
4、密码不正确
5、登录成功