学习网址:https://www.runoob.com/python/python-basic-syntax.html
运行代码后即可生成笔记
未完成部分:python函数未实践操作
#代码如下:
# chapter8:Python3 元组
print("\nchapter8:Python3 元组\n")
print("1.Python 的元组与列表类似,不同之处在于元组的元素不能修改。")
print("2.元组使用小括号,列表使用方括号。")
print("3.元组创建很简单,只需要在括号中添加元素,并使用逗号隔开即可。")
print("eg:有如下实例:")
print(">>>tup1 = ('Google', 'Runoob', 1997, 2000)\n"
">>> tup2 = (1, 2, 3, 4, 5 )\n"
">>> tup3 = \"a\", \"b\", \"c\", \"d\" # 不需要括号也可以\n"
">>> type(tup3)\n"
"<class 'tuple'>")
print("4.创建空元组:tup1 = ()")
print("5.元组中只包含一个元素时,需要在元素后面添加逗号,否则括号会被当作运算符使用:")
print("eg:")
print(">>>tup1 = (50)\n"
">>> type(tup1) # 不加逗号,类型为整型\n"
"<class 'int'>\n\n"
">>> tup1 = (50,)\n"
">>> type(tup1) # 加上逗号,类型为元组\n"
"<class 'tuple'>")
print("6.元组与字符串类似,下标索引从0开始,可以进行截取,组合等。")
print("7.元组可以使用下标索引来访问元组中的值")
print("eg:有如下实例:")
print("tup1 = ('Google', 'Runoob', 1997, 2000)\n"
"tup2 = (1, 2, 3, 4, 5, 6, 7 )\n"
"print (\"tup1[0]: \", tup1[0])\n"
"print (\"tup2[1:5]: \", tup2[1:5])")
print("\n上述实例输出结果为:")
tup1 = ('Google', 'Runoob', 1997, 2000)
tup2 = (1, 2, 3, 4, 5, 6, 7)
print("tup1[0]: ", tup1[0])
print("tup2[1:5]: ", tup2[1:5])
print("8.修改元组")
print("1)元组中的元素值是不允许修改的,但我们可以对元组进行连接组合")
print("eg:有如下实例:")
print("tup1 = (12, 34.56)\n"
"tup2 = ('abc', 'xyz')\n"
"# 以下修改元组元素操作是非法的。\n"
"# tup1[0] = 100\n"
"# 创建一个新的元组\n"
"tup3 = tup1 + tup2\n"
"print (tup3)")
print("\n上述实例输出结果为:")
tup1 = (12, 34.56)
tup2 = ('abc', 'xyz')
# 以下修改元组元素操作是非法的。
# tup1[0] = 100
# 创建一个新的元组
tup3 = tup1 + tup2
print(tup3)
print("9.删除元组")
print("1)元组中的元素值是不允许删除的,但我们可以使用del语句来删除整个元组")
print("eg:有如下实例:")
print("tup = ('Google', 'Runoob', 1997, 2000)\n"
"print (tup)\n"
"del tup\n"
"print (\"删除后的元组 tup : \")\n"
"print (tup)")
print("\n上述实例执行后的输出结果为:")
print("删除后的元组 tup : \n"
"Traceback (most recent call last):\n"
" File \"test.py\", line 8, in <module>\n"
" print (tup)\n"
"NameError: name 'tup' is not defined")
print("10.元组运算符")
print("1)与字符串一样,元组之间可以使用 + 号和 * 号进行运算。这就意味着他们可以组合和复制,运算后会生成一个新的元组")
print("2)使用规则:")
print("Python 表达式 结果 描述\n"
"len((1, 2, 3)) 3 计算元素个数\n"
"(1, 2, 3) + (4, 5, 6) (1, 2, 3, 4, 5, 6) 连接\n"
"('Hi!',) * 4 ('Hi!', 'Hi!', 'Hi!', 'Hi!') 复制\n"
"3 in (1, 2, 3) True 元素是否存在\n"
"for x in (1, 2, 3): print (x,) 1 2 3 迭代")
print("11.元组索引,截取")
print("1)因为元组也是一个序列,所以我们可以访问元组中的指定位置的元素,也可以截取索引中的一段元素")
print("eg:")
print("L = ('Google', 'Taobao', 'Runoob')\n"
"Python 表达式 结果 描述\n"
"L[2] 'Runoob' 读取第三个元素\n"
"L[-2] 'Taobao' 反向读取,读取倒数第二个元素\n"
"L[1:] ('Taobao', 'Runoob') 截取元素,从第二个开始后的所有元素。")
print("12.元组内置函数")
print("1)Python元组包含了以下内置函数")
print("序号 方法及描述 实例\n"
"1 len(tuple):计算元组元素个数。\n"
">>> tuple1 = ('Google', 'Runoob', 'Taobao')\n"
">>> len(tuple1)\n"
"3\n"
">>>\n"
"2 max(tuple):返回元组中元素最大值。\n"
">>> tuple2 = ('5', '4', '8')\n"
">>> max(tuple2)\n"
"'8'\n"
">>>\n"
"3 min(tuple):返回元组中元素最小值。\n"
">>> tuple2 = ('5', '4', '8')\n"
">>> min(tuple2)\n"
"'4'\n"
">>>\n"
"4 tuple(iterable):将可迭代系列转换为元组。\n"
">>> list1= ['Google', 'Taobao', 'Runoob', 'Baidu']\n"
">>> tuple1=tuple(list1)\n"
">>> tuple1\n"
"('Google', 'Taobao', 'Runoob', 'Baidu')")
print("13.所谓元组的不可变指的是元组所指向的内存中的内容不可变。")
#运行结果如下:
chapter8:Python3 元组
1.Python 的元组与列表类似,不同之处在于元组的元素不能修改。
2.元组使用小括号,列表使用方括号。
3.元组创建很简单,只需要在括号中添加元素,并使用逗号隔开即可。
eg:有如下实例:
tup1 = (‘Google’, ‘Runoob’, 1997, 2000)
tup2 = (1, 2, 3, 4, 5 )
tup3 = “a”, “b”, “c”, “d” # 不需要括号也可以
type(tup3)
<class ‘tuple’>
4.创建空元组:tup1 = ()
5.元组中只包含一个元素时,需要在元素后面添加逗号,否则括号会被当作运算符使用:
eg:tup1 = (50)
type(tup1) # 不加逗号,类型为整型
<class ‘int’>
tup1 = (50,)
type(tup1) # 加上逗号,类型为元组
<class ‘tuple’>
6.元组与字符串类似,下标索引从0开始,可以进行截取,组合等。
7.元组可以使用下标索引来访问元组中的值
eg:有如下实例:
tup1 = (‘Google’, ‘Runoob’, 1997, 2000)
tup2 = (1, 2, 3, 4, 5, 6, 7 )
print ("tup1[0]: ", tup1[0])
print ("tup2[1:5]: ", tup2[1:5])
上述实例输出结果为:
tup1[0]: Google
tup2[1:5]: (2, 3, 4, 5)
8.修改元组
1)元组中的元素值是不允许修改的,但我们可以对元组进行连接组合
eg:有如下实例:
tup1 = (12, 34.56)
tup2 = (‘abc’, ‘xyz’)
以下修改元组元素操作是非法的。
tup1[0] = 100
创建一个新的元组
tup3 = tup1 + tup2
print (tup3)
上述实例输出结果为:
(12, 34.56, ‘abc’, ‘xyz’)
9.删除元组
1)元组中的元素值是不允许删除的,但我们可以使用del语句来删除整个元组
eg:有如下实例:
tup = (‘Google’, ‘Runoob’, 1997, 2000)
print (tup)
del tup
print ("删除后的元组 tup : ")
print (tup)
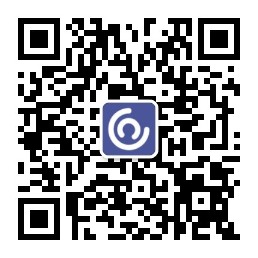
上述实例执行后的输出结果为:
删除后的元组 tup :
Traceback (most recent call last):
File “test.py”, line 8, in
print (tup)
NameError: name ‘tup’ is not defined
10.元组运算符
1)与字符串一样,元组之间可以使用 + 号和 * 号进行运算。这就意味着他们可以组合和复制,运算后会生成一个新的元组
2)使用规则:
Python 表达式 结果 描述
len((1, 2, 3)) 3 计算元素个数
(1, 2, 3) + (4, 5, 6) (1, 2, 3, 4, 5, 6) 连接
(‘Hi!’,) * 4 (‘Hi!’, ‘Hi!’, ‘Hi!’, ‘Hi!’) 复制
3 in (1, 2, 3) True 元素是否存在
for x in (1, 2, 3): print (x,) 1 2 3 迭代
11.元组索引,截取
1)因为元组也是一个序列,所以我们可以访问元组中的指定位置的元素,也可以截取索引中的一段元素
eg:
L = (‘Google’, ‘Taobao’, ‘Runoob’)
Python 表达式 结果 描述
L[2] ‘Runoob’ 读取第三个元素
L[-2] ‘Taobao’ 反向读取,读取倒数第二个元素
L[1:] (‘Taobao’, ‘Runoob’) 截取元素,从第二个开始后的所有元素。
12.元组内置函数
1)Python元组包含了以下内置函数
序号 方法及描述 实例
1 len(tuple):计算元组元素个数。
tuple1 = (‘Google’, ‘Runoob’, ‘Taobao’)
len(tuple1)
3
2 max(tuple):返回元组中元素最大值。
tuple2 = (‘5’, ‘4’, ‘8’)
max(tuple2)
‘8’
3 min(tuple):返回元组中元素最小值。
tuple2 = (‘5’, ‘4’, ‘8’)
min(tuple2)
‘4’
4 tuple(iterable):将可迭代系列转换为元组。
list1= [‘Google’, ‘Taobao’, ‘Runoob’, ‘Baidu’]
tuple1=tuple(list1)
tuple1
(‘Google’, ‘Taobao’, ‘Runoob’, ‘Baidu’)
13.所谓元组的不可变指的是元组所指向的内存中的内容不可变。
Process finished with exit code 0