文件结构如下
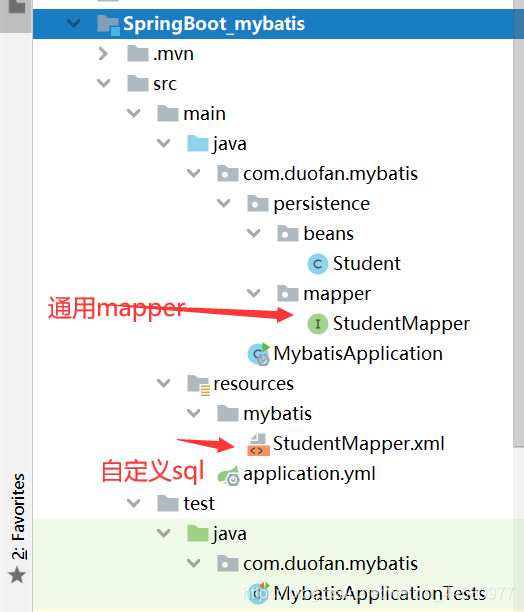
导入的依赖
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>tk.mybatis</groupId>
<artifactId>mapper-spring-boot-starter</artifactId>
<version>2.1.5</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
配置
spring:
datasource:
username: root
password: root
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf-8&useSSL=true&serverTimezone=UTC
driver-class-name: com.mysql.cj.jdbc.Driver
mybatis:
type-aliases-package: com.duofan.mybatis.persistence.beans
mapper-locations: classpath:/mybatis/*.xml
StudentMapper
package com.duofan.mybatis.persistence.mapper;
import com.duofan.mybatis.persistence.beans.Student;
import org.springframework.stereotype.Repository;
import tk.mybatis.mapper.common.Mapper;
import java.util.List;
@Repository
public interface StudentMapper extends Mapper<Student> {
List<Student> getAllStudent();
}
StudentMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.duofan.mybatis.persistence.mapper.StudentMapper">
<resultMap id="rm" type="com.duofan.mybatis.persistence.beans.Student">
<result property="id" jdbcType="BIGINT" column="id"/>
<result property="name" jdbcType="VARCHAR" column="name"/>
<result property="sex" jdbcType="VARCHAR" column="sex"/>
<result property="age" jdbcType="BIGINT" column="age"/>
</resultMap>
<select id="getAllStudent" resultType="com.duofan.mybatis.persistence.beans.Student">
SELECT * FROM STUDENT
</select>
</mapper>
测试代码
package com.duofan.mybatis;
import com.duofan.mybatis.persistence.beans.Student;
import com.duofan.mybatis.persistence.mapper.StudentMapper;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import tk.mybatis.spring.annotation.MapperScan;
import java.util.List;
@SpringBootTest
@MapperScan("com.duofan.mybatis.persistence.mapper")
class MybatisApplicationTests {
@Autowired
private StudentMapper studentMapper;
@Test
void contextLoads() {
System.out.println("Mapper 查询");
List<Student> students = studentMapper.selectAll();
students.forEach(student -> System.out.println(student));
System.out.println("mybatis xml查询");
List<Student> allStudent = studentMapper.getAllStudent();
allStudent.forEach(student -> System.out.println(student));
}
}
输出结果
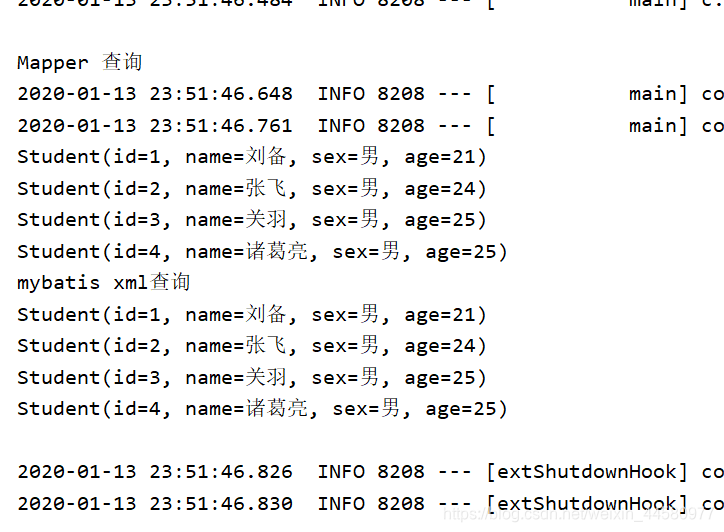