linux应用程序_1_文本浏览器_9_网络打印_3_debug_manager
前面一直使用串口输出调试信息,本篇开始添加网络打印调试信息功能。
仿照内核打印调试信息的方式,这里也设定0-7这8个打印等级,数字越小,等级越高,只有指定等级以下的调试信息能够被打印,打印等级、打印通道(从串口、网络两种方式)由客户端发送指令设置
为调试信息模块抽象一个结构体
typedef struct DebugOpr {
char *pcName;
int iCanUse;
int (*DebugInit)(void);
int (*DebugExit)(void);
int (*DebugPrint)(char *pcData);
struct DebugOpr *ptNext;
}T_DebugOpr, *PT_DebugOpr;
注册、入口出口、初始化等函数同前面类似
打印接口:
1、将调试信息存入缓存
2、比较打印等级,不满足打印等级则退出
3、选择可以使用的打印通道进行打印
int DebugPrint(const char *pcFormat, ...)
{
va_list tArg;
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
char pcBuf[1024];
int iLen;
char *pcBufTmp = pcBuf;
int iPrintLevel = DEFAULT_DBGLEVEL;
va_start (tArg, pcFormat);
iLen = vsprintf (pcBuf, pcFormat, tArg);
va_end (tArg);
if(pcBuf[0] == '<' && pcBuf[2] == '>')
{
iPrintLevel = pcBuf[1] - '0';
if(iPrintLevel < 8 && iPrintLevel >= 0)
pcBufTmp += 3;
else
iPrintLevel = DEFAULT_DBGLEVEL;
}
if(iPrintLevel > g_iPrintLevel)
return -1;
while(ptDebugOprTmp)
{
if(ptDebugOprTmp->iCanUse)
ptDebugOprTmp->DebugPrint(pcBuf);
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
return iLen;
}
扫描二维码关注公众号,回复:
8912175 查看本文章
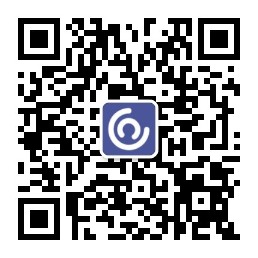
附代码:
debug_manager.h
#ifndef __DEBUG_MANAGER_H
#define __DEBUG_MANAGER_H
#define APP_EMERG "<0>" /* system is unusable */
#define APP_ALERT "<1>" /* action must be taken immediately */
#define APP_CRIT "<2>" /* critical conditions */
#define APP_ERR "<3>" /* error conditions */
#define APP_WARNING "<4>" /* warning conditions */
#define APP_NOTICE "<5>" /* normal but significant condition */
#define APP_INFO "<6>" /* informational */
#define APP_DEBUG "<7>" /* debug-level messages */
#define DEFAULT_DBGLEVEL 4
typedef struct DebugOpr {
char *pcName;
int iCanUse;
int (*DebugInit)(void);
int (*DebugExit)(void);
int (*DebugPrint)(char *pcData);
struct DebugOpr *ptNext;
}T_DebugOpr, *PT_DebugOpr;
int DebugPrint(const char *pcFormat, ...);
int SetDebugCanUse(char *pcBuf);
int SetDebugLevel(char *pcBuf);
int RegisterDebugOpr(PT_DebugOpr ptDebugOpr);
PT_DebugOpr GetDebugOpr(char *pcName);
void ShowDebugOpr(void);
int DebugInit(void);
int ReallyDebugInit(void);
void DebugExit(void);
int StdoutInit(void);
int NetInit(void);
#endif
debug_manager.c
#include <debug_manager.h>
#include <config.h>
#include <string.h>
#include <stdio.h>
#include <stdarg.h>
static PT_DebugOpr g_ptDebugOpr;
static int g_iPrintLevel = 8;
int RegisterDebugOpr(PT_DebugOpr ptDebugOpr)
{
PT_DebugOpr ptDebugOprTmp;
if(!g_ptDebugOpr)
{
g_ptDebugOpr = ptDebugOpr;
return 0;
}
ptDebugOprTmp = g_ptDebugOpr;
while(ptDebugOprTmp->ptNext)
{
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
ptDebugOprTmp->ptNext = ptDebugOpr;
return 0;
}
PT_DebugOpr GetDebugOpr(char *pcName)
{
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
while(ptDebugOprTmp)
{
if(strcmp(ptDebugOprTmp->pcName, pcName) == 0)
{
return ptDebugOprTmp;
}
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
return NULL;
}
void ShowDebugOpr(void)
{
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
int iCnt = 0;
printf("\r\n");
printf("supported debug:\r\n");
while(ptDebugOprTmp)
{
printf("%02d : %s\r\n",++iCnt, ptDebugOprTmp->pcName);
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
}
int SetDebugCanUse(char *pcBuf)
{
char *pcTmp;
char strOprName[20];
PT_DebugOpr ptTmp;
pcTmp = strchr(pcBuf, '=');
if(!pcTmp)
return -1;
strncpy(strOprName, pcBuf + sizeof("set") - 1, pcTmp - (pcBuf + sizeof("set") - 1));
strOprName[pcTmp - (pcBuf + sizeof("set") - 1)] = '\0';
ptTmp = GetDebugOpr(strOprName);
if(!ptTmp)
return -1;
if(pcTmp[1] == '0')
ptTmp->iCanUse = 0;
else if(pcTmp[1] == '1')
ptTmp->iCanUse = 1;
else
return -1;
return 0;
}
int SetDebugLevel(char *pcBuf)
{
if(strncmp(pcBuf, "setdebuglevel=", strlen("setdebuglevel=")))
return -1;
g_iPrintLevel = pcBuf[strlen("setdebuglevel=")] - '0';
return 0;
}
int DebugPrint(const char *pcFormat, ...)
{
va_list tArg;
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
char pcBuf[1024];
int iLen;
char *pcBufTmp = pcBuf;
int iPrintLevel = DEFAULT_DBGLEVEL;
va_start (tArg, pcFormat);
iLen = vsprintf (pcBuf, pcFormat, tArg);
va_end (tArg);
if(pcBuf[0] == '<' && pcBuf[2] == '>')
{
iPrintLevel = pcBuf[1] - '0';
if(iPrintLevel < 8 && iPrintLevel >= 0)
pcBufTmp += 3;
else
iPrintLevel = DEFAULT_DBGLEVEL;
}
if(iPrintLevel > g_iPrintLevel)
return -1;
while(ptDebugOprTmp)
{
if(ptDebugOprTmp->iCanUse)
ptDebugOprTmp->DebugPrint(pcBuf);
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
return iLen;
}
int DebugInit(void)
{
int iError = 1;
iError = StdoutInit();
iError &= NetInit();
return iError;
}
int ReallyDebugInit(void)
{
int iError = 1;
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
while(ptDebugOprTmp)
{
if(ptDebugOprTmp->DebugInit)
{
iError &= ptDebugOprTmp->DebugInit();
}
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
return iError;
}
void DebugExit(void)
{
PT_DebugOpr ptDebugOprTmp = g_ptDebugOpr;
while(ptDebugOprTmp)
{
if(ptDebugOprTmp->DebugExit)
{
ptDebugOprTmp->DebugExit();
}
ptDebugOprTmp = ptDebugOprTmp->ptNext;
}
}