Python语句、缩进、注释
在下面的文章中,将学习Python语句,为什么缩进很重要,如何在编程时使用注释。
Python Statement
Instructions that a Python interpreter can execute are called statements. For example, a = 1
is an assignment statement. if
statement, for
statement, while
statement etc. are other kinds of statements which will be discussed later.
Python解释器可以执行的命令,叫做语句。
比如 a = 1是一个赋值语句。if语句、for语句、while语句等等是其它类型的语句(后面会讲到)。
Multi-line statement
多行的语句
在Python中,用换行符标志一个语句的结束。但是我们可以使用行继续符(\)使语句扩展到多行。例如
a = 1+2+3+4+\
5+6+7
print(a)
#结果是28
上面这是显示的行继续符号的使用。
In Python, line continuation is implied inside parentheses ( ), brackets [ ] and braces { }.(在Python中,行的连续性隐藏在(),[],{}中)
For instance, we can implement the above multi-line statement as(例如,我们可以用这样的方法实现上面的代码)
a = (1+2+3+
4+5+6+7)
执行结果也是一样的。(但要注意,写代码的时候,最后一个括号")"要最后写。否则Python仍然认为换行符是语句结束的标志)
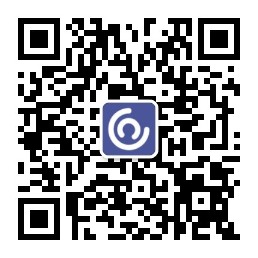
这个代码是用圆括号"()"做多行的命令。我们也可以用"[]"和"{}"来做,看例子:
colors = ['red','blue',
'yellow',
'green']
print(colors) #结果是['red','green',...]
#感觉不实用
colors = {'red','blue',
'yellow',
'green'}
print(colors) #结果是 set(['red','yellow',...])
我们也可以用分号 " ; "将多行命令放入一行,比如:
a=1; b=2; c=3;
#不建议这么搞,还是多行好
Python Indentation
Python缩进
大多数编程语言如C、C++、Java都使用括号{}来定义一个代码块。
Python使用缩进。
代码块(函数体、循环等)以缩进开始,以第一行未缩进结束。缩进量由您决定,但必须在整个块中保持一致。
通常四个空格用于缩进,并且优先于制表符。
for i in range(1,11):
print(i)
if(i==5):
break
在Python中强制缩进使代码看起来整洁干净。这个结果将使Python程序看起来相似且一致。
不正确的缩进将导致:IndentationError
Python Comments
Python注释
Comments are very important while writing a program. It describes what's going on
inside a program so that a person looking at the source code does not have a
hard time figuring it out. You might forget the key details of the program you just
wrote in a month's time. So taking time to explain these concepts in form of
comments is always fruitful.
In Python, we use the hash (#) symbol to start writing a comment.
It extends up to the newline character. Comments are for programmers for better
understanding of a program. Python Interpreter ignores comment.
在编程时注释非常重要。注释描述了程序的功能,所以当一个人读源码时不必花费很多精力来搞明白这段代码到底是干嘛的。
写的代码在一个月以后,可能就忘了关键性的细节了。花时间写注释是非常有帮助的。
在Python中,我们用 "#"开始写注释。"#"这个只能写一行注释。换行时需要另写"#"。
注释是为了让程序员更好地理解程序。Python解释器忽略注释(Python Interpreter ignores comment)。
#This is a comment
#print out hello
print('hello')
Multi-line comments
一次性写多行注释。(上面的都是单行注释。内容多时明显不方便)
我们可以用三重引号(triple quotes)写多行注释 (''') (""")
这些三重引号通常用于多行字符串。但它们也可以用作多行注释。除非它们不是docstring(是doc string?),否则不会生成任何额外的代码?(DocStrings见链接:《Python DocStrings》)
#三重引号拼写多行字符串
name = '''tom,jerry,
xiaoming'''
print(name) # 结果是这样: tom,jerry,\nxiaoming
#注意结果中有 \n
"""This is also a
perfect example of
multi-line comments"""