一、建数据库
MyDatabaseHelper.java
public class MyDatabaseHelper extends SQLiteOpenHelper { /*integer 整型,real 浮点型,primary key 主键,autoincrement 自增长,text 文本类型,blob 二进制数,*/ public static final String CREATE_BOOK="create table Book(" +"id integer primary key autoincrement," +"author text," +"price real," +"pages integer,"+ "name text)"; public static final String CREATE_CATEGORY="create table Category(" +"id integer primary key autoincrement," +"category_name text," +"category_code integer)"; private Context mContext; public MyDatabaseHelper(Context context, String name, SQLiteDatabase.CursorFactory factory, int version) { super(context, name, factory, version); mContext=context; } @Override public void onCreate(SQLiteDatabase sqLiteDatabase) { sqLiteDatabase.execSQL(CREATE_BOOK); sqLiteDatabase.execSQL(CREATE_CATEGORY); Toast.makeText(mContext,"create success!",Toast.LENGTH_SHORT).show(); } @Override public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) { sqLiteDatabase.execSQL("drop table if exists Book"); sqLiteDatabase.execSQL("drop table if exists Category"); onCreate(sqLiteDatabase); } }
main活动布局文件 activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <Button android:id="@+id/Create_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="创建数据库" /> <Button android:id="@+id/Add_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="添加数据" /> <Button android:id="@+id/Update_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="更新数据"/> <Button android:id="@+id/Delete_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="删除数据"/> <Button android:id="@+id/Query_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="查询数据"/> </LinearLayout>
在main活动中操作数据库(增删改查):
public class MainActivity extends AppCompatActivity { private Button bt1,bt2,bt3,bt4,bt5; private MyDatabaseHelper databaseHelper; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); databaseHelper=new MyDatabaseHelper(this,"BookStore.db",null,2); setContentView(R.layout.activity_main); bt1=(Button)findViewById(R.id.Create_button); bt1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { databaseHelper.getWritableDatabase();//先检测程序中有无这个数据库,如果没有,则创建 } }); bt2=(Button)findViewById(R.id.Add_button); bt2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { SQLiteDatabase db=databaseHelper.getWritableDatabase(); ContentValues values=new ContentValues();//获取ContentValues对象 values.put("name","The Da Code"); values.put("author","Dan"); values.put("pages",454); values.put("price",46.69); db.insert("Book",null,values);//插入第一条数据 values.clear(); values.put("name","The Lost Symbol"); values.put("author","Lost"); values.put("pages",996); values.put("price",82.65); db.insert("Book",null,values);//插入第二条数据 } }); bt3=(Button)findViewById(R.id.Update_button); bt3.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { SQLiteDatabase db=databaseHelper.getWritableDatabase(); ContentValues values=new ContentValues(); values.put("price",10.99); db.update("Book",values,"name=?",new String[]{"The Da Code"}); } }); bt4=(Button)findViewById(R.id.Delete_button); bt4.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { SQLiteDatabase db=databaseHelper.getWritableDatabase(); db.delete("Book","pages > ?",new String[]{"500"});//删除页数超过500的书 } }); bt5=(Button)findViewById(R.id.Query_button); bt5.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { // SQLiteDatabase db=databaseHelper.getWritableDatabase();//获取数据库对象 // Cursor cursor=db.query("Book",null,null,null,null,null,null);//查询Book表中的所有数据 // if(cursor.moveToFirst()){ // do{ // String name=cursor.getString(cursor.getColumnIndex("name")); // String author=cursor.getString(cursor.getColumnIndex("author")); // int pages=cursor.getInt(cursor.getColumnIndex("pages")); // double price=cursor.getDouble(cursor.getColumnIndex("price")); // Log.d("MainActivity","Book name is:"+name); // Log.d("MainActivity","Book author is:"+author); // Log.d("MainActivity","Book page is:"+pages); // Log.d("MainActivity","Book price is:"+price); // }while(cursor.moveToNext()); // } // cursor.close(); Intent intent=new Intent(MainActivity.this,ShowDataActivity.class); startActivity(intent); } }); } }
BookStore.java
public class BookStore { private int id; private String name; private String author; private int pages; private double price; public BookStore(int id,String name,String author,int pages,double price){ this.id=id; this.name=name; this.author=author; this.pages=pages; this.price=price; } public int getId() { return id; } public String getName() { return name; } public String getAuthor() { return author; } public int getPages() { return pages; } public double getPrice() { return price; } }
建适配布局文件 book_item.xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content"> <TextView android:id="@+id/book_id" android:gravity="center" android:layout_weight="1" android:text="编号" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_name" android:gravity="center" android:layout_weight="1" android:text="书名" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_author" android:gravity="center" android:layout_weight="1" android:text="作者" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_pages" android:gravity="center" android:layout_weight="1" android:text="页数" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_price" android:gravity="center" android:layout_weight="1" android:text="价格" android:layout_width="0dp" android:layout_height="50dp" /> </LinearLayout>
ListView显示界面 datalayout.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content"> <TextView android:id="@+id/book_id" android:gravity="center" android:layout_weight="1" android:text="编号" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_name" android:gravity="center" android:layout_weight="1" android:text="书名" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_author" android:gravity="center" android:layout_weight="1" android:text="作者" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_pages" android:gravity="center" android:layout_weight="1" android:text="页数" android:layout_width="0dp" android:layout_height="50dp" /> <TextView android:id="@+id/book_price" android:gravity="center" android:layout_weight="1" android:text="价格" android:layout_width="0dp" android:layout_height="50dp" /> </LinearLayout> <TextView android:layout_width="match_parent" android:layout_height="1dp" android:background="@android:color/background_dark"/> <ListView android:id="@+id/book_lv" android:layout_width="match_parent" android:layout_height="match_parent"> </ListView> </LinearLayout>
ListView适配器:BookAdapter.java
public class BookAdapter extends ArrayAdapter<BookStore> { private int resourceId; public BookAdapter(Context context, int resource,List<BookStore>objects) { super(context, resource,objects); resourceId=resource; } @NonNull public View getView(int position, View convertView,ViewGroup parent) { BookStore bs=getItem(position);//获取当前项的book实例 View view= LayoutInflater.from(getContext()).inflate(resourceId,parent,false); TextView book_id=(TextView)view.findViewById(R.id.book_id); TextView book_name=(TextView)view.findViewById(R.id.book_name); TextView book_author=(TextView)view.findViewById(R.id.book_author); TextView book_pages=(TextView)view.findViewById(R.id.book_pages); TextView book_price=(TextView)view.findViewById(R.id.book_price); book_id.setText(String.valueOf(bs.getId())); book_name.setText(bs.getName()); book_author.setText(bs.getAuthor()); book_pages.setText(String.valueOf(bs.getPages())); book_price.setText(String.valueOf(bs.getPrice())); return view; } }
ListView显示页面:ShowDataActivity.java
public class ShowDataActivity extends AppCompatActivity { private List<BookStore> bookList=new ArrayList<>(); private ListView lv; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.datalayout); initBooks(); BookAdapter adapter = new BookAdapter(ShowDataActivity.this, R.layout.book_item, bookList); ListView listView = (ListView) findViewById(R.id.book_lv); listView.setAdapter(adapter); } private void initBooks() { //扫描数据库,将信息放入booklist MyDatabaseHelper mdb = new MyDatabaseHelper(this, "BookStore.db", null, 2);//打开数据库 SQLiteDatabase sd = mdb.getReadableDatabase();//获取数据库 Cursor cursor=sd.query("Book",null,null,null,null,null,null);//查询Book表中的所有数据 while(cursor.moveToNext()){ int id=cursor.getInt(cursor.getColumnIndex("id")); String name=cursor.getString(cursor.getColumnIndex("name")); String author=cursor.getString(cursor.getColumnIndex("author")); int pages=cursor.getInt(cursor.getColumnIndex("pages")); double price=cursor.getDouble(cursor.getColumnIndex("price")); BookStore bookStore=new BookStore(id,name,author,pages,price); bookList.add(bookStore); } cursor.close(); } }
运行截图:
扫描二维码关注公众号,回复:
881252 查看本文章
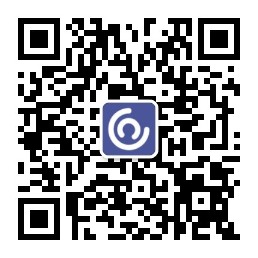