1.原题:
describe
A sequence of integer numbers a1 , a2 , ..., an is called a Fibonacci sequence if ai = ai-2+ai-1 for all i=3,4,...,n.
Given a sequence of integer numbers c1 , c2 , ..., cm you have to find its longest Fibonacci subsequence.
Input
There are several test cases in the input. The first line of each case contains m (1 <= m <= 3,000). Next line contains m integer numbers not exceeding 109 by their absolute value.
There is a new line between each case.
Output
On the first line of each case print the maximal length of the Fibonacci subsequence of the given sequence. On the second line print the subsequence itself.
There is a new line between each case.
Sample Input
10
1 1 3 -1 2 0 5 -1 -1 8
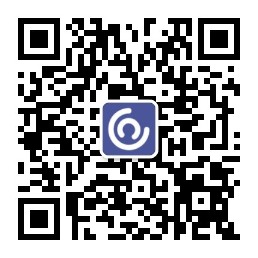
Sample Output
5
1 -1 0 -1 -1
中文解释:就是讲有一堆数据比如这里的样例输入的1 1 3 -1 2 0 5 -1 -1 8,在这里呢,我们需要找到一组斐波那契数列,并且这组斐波那契数列能够最长。
斐波那契数列...基本上学过c语言的开头解的几个程序,就是它了,使用递归思想是吧。
斐波那契额数列的表达式为
an = an-1+an-2
所以你只需要确定an-1和an-2,那你就能疯狂的增长下去。
按照题目的意思,你的an,an-1,an-2都是给定的序列。
所以要怎么找?
2 解法
其实这个问题十分简单,就是一个搜索问题,使用深度搜索和广度搜索都可以。
想要了解深度搜索和广度搜索的同学,运气好的可以在这个系列中找到,运气不好的...去其他小哥哥的小姐姐的博客去找一下哟~
简单的算法思想:
我拥有一个搜索栈,第一次,我将所有数列入栈。
然后开始循环搜索。
首先弹出栈顶。
然后根据栈顶值生成下一层搜索的节点,栈顶值为父节点。
下一层节点的剪枝条件就是本层节点值(an-1)+父节(an-2)点值产生的an值
是否存在于数列当中。如果不存在,则剪枝,存在,一次入栈,向下搜索。
我们可以使用代码来进行表达
图:暂略
深度搜索过程
3 代码
节点组成结构
class CPoint{
public int value = 0;
public CPoint parent;
public int index =0;
public int steps = 0;
}
深度优先搜索里面的几个重要条件
1.子节点的index>父节点的index,这是搜索组合的基本操作
2.字节点入栈的合法条件
子节点+子节点的父节点的值=当前候选入栈节点的值
java程序
package com.company;
import javafx.scene.Parent;
import java.util.Scanner;
import java.util.Stack;
public class FBNQArray {
/**
* 原始数据
*/
public CPoint[] originData;
public CPoint maxPoint;
public int size;
public boolean isLegal(CPoint cPoint,CPoint compare){
if (cPoint.value+ cPoint.parent.value == compare.value ){
return true;
}
return false;
}
public CPoint[] insNextPoint(CPoint parent){
Stack stack = new Stack();
for (int i =0;i<originData.length;i++){
if (originData[i].index>parent.index){
stack.push( new CPoint(originData[i].value,parent,originData[i].index).setSteps(parent.steps+1)) ;
}
}
CPoint[] cahceList = new CPoint[stack.size()];
while (!stack.isEmpty()){
cahceList[stack.size() - 1] = (CPoint) stack.pop();
}
return cahceList;
}
public static void main(String[] args) {
FBNQArray array = new FBNQArray();
Stack mainStack = new Stack();
Scanner scanner = new Scanner(System.in);
array.size = scanner.nextInt();
array.originData = new CPoint[array.size];
for (int i =0;i<array.size;i++){
array.originData[i] = new CPoint(scanner.nextInt(),null,i).setSteps(0);
}
for (int i =0;i<array.originData.length;i++){
mainStack.push(array.originData[i]);
}
array.maxPoint = array.originData[0];
while (!mainStack.isEmpty()){
CPoint tempValue = (CPoint) mainStack.pop();
if (array.maxPoint.steps<tempValue.steps){
array.maxPoint = tempValue;
}
if (tempValue.parent!= null) {
CPoint[] nextPointList = array.insNextPoint(tempValue);
for (int i = 0; i < nextPointList.length; i++) {
if (array.isLegal(tempValue,nextPointList[i]) == true) {
mainStack.push(nextPointList[i]);
}
}
}else {
CPoint[] nextPointList = array.insNextPoint(tempValue);
for (int i =0;i<nextPointList.length;i++){
mainStack.push(nextPointList[i]);
}
}
}
System.out.println(array.maxPoint.reValue());
}
}
class CPoint{
public int value = 0;
public CPoint parent;
public int index =0;
public int steps = 0;
public CPoint(int ci,CPoint cp,int index){
this.value = ci;
this.parent = cp;
this.index = index;
}
public CPoint setSteps(int sp){
this.steps = sp;
return this;
}
public String reValue(){
CPoint cache = this;
String cacheStr ="";
Stack emptyStack = new Stack();
while (cache!= null){
emptyStack.push(cache);
cache = cache.parent;
}
while (!emptyStack.isEmpty()){
CPoint cache2 = (CPoint)emptyStack.pop();
cacheStr += cache2.value+" ";
}
return cacheStr;
}
}
结果: