双端队列是由一些表组成的数据结构,具体简单实现代码如下(不是c++STL里面的哦~)
1.Push(X):将项X插入双端队列的前端。
2.Pop ( ): 从双端队列中删除前端项并将其返回。
3.Inject (X) :将项X插入到双端队列的尾端。
4.Eject():从双端队列中删除尾端项并将其返回。
5.Empty () :双端队列是否为空。
6.IsFull () :双端队列是否满。
7.Show():打印双端队列中的元素。
扫描二维码关注公众号,回复:
8775529 查看本文章
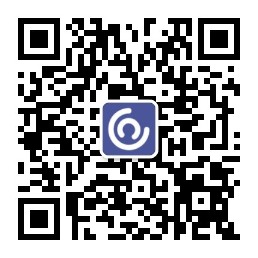
typedef class Double_ended_queue Queue;
typedef int ElemType;
typedef class Double_ended_queue Queue_Node;
const ElemType MaxSize = 100;
class Double_ended_queue {
public:
Double_ended_queue(int _f, int _l) :first(_f), last(_l), cur_f(_f), cur_l(_l) {};
~Double_ended_queue() {};
public:
void Push(ElemType X);
ElemType& Pop();
void Inject(ElemType X);
ElemType& Eject();
std::size_t Size()const;
bool Empty()const;
bool IsFull()const;
void Show()const;
private:
int first;
int last;
int cur_f;
int cur_l;
ElemType array[MaxSize];
};
void Double_ended_queue::Push(ElemType X) {
if (cur_f == cur_l)
throw std::out_of_range("Queue is Full!!");
else {
++cur_f;
array[cur_f] = X;
}
}
ElemType& Double_ended_queue::Pop() {
if (cur_f == first)
throw std::out_of_range("Queue is Empty!!");
else {
--cur_f;
return array[cur_f];
}
}
void Double_ended_queue::Inject(ElemType X) {
if (cur_l == cur_f)
throw std::out_of_range("Queue is Full");
else {
--cur_l;
array[cur_l] = X;
}
}
ElemType& Double_ended_queue::Eject() {
if (cur_l == last)
throw std::out_of_range("Queue is Empty!!");
else {
++cur_l;
return array[cur_l - 1];
}
}
bool Double_ended_queue::Empty()const {
if (cur_f == first && cur_l == last)
return true;
else
return false;
}
bool Double_ended_queue::IsFull()const {
if (cur_f == last - 1 || cur_l == first + 1 || cur_f + cur_l == MaxSize - 1)
return true;
else
return false;
}
void Double_ended_queue::Show()const {
if (!Empty()) {
for (int i = first + 1; i <= cur_f; ++i) {
std::cout << array[i] << "\n";
}
for (int i = cur_l; i < last; ++i) {
std::cout << array[i] << "\n";
}
}
else
throw std::out_of_range("Queue is Empty!!");
}
std::size_t Double_ended_queue::Size()const {
return (cur_f - first) + (last - cur_l);
}
int main(void)
{
int _first = -1;
int _last = MaxSize;
Queue queue(_first, _last);
for (int i = 1; i < 3; ++i) {
queue.Push(i);
}
for (int i = 4; i < 7; ++i) {
queue.Inject(i);
}
queue.Show();
std::cout <<"size:"<< queue.Size() << "\n";
std::cout <<"Delete the elements of the front end is:"<<queue.Pop() << "\n";
std::cout<<"The element at the end of the deletion is: "<<queue.Eject() << "\n";
return 0;
}