一、实验要求
- 顺序栈结构和运算定义,算法的实现以库文件方式实现,不得在测试主程序中直接实现;
- 程序有适当的注释。
- 实验程序有较好可读性,各运算和变量的命名直观易懂,符合软件工程要求;
二、数据结构设计
template<class ElementType>
class Stack{
public :
Stack(); //初始化栈
~Stack(); //销毁栈
int empty(); //判断栈是否为空
int pop(ElementType & x); //出栈
int push(ElementType x); //压栈
ElementType top(); //取栈顶元素
void print(); //遍历栈
private :
ElementType * data; //栈数据储存
int len; //栈当前元素个数
};
三、代码实现
#ifndef _QUEUE_H_
#define _QUEUE_H_
#include <iostream>
#define MAXLEN 100
using namespace std;
template<class ElementType>
class Queue{
public:
Queue(); //初始化队列
~Queue(); //销毁队列
bool empty(); //判断是否为空
bool full(); //判断栈是否为满
void enter(ElementType x); //入队
void out(); //出队
void getFront(ElementType &x); //取队头元素
int getLength(); //求当前队列中元素个数
void print(); //遍历队列
private:
ElementType * data;
int front;
int rear;
};
//初始化队列
template<class ElementType>
Queue<ElementType>::Queue()
{
data=new ElementType[MAXLEN];
front=rear=0;
}
//销毁队列
template<class ElementType>
Queue<ElementType>::~Queue()
{
delete [] data;
}
//判断是否为空
template<class ElementType>
bool Queue<ElementType>::empty()
{
if( front==rear)
{
return true;
}
else
{
return false;
}
}
//判断栈是否为满
template<class ElementType>
bool Queue<ElementType>::full()
{
if((rear+1)%MAXLEN==front)
{
return true; //队满
}
else{
return false;
}
}
//入队
template<class ElementType>
void Queue<ElementType>::enter(ElementType x)
{
if(full())
{
cout<<"队满,不能入队!"<<endl;
}
else{
rear=(rear+1)%MAXLEN;
data[rear]=x;
}
}
//出队
template<class ElementType>
void Queue<ElementType>::out()
{
if(front==rear)
{
cout<<"队空,不能出队!"<<endl;
}
else{
front=(front+1)%MAXLEN; //指针后移
}
}
//取队头元素
template<class ElementType>
void Queue<ElementType>::getFront(ElementType &x)
{
if(empty())
{
cout<<"队空,不能取元素!"<<endl;
}
else{
x=data[(front+1)%MAXLEN];
}
}
//求当前队列中元素个数
template<class ElementType>
int Queue<ElementType>::getLength()
{
return (rear-front+MAXLEN)%MAXLEN;
}
//遍历队列
template<class ElementType>
void Queue<ElementType>::print()
{
int p=front;
while(rear!=p)
{
p=(p+1)%MAXLEN;
cout<<data[p]<<" ";
}
}
#endif // _QUEUE_H_
#ifndef _SEQSTACK_H_
#define _SEQSTACK_H_
#include <iostream>
#define MAXLEN 100
using namespace std;
template<class ElementType>
class Stack{
public :
Stack(); //初始化栈
~Stack(); //销毁栈
int empty(); //判断栈是否为空
int pop(ElementType & x); //出栈
int push(ElementType x); //压栈
ElementType top(); //取栈顶元素
void print(); //遍历栈
private :
ElementType * data; //栈数据储存
int len; //栈当前元素个数
// int size; //栈的大小
};
//构造函数,初始化栈
template<class ElementType>
Stack<ElementType>::Stack()
{
data=new ElementType[MAXLEN];
len=0;
}
//析构函数,销毁栈
template<class ElementType>
Stack<ElementType>::~Stack()
{
delete [] data;
}
//判断栈是否为空
template<class ElementType>
int Stack<ElementType>::empty()
{
if(len==0)
{
return 1;
}
else{
return 0;
}
}
//出栈,成功返回1,否则返回0
template<class ElementType>
int Stack<ElementType>::pop(ElementType & x)
{
if(len==0)
{
return 0;
}
else{
x=data[len-1];
len--;
return 1;
}
}
//压栈
template<class ElementType>
int Stack<ElementType>::push(ElementType x)
{
if(len==MAXLEN)
{
return 0;
}
else{
len++;
data[len-1]=x;
return 1;
}
}
//取栈顶元素
template<class ElementType>
ElementType Stack<ElementType>::top()
{
if(empty())
{
return NULL;
}
else{
return data[len-1];
}
}
//遍历栈
template<class ElementType>
void Stack<ElementType>::print()
{
int i=0;
for(i;i<len;i++)
{
cout<<data[i]<<" ";
}
}
#endif // _SEQSTACK_H_
#include <iostream>
#include <string>
#include <Cstdlib>
#include "seqStack.h"
#include "queue.h"
using namespace std;
//1.输出函数
void print(int x)
{
char a[17]={'0','1','2','3','4','5','6','7','8','9','A','B','C','D','E','F'};
switch( x)
{
case 0 :
cout<<a[x];
break;
case 1 :
cout<<a[x];
break;
case 2 :
cout<<a[x];
break;
case 3 :
cout<<a[x];
break;
case 4 :
cout<<a[x];
break;
case 5 :
cout<<a[x];
break;
case 6 :
cout<<a[x];
break;
case 7 :
cout<<a[x];
break;
case 8 :
cout<<a[x];
break;
case 9 :
cout<<a[x];
break;
case 10 :
cout<<a[x];
break;
case 11 :
cout<<a[x];
break;
case 12 :
cout<<a[x];
break;
case 13 :
cout<<a[x];
break;
case 14 :
cout<<a[x];
break;
case 15 :
cout<<a[x];
break;
case 16 :
cout<<a[x];
break;
}
}
//2.十进制转化为16进制(顺序栈实验),其中i为十进制数,k为16
void convert(int i,int k)
{
Stack<int> S;
int mod,x; //mod为余数,x保存出栈元素
while(i!=0)
{
mod=i%k; //mod保存余数
S.push(mod); //将mod入栈
i=i/k; //商保存在i中
}
cout<<k<<"进制为:";
while(!S.empty())
{
S.pop(x); //取栈顶元素,并出栈
print(x); //输出x
}
}
//3.括号匹配
bool is_match(const string &str)
{
size_t len = str.length(); //获取字符串长度
Stack<char> s; //定义栈s
Queue<char> t; //定义队列t
int i;
char x,y;
int p=0,q=0; //p,q分别记录左括号个数和右括号个数
for (i = 0; i < len; ++i)
{
if (str[i] =='('||str[i] =='{' ||str[i] =='[')
{
s.push(str[i]); //栈s保存左括号
p++;
continue;
}
if (str[i] ==')'||str[i] =='}' ||str[i] ==']')
{
t.enter(str[i]); //队t保存右括号
q++;
continue;
}
}
if(p==q)
{
for(i=0;i<p;i++)
{
t.getFront(x);
if((s.top()=='('&& x==')')||(s.top()=='{'&& x=='}')||(s.top()=='['&& x==']'))
{
s.pop(y);
t.out();
}
}
}
else
{
return false;
}
if (s.empty()&&t.empty())
{
return true;
}
else{
return false;
}
s.~Stack();
t.~Queue();
}
int main()
{
int a;
cout<<"******************************************"<<endl;
cout<<"按1十进制转十六进制"<<endl;
cout<<"按2括号匹配"<<endl;
cout<<"按3退出"<<endl;
cout<<"******************************************"<<endl;
cout<<"请输入执行算法的序号:";
cin>>a;
while(a!=3)
{
switch(a)
{
case 1 :
int i;
cout<<"请输入要转换的数字:";
cin>>i;
convert(i,16);
cout<<endl;
break;
case 2:
string str="(1+2)";
cout<<"请输入字符串:";
cin>>str;
if(is_match(str))
{
cout<<"匹配"<<endl;
}
else{
cout<<"不匹配"<<endl;
}
break;
}
system("PAUSE");
system("CLS");
cout<<"******************************************"<<endl;
cout<<"按1十进制转十六进制"<<endl;
cout<<"按2括号匹配"<<endl;
cout<<"按3退出"<<endl;
cout<<"******************************************"<<endl;
cout<<"请输入执行算法的序号:";
cin>>a;
}
return 0;
}
四、实验截图
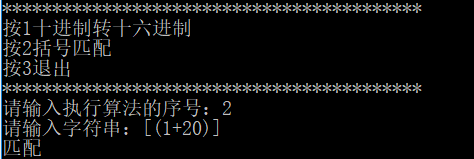
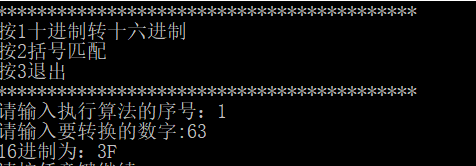