订单商品模型分析
对于数据模型分析思路
1)搞清楚每张表的数据内容
分模块对每张表的内容进行熟悉, 相当于你学习系统,需求功能的过程
2)每张表重要的字段设置
非空、外键字段
3)数据库级别,表与表之间的关系 外键关系
4)表与表之间的业务关系
在分析表与表之间的业务关系时,一定要建立在某个业务意义的基础之上
数据模型分析
用户表user 记录了购买商品的用户信息
id:自增主键
订单表order 记录了用户所创建的订单
number:订单号
user_id : 外键 用户id
订单明细表orderall 记录了订单的详细信息
order_id 外键 订单id
items_id 商品id
商品表items 记录了商品信息
表与表之间的业务关系时需要建立,在某个业务意义基础上去分析
先分析数据级别之间有关系的表之间的业务关系
user和order
user ->orders 一个用户可以创建多个订单
orders ->user 一个订单只能由一个用户创建
orders与orderall
orders ->orderall 一个订单可以包括多个订单明细 因为一个订单可以购买多个商品,每个商品的购买信息在orderall记录,一对多
返回过来,一个订单明细 只能包括在一个订单中 一对一
orderall ->item 一个订单明细,只对应一个商品信息
item -> 一个商品可以包括在多个订单中
在分析数据库级别没有关系的表之间是否有业务关系
order与item 可以通过orderall建议关系
一对一查询
需求:查询订单信息,关联查询创建订单的信息
resultType实现与resultMap分别实现
sql语句
确定查询的主表: 订单表
确定查询的关联表: 用户表
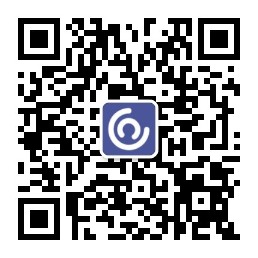
关联查询:使用内连接还是外连接
由于orders表中有一个外键(user_id)
通过外键关联查询用户表只能查询出用户一条记录,可以使用内连接
SELECT
orders.*,
user.username,
userss.address
FROM
orders,
user
WHERE orders.user_id = user.id
创建pojo
将上边的sql结果集映射到pojo,pojo中必须包括所有的查询列名
原始的order.java不能映射全部字段,需要创建新的pojo
创建一个pojo,继承包括查询字段较多的类
package cn.mmz.mybatis.pojo;
/**
* @Classname 订单的拓展类
* @Description TODO
* @Date 2020/1/5 12:13
* @Created by mmz
*/
//通过此类映射订单和用户查询的结果,让此类继承包括字段较多的pojo类
public class OrderCustom extends Orders{
//添加用户属性
}
package cn.mmz.mybatis.pojo;
/**
* @Classname 订单的拓展类
* @Description TODO
* @Date 2020/1/5 12:13
* @Created by mmz
*/
//通过此类映射订单和用户查询的结果,让此类继承包括字段较多的pojo类
public class OrderCustom extends Orders{
//添加用户属性
private String username;
private String sex;
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
private String address;
}
映射的结果就创建好了
mapper.xml的编写
<select id="findOrderUser" resultType="cn.mmz.mybatis.pojo.OrderCustom">
SELECT
orders.*,
user.username,
userss.address
FROM
orders,
user
WHERE orders.user_id = user.id
</select>
mapper.java
接口也编写完成了
public interface OrdersMapperCustom {
public List<OrderCustom> findOrdersUser() throws Exception;
}
最终的测试类
public void testFindOrdersUser() throws Exception{
SqlSession sqlSession = sqlSessionFactory.openSession();
//创建代理对象
OrdersMapperCustom ordersMapperCustom = sqlSession.getMapper(OrdersMapperCustom.class);
//调用Mapper方法
List<OrderCustom> list = ordersMapperCustom.findOrdersUser();
System.out.println(list);
sqlSession.close();
}
resultMap实现
同resultType的sql语句
使用resultMap映射的思路
使用resultMap将查询结果映射到Orders这个对象中
在order这个类中添加User属性
将关联查询出来的用户信息映射到order对象中的user属性中
需要Orders类中添加user属性
需要定义mapper.xml与.java
resultMap要配置订单信息和用户信息
如果有个多个列组成唯一标识,需要配置多个id
column:订单信息唯一标识的列
property:订单信息唯一标识的列 所映射到的哪个属性中
<id column="id" property="id"></id>
<result column="user_id" property="userId"></result>
<result column="number" property="number"></result>
<result column="createtime" property="createtime"></result>
<result column="note" property="note"></result>
association这个关键字用来映射关联查询单个对象的信息
property:要将关联查询的用户信息映射到Orders中的哪个属性
javaType 映射成为的java类型
column:指定唯一标识用户信息的列
<association property="user" javaType="cn.mmz.mybatis.pojo.User">
<id column="user_id" javaType="id"></id>
<result column="username" property="username"></result>
<result column="sex" property="sex"></result>
<result column="address" property="address"></result>
</association>
resultMap与resultType
实现一对一查询: resultType实现较为简单,如果pojo没有包括没有查询出来的列名,需要增加列名对应的属性,即可完成映射
如果没有查询结果的特殊要求建议使用resultType
resultMap需要单独的定义,实现起来比较麻烦,如果有特殊的对查询结果要求。使用resultMap可以完成对关联的信息,映射到pojo的属性中
resultMap可以实现延迟加载,而Type不可以
一对多查询
查询订单以及订单明细
sql语句
确定主查询表 订单表
确定关联查询表 订单明细表
在一对一查询基础上添加订单明细表关联即可
使用resultType将上边的查询结果集映射到pojo中,最终的list的订单信息的pojo就会重复
要求:对主查询orders映射不能出现重复记录
在orders.java添加一个list List<orderDetails> orderDetails
属性
最终会将订单信息映射到orders中,订单所对应的订单的明细映射到orders中的orderDetails属性中。
每个orders中的属性List<orderDetails> orderDetails
存储了该订单的所对应的订单明细
mapper.xml
association映射到单个记录中
collection映射到集合中
ofType 指定映射到集合中pojo的类型
还可以使用继承,不用在此中配置订单信息和用户信息的映射
<resultMap id="OrdersAndOrderDeResultMap" type="cn.mmz.mybatis.pojo.Orders">
<id column="id" property="id"></id>
<result column="user_id" property="userId"></result>
<result column="number" property="number"></result>
<result column="createtime" property="createtime"></result>
<result column="note" property="note"></result>
<association property="user" javaType="cn.mmz.mybatis.pojo.User">
<id column="user_id" property="id"></id>
<result column="username" property="username"></result>
<result column="sex" property="sex"></result>
<result column="address" property="address"></result>
</association>
<collection property="orderdetails" ofType="cn.mmz.mybatis.pojo.Orderdetail">
<id column="orderdetail_id" property="id"></id>
<result column="items_id" property="itemsId"></result>
<result column="items_num" property="itemsNum"></result>
<result column="orders_id" property="ordersId"></result>
</collection>
</resultMap>
mapper.java
public List<Orders> findOrdersAndOrderDeResultMap() throws Exception;
@Test
public void testfindOrdersAndOrderDeResultMap() throws Exception{
SqlSession sqlSession = sqlSessionFactory.openSession();
//创建代理对象
OrdersMapperCustom ordersMapperCustom = sqlSession.getMapper(OrdersMapperCustom.class);
//调用Mapper方法
List<Orders> list = ordersMapperCustom.findOrdersAndOrderDeResultMap();
System.out.println(list);
sqlSession.close();
}
小结
mybatis使用resultMap的collection对关联查询的多条记录映射到一个list集合属性中
使用resultType:将订单明细 映射到orders映射到orderdetails,需要自己处理,双重循环遍历,去掉重复记录,存储在list中