div 实现input 的placeholder效果
contenteditable="true"属性
1 设置div为可编辑状态,则可点击获取焦点,同时div的内容也是可以编辑的;如:
<div contenteditable="true"></div>
.dom1, .dom2, .dom3 {
color: black;
width: 400px;
height: 30px;
border: 1px solid red;
margin: 20px 50%;
}
.dom1:empty:after,
.dom2:empty:after,
.dom3:empty:after {
content: attr(placeholder);
color: red;
}
.dom1:focus:after {
content: none;
}
html部分
<div class="dom1 dom" contenteditable="true" placeholder="请输入"></div>
<div class="dom2 dom" contenteditable="true" placeholder="请输入"></div>
<div class="dom3 dom" contenteditable="true" placeholder="请输入"></div>
效果
@supports属性
@supports语法规则
@supports <条件规则> {
/* 特殊样式规则 */
}
@supports中的“条件规则”可以声明一条或者几个由不同的逻辑运算符相结合的声明(比如说,非(not),或(or),与(and)等)。而且还可以使用括号来确定其操作的优先级关系。
最简单的条件表达式是一个CSS声明,一个CSS属性名,后面加上一个属性值,属性名与属性值之前用分号隔开,比如:
(display:flex)
我们来看一个简单的例子:
@supports (display:flex) {
section { display: flex } ... }
上面这段代码的意思是:如果浏览器支持“display:flex”属性,那么在“section”元素上就运用“display:flex”样式。
@supports还可以根据不同的逻辑运算符相结合,具有不同的语法规则,接下来我们一起来细化一下@supports的语法规则,以及使用细节。
基本属性的检查
正如前面的示例一样,你可以使用CSS的基本属性来进行检查:
@supports (display: flex) {
div { display: flex; } }
这种是@supports最基本的使用规则。
not逻辑声明——排除
@supports可以使用not逻辑声明,主要作用是,在不支持新特性时,可以提供备用样式。换过来也可以理解,如果你的浏览器不支持@supports条件判断中的样式,你可以通过@supports为浏览器提供一种备用样式,如:
@supports not (display: flex){
#container div{float:left;} }
上面的代码表示的是,如果你的浏览器不支持“display:flex”属性,那么你可以使用“float:left”来替代。
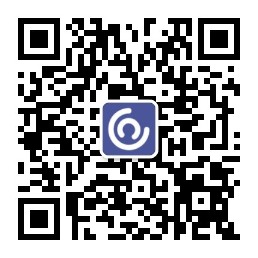
and逻辑声明——联合(与)
@supports的条件判断中也可以使用“and”逻辑声明。用来判断是否支持多个属性。例如:
@supports (column-width: 20rem) and (column-span: all) {
div { column-width: 20rem } div h2 { column-span: all } div h2 + p { margin-top: 0; } ... }
上面的代码表示的是,如果你的浏览器同时支持“column-width:20rem”和“column-span:all”两个条件,浏览器将会调用下面的样式:
div { column-width: 20rem } div h2 { column-span: all } div h2 + p { margin-top: 0; } ...
反之,如果不同时支持这两个条件,那么浏览器将不会调用这部分样式。
注:多个and边接并不需要使用括号:
@supports (display: table-cell) and (display: list-item) and (display:run-in){
/*样式*/
}
or逻辑声明——or(或)
@supports除了“not”和“and”逻辑声明之外,还可以使用“or”逻辑声明。主要用来判断浏览器是否支持某一CSS特性。也就是说,可以使用“or”逻辑声明,同时专声明多个条件,只要其中一个条件成立就会启用@supports中的样式:
@supports (display: -webkit-flex) or (display: -moz-flex) or (display: flex) {
section {
display: -webkit-flex; display: -moz-flex; display: flex; … } }
上面的例子是@supports中“or”运用场景之一。flex在opera和IE10中不需要前缀,而在其他的现代浏览器中还是需要浏览器厂商的前缀,通过上面的使得,浏览器支持“flex”属性就会调用下面的样式:
section {
display: -webkit-flex;
display: -moz-flex;
display: flex;
…
}
上面是有关于@supports的几种语法的使用规则介绍,在使用这几种规则时,有几点需要特别注意:
“or”和“and”混用
在@supports中“or”和“and”混用时,必须使用括号()来区分其优先级:
@supports ((transition-property: color) or (animation-name: foo)) and (transform: rotate(10deg)) {
// ...
}
@supports (transition-property: color) or ((animation-name: foo) and (transform: rotate(10deg))) {
// ...
}
只有一条表达式时必须用括号()
在@supports中,如果条件判断表达式只有一条,那么这条表达式必须使用括号包起来:
@supports (display: flex) { // ... }
在javascript中支持css的@supports的写法是“window.CSS.supports”。CSS.supports规范提供了两种写法。第一种方法包括了两个参数,一个是属性,另一个是属性值:
var supportsFlex = CSS.supports("display", "flex");
第二种写法简单的提供整个字符串的写法:
var supportsFlexAndAppearance = CSS.supports("(display: flex) and (-webkit-appearance: caret)");
在使用javascript的supports,最重要的是先检测他的特性,Opera浏览器使用不同的名称方法:
var supportsCSS = !!((window.CSS && window.CSS.supports) || window.supportsCSS || false);
// 谷歌浏览器
@supports (-webkit-box-reflect:below 10px) { body{ background-color: black; } .circle { width: 100px; height: 100px; background: #0cc; -webkit-clip-path: circle(50% at 50% 50%); } } // 火狐浏览器 @supports not (-webkit-box-reflect:below 10px) { body{ background-color: #fff; } .circle { width: 100px; height: 100px; background: red; border-radius: 100px; } }
@supports not (clip-path: circle(50% at 50% 50%)) { .circle { width: 100px; height: 100px; background: red; } } @supports (-webkit-clip-path: circle(50% at 50% 50%)) { .circle { width: 100px; height: 100px; background: #0cc; clip-path: circle(50% at 50% 50%) } }
html 部分
<div class="circle">口垒起哇</div>
火狐浏览器