1. 光点沿边框运动
<div class="spot-rotate">
<a class="spot-box">点 我</a>
<div class="spark"></div>
</div>
.spot-rotate {
position: relative;
left: 50%;
width: 280px;
height: 400px;
background: blue;
}
.spot-rotate .spot-box {
position:absolute;
display:block;
text-align:center;
line-height:40px;
width:148px;
height:40px;
color:#fff5d1;
border-radius:20px;
border:1px solid#fff;
font-size:20px;
text-decoration:none;
top:0;
left:0px;
right: 0;
bottom: 0;
margin: auto;
}
.spot-rotate .spark {
position: absolute;
width: 30px;
height: 41px;
background: url(../../static/images/spot.png) no-repeat;
top: -40px;
left: -66px;
right: 0;
bottom: 0;
margin: auto;
-webkit-animation-name: spark;
-webkit-transform-origin: 50% 100%;
-webkit-animation-duration: 8s;
-webkit-animation-timing-function: linear;
-webkit-animation-iteration-count: infinite;
-webkit-animation-direction: normal;
}
@-webkit-keyframes
spark
{
0% {
left: -68px;
transform: rotate(0deg);
}
35% {
left: 112px;
transform: rotate(0deg);
}
50% {
left: 112px;
transform:rotate(180deg)
}
85%{
left: -138px;
transform:rotate(180deg)
}
100%{
transform:rotate(360deg)
}
}
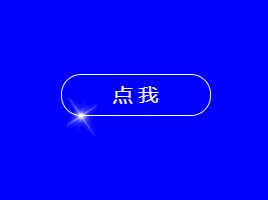
2. 线条沿边框运动
<div class="line-run"></div>
.line-run, .line-run::before, .line-run::after {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
}
.line-run {
width: 200px;
height: 200px;
margin: auto;
color: red;
box-shadow: inset 0 0 0 1px rgba(105, 202, 98, 0.5);
}
.line-run::before, .line-run::after {
content: '';
z-index: -1;
margin: -5%;
box-shadow: inset 0 0 0 2px;
animation: myAni 8s linear infinite;
}
.line-run::before {
animation-delay: -4s;
}
.line-run:hover::after, .line-run:hover::before {
background-color: rgba(255, 0, 0, 0.3);
}
@keyframes myAni {
0%, 100% {
clip: rect(0px, 220.0px, 2px, 0px);
}
25% {
clip: rect(0px, 2px, 220.0px, 0px);
}
50% {
clip: rect(218.0px, 220.0px, 220.0px, 0px);
}
75% {
clip: rect(0px, 220.0px, 220.0px, 218.0px);
}
}
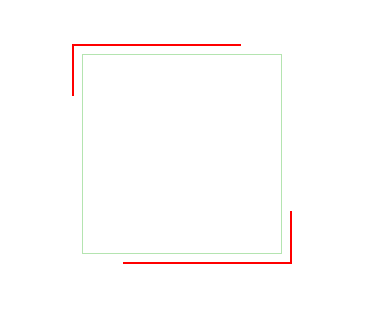
3. 线条从四周向中心靠拢
<div class="border-animation">
<a href="#" class="box-link">
Css 边框动画
<span class="line line_top"></span>
<span class="line line_right"></span>
<span class="line line_bottom"></span>
<span class="line line_left"></span>
</a>
</div>
.border-animation {
background: #000;
width:902px;
margin:40px auto 0 auto;
}
.border-animation .box-link {
display:inline-block;
position:relative;
text-decoration:none;
font-size:15px;
color:#33ab6a;
font-weight:bold;
width:255px;
text-align:center;
height:100px;
line-height:56px;
border:2px solid rgba(255,255,255,.8);
margin:20px 96px;
padding:20px;
-webkit-box-sizing:border-box;
-moz-box-sizing:border-box;
box-sizing:border-box;
-webkit-transition:0.4s;
-o-transition:0.4s;
transition:0.4s;
}
.box-link:hover{
border:2px solid rgba(255,255,255,1);
}
.box-link .line{
display:inline-block;
background-color:#FFF;
position:absolute;
-webkit-transition:0.5s ease;
-o-transition:0.5s ease;
transition:0.5s ease;
}
.box-link .line_top{
height:2px;
width:0;
left:-50%;
top:-2px;
}
.box-link:hover .line_top{
width:100%;
left:-2px;
}
.box-link .line_right{
height:0;
width:2px;
top:-50%;
right:-2px;
}
.box-link:hover .line_right{
height:100%;
top:-2px;
}
.box-link .line_bottom{
width:2px;
height:0;
bottom:-50%;
left:-2px;
}
.box-link:hover .line_bottom{
height:100%;
bottom:-2px;
}
.box-link .line_left{
height:2px;
width:0;
right:-50%;
bottom:-2px;
}
.box-link:hover .line_left{
width:100%;
right:-2px;
}

4. 鼠标悬乎特效
<div class="container">
<button class="corner-button">
<span>Click me</span>
</button>
</div>
.container {
background: #2f2f2f;
width: 80%;
height: 300px;
box-sizing: border-box;
margin: 0 auto;
text-align: center;
padding-top: 5%;
}
.corner-button {
letter-spacing: 0.32px;
cursor: pointer;
background: transparent;
border: 8px solid currentColor;
padding: 24px 32px;
font-size: 35px;
color: #06c17f;
position: relative;
transition: color 0.3s;
}
.corner-button:hover {
color: blue;
}
.corner-button:hover::before {
width: 0;
}
.corner-button:hover::after {
height: 0;
}
.corner-button:active {
border-width: 4px;
}
.corner-button span {
position: relative;
z-index: 2;
}
.corner-button::before,
.corner-button::after {
content: '';
position: absolute;
background: #2f2f2f;
z-index: 1;
transition: all 0.3s;
}
.corner-button::before {
width: calc(100% - 48px);
height: calc(100% + 16px);
top: -8px;
left: 50%;
-webkit-transform: translateX(-50%);
transform: translateX(-50%);
}
.corner-button::after {
height: calc(100% - 48px);
width: calc(100% + 16px);
left: -8px;
top: 50%;
-webkit-transform: translateY(-50%);
transform: translateY(-50%);
}
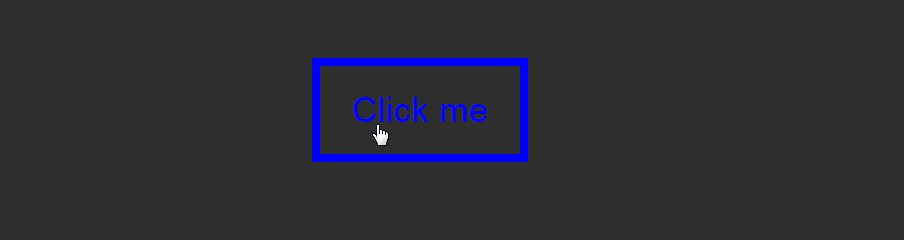