654. Maximum Binary Tree
Given an integer array with no duplicates. A maximum tree building on this array is defined as follow:
- The root is the maximum number in the array.
- The left subtree is the maximum tree constructed from left part subarray divided by the maximum number.
- The right subtree is the maximum tree constructed from right part subarray divided by the maximum number.
Construct the maximum tree by the given array and output the root node of this tree.
------------------------------------------------------------------------------------------------------------------
给定一个没有重复的整数数组。在此阵列上建立的最大树定义如下:
- 根是数组中的最大数目。
- 左边的子树是从左边的子数组中除以最大数目而得到的最大树。
- 右子树是从右部分子数组构造的最大树除以最大数目。
通过给定的数组构造最大树并输出此树的根节点。
------------------------------------------------------------------------------------------------------------------
Example 1:
Input: [3,2,1,6,0,5] Output: return the tree root node representing the following tree: 6 / \ 3 5 \ / 2 0 \ 1
Note:
- The size of the given array will be in the range [1,1000].
题目不算难,可以很容易的想到解决思路:
- 创建一个根节点,其值为数组的最大值
- 创建根节点的左节点,左节点的值为数组左半部分的最大值
- 创建根节点的右节点,右节点的值为数组右半部分的最大值
- 递归以上操作
# Definition for a binary tree node. # class TreeNode: # def __init__(self, x): # self.val = x # self.left = None # self.right = None class Solution: def constructMaximumBinaryTree(self, nums: List[int]) -> TreeNode: if nums ==[]: return None index = nums.index(max(nums)) root = TreeNode(max(nums)) root.left = self.constructMaximumBinaryTree(nums[:index]) root.right = self.constructMaximumBinaryTree(nums[index+1:]) return root
1305. All Elements in Two Binary Search Trees
Given two binary search trees root1
and root2
.
Return a list containing all the integers from both trees sorted in ascending order.
Example 1:
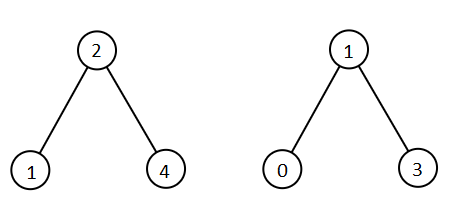
Input: root1 = [2,1,4], root2 = [1,0,3] Output: [0,1,1,2,3,4]
Example 2:
Input: root1 = [0,-10,10], root2 = [5,1,7,0,2] Output: [-10,0,0,1,2,5,7,10]
Example 3:
Input: root1 = [], root2 = [5,1,7,0,2] Output: [0,1,2,5,7]
Example 4:
Input: root1 = [0,-10,10], root2 = [] Output: [-10,0,10]
Example 5:
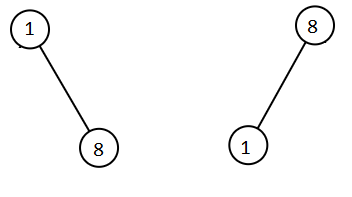
Input: root1 = [1,null,8], root2 = [8,1] Output: [1,1,8,8]
Constraints:
- Each tree has at most
5000
nodes. - Each node's value is between
[-10^5, 10^5]
.