charAt indexOf length startsWith substring 转换大小写 trim valueOf replaceAll split matches StringBuilder
String类介绍
/** * String是不变对象,即: * 字符串对象创建后,表示的字符内容不可变,若 * 改变必定创建新对象. * @author ta * */ public class StringDemo { public static void main(String[] args) { /* * java对于字符串有一个优化,即: * 字符串常量池,这是在堆内存中开辟的 * 一块空间,用于保存所有使用字面量形式 * 创建的字符串对象,当再次使用该字面量 * 创建新的字符串时会直接重用而不会再 * 创建新的来节省内存开销. */ String s1 = "123abc"; //s2会重用s1创建的字符串对象 String s2 = "123abc"; String s3 = "123abc"; System.out.println(s1==s2);//true System.out.println(s1==s3);//true //修改内容会创建新对象 s1 = s1 + "!";//s1不再指向原对象 System.out.println("s1:"+s1);//123abc! System.out.println("s2:"+s2);//123abc System.out.println(s1==s2);//false System.out.println(s2==s3);//true /* * new关键字很强制,一定会创建新对象 */ String s4 = new String("123abc"); System.out.println("s4:"+s4); /* * s2与s4是两个不同的字符串对象,虽然 * 内容都是"123abc",但是两个变量保存 * 的地址不同. */ System.out.println(s4==s2);//false /* * 通常我们比较字符串都是比较字符串的 * 内容,因此比较字符串时我们不会使用 * "==",而是使用字符串的方法equals. * equals方法是用来比较两个字符串对象 * 所表示的内容是否相同的. */ boolean tf = s2.equals(s4); System.out.println(tf);//true /* * 这里用到了编译器的一个特性: * 编译器在编译源代码时,当遇到一个计算 * 表达式可以在编译期间确定结果时就会 * 直接进行计算,并将结果编译到class文件 * 中,这样JVM每次运行程序时就无需再计算. * 比如: int a = 1+1; * 编译器编译后class改为:int a = 2; * 下面的字符串也是如此,会被改为: * String s5 = "123abc"; */ String s5 = "123"+"abc"; System.out.println("s5:"+s5); System.out.println(s2==s5);//true String s = "123"; String s6 = s + "abc"; System.out.println("s6:"+s6); System.out.println(s2==s6);//false } }
1.charAt
/** * char charAt(int index) * 获取当前字符串中指定位置对应的字符 * @author ta * */ public class CharAtDemo { public static void main(String[] args) { // 0123456789012345 String str = "thinking in java"; char c = str.charAt(9); System.out.println(c); //判断回文 0 1 2 3 4 5 6 7 8 String line = "上海自来水来自海上"; for(int i=0;i<line.length()/2;i++) { char c1 = line.charAt(i); char c2 = line.charAt(line.length()-1-i); if(c1!=c2) { System.out.println("不是回文!"); /* * 当方法返回值类型为void时,return是 * 可以单独使用的,目的是结束方法 */ return; } } System.out.println("是回文!"); } }
2.indexOf
/** * int indexOf(String str) * 查找给定字符串在当前字符串中的位置,若当前 * 字符串不包含给定内容则返回值为-1. * @author ta * */ public class IndexOfDemo { public static void main(String[] args) { // 0123456789012345 String str = "thinking in java"; int index = str.indexOf("in"); System.out.println(index);//2 /* * 重载的方法允许我们从指定位置开始 * 查找第一次出现指定字符的位置 */ index = str.indexOf("in", 3); System.out.println(index);//5 //查找最后一次出现指定字符串的位置 index = str.lastIndexOf("in"); System.out.println(index);//9 } }
3.length
/** * int length() * 获取当前字符串的长度(字符个数) * @author ta * */ public class LengthDemo { public static void main(String[] args) { String str = "我爱java!"; int len = str.length(); System.out.println(len); } }
4.startsWith
/** * boolean startsWith(String str) * boolean endsWith(String str) * 判断字符串是否是以给定的字符串开始或结尾的 * @author ta * */ public class StartsWithDemo { public static void main(String[] args) { String str = "thinking in java"; boolean starts = str.startsWith("thi"); System.out.println(starts); boolean ends = str.endsWith("ava"); System.out.println(ends); } }
5.substring
/** * String substring(int start,int end) * 截取当前字符串中指定范围内的字符串. * * 注:java API中,通常使用两个数字表示范围时, * 都是含头不含尾的. * @author ta * */ public class SubstringDemo { public static void main(String[] args) { // 01234567890 String line = "www.eeee.cn"; String sub = line.substring(4,8); System.out.println(sub); //从指定位置开始截取到字符串末尾 sub = line.substring(4); System.out.println(sub); } }
6.转换大小写
/** * String toUpperCase() * String toLowerCase() * 将当前字符串中的英文部分转换为全大写或全小写 * @author ta * */ public class ToUpperCaseDemo { public static void main(String[] args) { String str = "我爱Java"; String upper = str.toUpperCase(); System.out.println(upper); String lower = str.toLowerCase(); System.out.println(lower); } }
7.trim
/** * String trim() * 去除当前字符串两侧的空白字符 * @author ta * */ public class TrimDemo { public static void main(String[] args) { String str = " hello "; String trim = str.trim(); System.out.println(str); System.out.println(trim); } }
8.valueOf
/** * 字符串提供了若干的重载的valueOf方法,它们 * 都是静态方法. * static String valueOf(XXX xxx) * 作用是将给定的内容转换为字符串 * * @author ta * */ public class ValueOfDemo { public static void main(String[] args) { int a = 123; //将int值转换为String String str = String.valueOf(a); System.out.println(str); double dou = 123.123; String str2 = String.valueOf(dou); System.out.println(str2); //任何类型与字符串连接结果都是字符串 str = a+""; System.out.println(str); } }
9.replaceAll
扫描二维码关注公众号,回复:
8325804 查看本文章
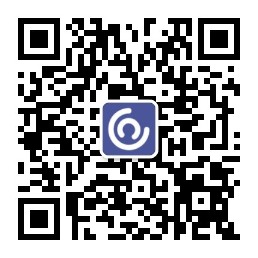
/** * 和谐用语 * @author ta * */ public class Demo2 { public static void main(String[] args) { String regex = "(wqnmlgb|dsb|cnm|nc|nmbwcnm|mdzz|djb)"; String message = "nmbwcnm!你是nc么?你个dsb,你就是一个djb!"; message = message.replaceAll(regex, "***"); System.out.println(message); } }
/** * String支持正则表达式方法三: * String replaceAll(String regex,String str) * 将当前字符串中满足正则表达式的部分替换为 * 给定的内容 * @author ta * */ public class ReplaceAllDemo { public static void main(String[] args) { String line = "abc123def456ghi789jkl"; /* * 将字符串中的数字部分替换为"#NUMBER#" */ line = line.replaceAll("[0-9]+", "#NUMBER#"); System.out.println(line); } }
10.split
import java.util.Arrays; /** * String支持正则表达式方法二: * String[] split(String regex) * 将当前字符串中按照满足正则表达式的部分拆分 * 然后将拆分后的字符串以数组形式返回. * @author ta * */ public class SplitDemo { public static void main(String[] args) { String line = "abc123def456ghi789jkl"; //按照数字部分拆分字符串 // String[] data = line.split("[0-9]+"); /* * 如果拆分过程中连续匹配到两次可拆分的 * 内容时,中间会拆分出一个空字符串. * 但是如果是在字符串末尾连续匹配上则所有 * 拆分出的空字符串会被忽略. */ String[] data = line.split("[0-9]"); System.out.println(data.length); System.out.println(Arrays.toString(data)); } }
11.matches(正则表达式是否匹配)
/** * String支持正则表达式的方法之一: * boolean matches(String regex) * 使用给定的正则表达式匹配当前字符串是否符合 * 格式要求,符合则返回true. * * 注意:给定的正则表达式就算不指定边界匹配符 * 即:(^...$)也是做完全匹配验证的. * @author ta * */ public class MatchesDemo { public static void main(String[] args) { String email = "[email protected]"; /* * [a-zA-Z0-9_]+@[a-zA-Z0-9]+(\.[a-zA-Z]+)+ * */ String regex = "[a-zA-Z0-9_]+@[a-zA-Z0-9]+(\\.[a-zA-Z]+)+"; boolean match = email.matches(regex); if(match) { System.out.println("是邮箱"); }else { System.out.println("不是邮箱"); } } }
12.StringBuilder
/** * String的优化措施仅照顾重用性,因此频繁修改 * 字符串会带来内存开销大,运行效率差的结果. * 对此,java提供了一个专门用于修改字符串的API * java.lang.StringBuilder * 其内部维护一个可变的char数组,所有的修改都是 * 在这个数组中进行的,因此开销小,性能好. * 并且其提供了便于修改字符串的一系列方法,包 * 括了:增,删,改,插等基本操作. * @author ta * */ public class StringBuilderDemo { public static void main(String[] args) { String str = "好好学习java"; StringBuilder builder = new StringBuilder(str); /* * 好好学习java * 好好学习java,为了找个好工作! */ builder.append(",为了找个好工作!"); System.out.println(str); //获取StringBuilder内部的字符串 str = builder.toString(); System.out.println(str); /* * 好好学习java,为了找个好工作! * 好好学习java,就是为了改变世界! */ builder.replace(9, 16, "就是为了改变世界"); System.out.println(builder); /* * 好好学习java,就是为了改变世界! * ,就是为了改变世界! */ builder.delete(0, 8); System.out.println(builder); /* * ,就是为了改变世界! * 活着,就是为了改变世界! */ builder.insert(0, "活着"); System.out.println(builder); } }