上次写了一个多条件查询,内容较为繁琐,而且可读性太差,于是有大佬给我提供了简单的方法。我于是按照大佬的方法重新敲了一遍(说实话就是大部分把人家的复制了一遍),发下有很多东西比之前的简单多了。于是我对JavaWeb的知识又有了更深的了解。
第一次写那个多条件查询难点在于sql语句的拼接和动态增加条件框。
动态增加条件框就是html的拼接,这方面有两个问题,一个是不好排版,第二个是代码重复,因为这部分拼接的代码再table本身就有,所以最终的解决办法就是复制。将原来table一行里的html代码复制,然后再新增一行。当然还有一个关键因素,就是你要改变某列是否可见。刚开始我们的一定得存在,但是新增的一行就不必存在,而且新增的一行需要出现
条件框,于是我们可以先在table里分别设置它们可见和不可见,然后再新增的函数中,再将他们设成相反的即可。
sql语句的拼接也别之前的简单很多,通过数组接受客户端传来的信息,省去手动添加到数组。然后就是String.format()(字符串的占位符),这个我再网上查了资料,我个人觉得和设置sql语句参数相似,比如 sql="select * from where name = ? and sex = ?"; ps.setString(1,"张三");ps.setString(2,"男"); ps(PreparedStatement ps = con.prepareStatement(SQL))。这个占位符很方便,把并拼接字符串省去。在对应的位置替换对应的值。(NB)
当然还有一些不规范,比如命名得用英文,变量名要有意义,见名知意等等。读者可以通过对比,来看差距。(通过名字获取我就没有写了,那个和之前的一样)
上代码
jsp代码:
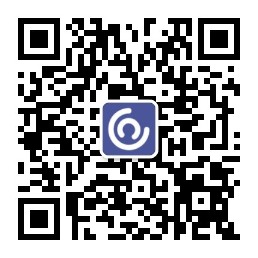
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%> <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>多条件查询</title> </head> <style type="text/css"> body{ background-color:#EBC79E } #table{ font-size:20px; } input[type='text'] { font-size:20px; width: 250px; height: 30px; } input[type='button'] { font-size:20px; width: 50px; height: 30px; } .small_select { width:50px; height:30px; fone-size:30px } .large_select { width:120px; font-size:17px; } </style> <body> <% Object message = request.getAttribute("message"); if(message!=null && !"".equals(message)){ %> <script type="text/javascript"> alert("<%=request.getAttribute("message")%>"); </script> <%} %> <form action="Servlet?method=search" method="post"> <table id="searchTable"> <tr> <td> <div class="div_operate"> <input type="button" value="+" onclick="insertRow()"> <input type="button" value="-" onclick="deleteRow()"> </div> <div class="div_relation" style="display:none"> <select name="relation" class="large_select"> <option value="and">并且</option> <option value="or">或者</option> <option value="not like">不含</option> </select> </div> </td> <td> <select name="field" class="large_select"> <option value="name">姓名</option> <option value="sex">性别</option> <option value="national">民族</option> <option value="political">政治面貌</option> <option value="serviceType">服务类别</option> <option value="registerTime">注册时间</option> </select> </td> <td> <input type="text" name="value"> </td> <td> <select name="symbol" class="small_select"> <option value="=">精确</option> <option value="like">模糊</option> </select> </td> </tr> </table> <input type="submit" value="提交" style="width:50px;height:30px;font-size:17px;"> </form> </body> <script type="text/javascript"> var table_row = 1; function insertRow(){ if(table_row>=6){ return; } var firstRow = searchTable.rows[0]; //获取table第一行 var cloneRow = firstRow.cloneNode(firstRow); // 讲table第一行复制到cloneRow cloneRow.getElementsByClassName("div_operate")[0].style.display="none"; //设置为不可见 cloneRow.getElementsByClassName("div_relation")[0].style.display="inline";// 设置为可见 searchTable.appendChild(cloneRow); // 添加到table table_row++; } function deleteRow(){ if(table_row<=1){ return; } searchTable.deleteRow(table_row-1); // 删除table最后一行 table_row--; } </script> </html>
Servlet
package Servlet; import java.io.IOException; import java.util.List; import javax.servlet.ServletException; import javax.servlet.annotation.WebServlet; import javax.servlet.http.HttpServlet; import javax.servlet.http.HttpServletRequest; import javax.servlet.http.HttpServletResponse; import Bin.Volunteer; import Dao.Dao; /** * Servlet implementation class Servlet1 */ @WebServlet("/Servlet") public class Servlet extends HttpServlet { private static final long serialVersionUID = 1L; /** * @see HttpServlet#HttpServlet() */ protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException { req.setCharacterEncoding("utf-8"); String method = req.getParameter("method"); if(method.equals("search")) { search(req,resp); }else if(method.equals("searchAtName")) { searchAtName(req,resp); } } // 通过多条件查询 private void search(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { request.setCharacterEncoding("utf-8"); String searchSql = "select * from volunteer where 1 = 1 "; String[] field = request.getParameterValues("field"); String[] value = request.getParameterValues("value"); String[] symbol = request.getParameterValues("symbol"); String[] relation = request.getParameterValues("relation"); for (int i = 0; i < relation.length; i++) { if(value[i].equals("")||value==null) { continue; } //查询值默认就是输入值 String searchValue = value[i]; //如果符号是like,再将前后加上% if (symbol[i].equals("like")){ //%%是对%的转义,%s是站位任意字符串 searchValue = String.format("%%%s%%", value[i]); } //使用String.format将关系、字段、符号、值拼接起来,可读性比+好更多 //根据关系是否为not like,调换一下拼接顺序 if (relation[i].equals("not like")){ searchSql += String.format("%s %s %s '%s' ", "and", field[i], relation[i], searchValue); }else { searchSql += String.format("%s %s %s '%s' ", relation[i], field[i], symbol[i], searchValue); } } System.out.println(searchSql); List<Volunteer> list = Dao.Search(searchSql); if(list.size()!=0) { request.setAttribute("list",list); request.getRequestDispatcher("searchResult.jsp").forward(request, response); }else { request.setAttribute("message","不存在符合条件的志愿者"); request.getRequestDispatcher("index.jsp").forward(request, response); } } // 通过单条件查询 private void searchAtName(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException{ request.setCharacterEncoding("utf-8"); String name = request.getParameter("name"); Volunteer volunteer = Dao.searchAtName(name); if(volunteer!=null) { request.setAttribute("volunteer", volunteer); request.getRequestDispatcher("searchResult.jsp").forward(request, response); } } }
dao层和上次的一样(当然你得把名改了)
package Dao; import java.sql.Connection; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.util.ArrayList; import java.util.List; import Bin.Volunteer; import DBUtil.Util; public class Dao { // 通过多条件查询 public static List<Volunteer> Search(String SQL){ Connection con = null; PreparedStatement ps = null; ResultSet rs = null; List<Volunteer> list = new ArrayList<Volunteer>(); try { // 获取连接 con = Util.getConnection(); // 执行sql语句 ps = con.prepareStatement(SQL); // 执行查询操作 rs = ps.executeQuery(); while(rs.next()) { Volunteer volunteer = new Volunteer(); volunteer.setName(rs.getString(1)); volunteer.setSex(rs.getString(2)); volunteer.setNational(rs.getString(3)); volunteer.setPolitical(rs.getString(4)); volunteer.setServiceType(rs.getString(5)); volunteer.setRegisterTime(rs.getString(6)); list.add(volunteer); } return list; } catch (SQLException e) { e.printStackTrace(); } try { if(rs!=null) { rs.close(); } Util.release(con, ps); } catch (SQLException e) { e.printStackTrace(); } return null; } // 通过名字查询 public static Volunteer searchAtName(String name) { Connection con = null; PreparedStatement ps = null; ResultSet rs = null; try { // 获取连接 con = Util.getConnection(); // 编写sql语句 String sql = "select * from volunteer where name = ?"; // 执行sql语句 ps = con.prepareStatement(sql); // 设置参数 ps.setString(1, name); // 执行查询操作 rs = ps.executeQuery(); while(rs.next()) { Volunteer volunteer = new Volunteer(); volunteer.setName(rs.getString(1)); volunteer.setSex(rs.getString(2)); volunteer.setNational(rs.getString(3)); volunteer.setPolitical(rs.getString(4)); volunteer.setServiceType(rs.getString(5)); volunteer.setRegisterTime(rs.getString(6)); return volunteer; } } catch (SQLException e) { e.printStackTrace(); } try { if(rs!=null) { rs.close(); } Util.release(con, ps); } catch (SQLException e) { e.printStackTrace(); } return null; } }
DBUtil(链接数据库的)
package DBUtil; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.SQLException; public class Util { // 获取连接 // 获取连接方法 public static Connection getConnection() { String driver = "com.mysql.jdbc.Driver"; String url = "jdbc:mysql://localhost:3306/tree?useSSL=false&useUnicode=true&characterEncoding=utf8"; String username = "root"; String password = "a3685371"; Connection con = null; try { Class.forName(driver); con = DriverManager.getConnection(url,username,password); }catch(Exception e) { throw new RuntimeException(e); } return con; } // 释放资源 public static void release(Connection con,PreparedStatement ps) { if(ps!=null) { try { ps.close(); } catch (SQLException e) { e.printStackTrace(); } } if(con!=null) { try { con.close(); } catch (SQLException e) { e.printStackTrace(); } } } }
代码博大精深,没有最好只有更好(大佬说的),只有一点一点提升自己,不断优化自己的代码,不断学习,才会月薪好几十K。
每天学习一些,天天都在进步!!!!