一、链表实现增删改查
1、链表定义
1 #include<stdio.h> 2 #include<string.h> 3 #include<windows.h> 4 #include<stdlib.h> 5 #define maxn 10 6 #define N 100005 7 typedef struct //歌曲信息 8 { 9 char author[20],style[20],name[20],belong[50]; 10 int is; 11 } songs; 12 typedef struct Sqlist //曲库链表 13 { 14 songs data; 15 struct Sqlist *next; 16 }; 17 typedef struct sang //点歌链表 18 { 19 songs data; 20 struct sang *next; 21 }; 22 struct sang *que,*str2,*s2,*s22; 23 struct Sqlist *r,*str1,*s1,*s11;
不要问为什么定义的都是指针类型结构体变量,因为一些变量定义指针类型,一些变量定义结构体类型。写着写着我就搞错了。。。。(是我的问题。。。)
链表最重要就是它不需要一次性定义好多此类型结构体,只需要用到一个开一个空间就可以了
2、初始化
1 int Create() //为各链表分配空间 2 { 3 r=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 4 que=(struct sang *)malloc(sizeof(struct sang)*1); 5 que->next=NULL; 6 r->next=NULL; 7 init(); 8 return 1; 9 }
这是两个链表,一个链表头为que、另一个链表头为r
3、增加数据(以链表r来举例子)
1 void Add() //增添曲库内歌曲 2 { 3 system("cls"); 4 str1=r; 5 while(str1->next!=NULL) 6 { 7 str1=str1->next; 8 } 9 s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 10 printf("请按顺序输入以下内容\n"); 11 printf("歌名:作者:曲风:语种:\n"); 12 scanf("%s%s%s%s",s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 13 s1->data.is=0; 14 s1->next=NULL; 15 str1->next=s1; 16 printf("添加成功\n"); 17 system("pause"); 18 }
这里要注意我们定义的s1是一个结构体指针,那么定义的时候是不会给他分配一个此结构体类型的空间的。换句话说,s1指向的地址是随机的。
所以我们要给s1开一个此结构体类型的空间,把里面放满数据。然后加在链表尾部。
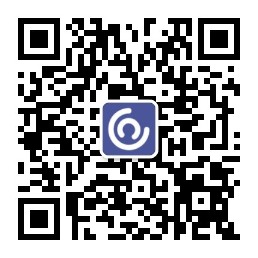
注意:这个过程中链表的头节点r指向的地址可不能变。所以要用一个此结构体类型指针去遍历这个链表(我代码中用的是str1)
4、删除
1 void Delete() //删除曲库内歌曲 2 { 3 4 int i,id; 5 system("cls"); 6 printf("输入你要删除歌曲的编号\n"); 7 scanf("%d",&id); 8 printf("编号:歌名:作者:曲风:语种:\n"); 9 str1=r; 10 i=0; 11 while(str1->next!=NULL) 12 { 13 14 if(i==id) 15 { 16 s1=str1->next; 17 printf("%d %s %s %s %s\n",i,s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 18 printf("你确定要删除吗?确定输入1,否则输入0\n"); 19 scanf("%d",&id); 20 if(id) 21 { 22 str1->next=s1->next; 23 free(s1); 24 } 25 break; 26 } 27 str1=str1->next; 28 i++; 29 } 30 system("pause"); 31 }
删除操作的进行需要找到两个位置
1、要删除节点的上一个节点(代码中这个位置由str1来保存)
2、要删除的这个节点 (代码中这个位置由s1来保存)
然后让 (上一个节点指向下一个节点的指针) 指向 (要删除节点指向下一个节点的指针)
理解一波。。。
5、修改
1 void Modify() //修改曲库 2 { 3 4 int i,id; 5 system("cls"); 6 printf("输入你要修改歌曲的编号\n"); 7 scanf("%d",&id); 8 printf("编号:歌名:作者:曲风:语种:\n"); 9 str1=r; 10 i=0; 11 while(str1->next!=NULL) 12 { 13 14 if(i==id) 15 { 16 s1=str1->next; 17 printf("%d %s %s %s %s\n",i,s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 18 printf("你确定要修改吗?确定输入1,否则输入0\n"); 19 scanf("%d",&id); 20 if(id) 21 { 22 printf("依次输入歌名:作者:曲风:语种:\n"); 23 scanf("%s%s%s%s",&s1->data.name,&s1->data.author,&s1->data.style,s1->data.belong); 24 } 25 break; 26 } 27 str1=str1->next; 28 i++; 29 } 30 system("pause"); 31 }
修改就没什么讲的了,和删除操作代码大致上同
6、查
1 void Show() 2 { 3 4 int i; 5 system("cls"); 6 printf("编号:歌名:作者:曲风:语种:\n"); 7 str1=r; 8 i=0; 9 while(str1->next!=NULL) 10 { 11 str1=str1->next; 12 printf("%d %s %s %s %s\n",i++,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 13 } 14 15 system("pause"); 16 }
7、文件读写
void init() //导入文件内容 { //第一个链表文件读取 int i=0; char ch; FILE *fp=NULL; fp=fopen("songs.txt","a+"); str1=r; s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); while(fscanf(fp,"%s%s%s%s",s1->data.name,s1->data.author,s1->data.style,s1->data.belong)!=EOF) { s1->data.is=0; s1->next=NULL; str1->next=s1; str1=str1->next; s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); } fclose(fp); //第二个链表文件读取 fp=fopen("sangs.txt","a+"); str2=que; s2=(struct sang *)malloc(sizeof(struct sang)*1); while(fscanf(fp,"%s%s%s%s",s2->data.name,s2->data.author,s2->data.style,s2->data.belong)!=EOF) { s2->data.is=0; s2->next=NULL; str2->next=s2; str2=str2->next; s2=(struct sang *)malloc(sizeof(struct sang)*1); } fclose(fp); } void Quit() //导出数据 { //第一个链表文件写入 int i; FILE *fp=NULL; system("cls"); fp=fopen("songs.txt","w"); str1=r; while(str1->next!=NULL) { str1=str1->next; fprintf(fp,"%s %s %s %s\n",str1->data.name,str1->data.author,str1->data.style,str1->data.belong); } fclose(fp); //第二个链表文件写入 fp=fopen("sangs.txt","w"); str2=que; while(str2->next!=NULL) { str2=str2->next; fprintf(fp,"%s %s %s %s\n",str2->data.name,str2->data.author,str2->data.style,str2->data.belong); } fclose(fp); printf("文件保存成功,程序运行结束\n"); system("pause"); }
二、C语言编写程序(链表实现)
(1) 录入歌曲语种分类信息,包括:中文,英文,日文,韩文,小语种;
(2) 录入、修改歌曲信息,包括:歌曲编号,歌曲名,演唱者,曲风;删除歌曲;
(3) 可以按歌曲语种分类信息显示歌曲信息。
(4) 可以根据演唱者查询指定演唱者的所有歌曲信息;根据曲风查询指定曲风的所有歌曲信息。
(5) 创建点歌列表。在曲库中按演唱者或曲风进行搜索,若查找成功将此歌曲添加到点歌链表中。
(6) 优先指定歌曲。在点歌列表中选定优先歌曲,将该歌曲移至点歌列表中的指定位置。
(7) 删除点歌列表中歌曲。
4、完成所有功能并能适当添加或完善功能,且理解代码,90分
(界面友好、系统健壮加1~10分不等)
代码:

1 #include<stdio.h> 2 #include<string.h> 3 #include<windows.h> 4 #include<stdlib.h> 5 #define maxn 10 6 #define N 100005 7 typedef struct //歌曲信息 8 { 9 char author[20],style[20],name[20],belong[50]; 10 int is; 11 } songs; 12 typedef struct Sqlist //曲库链表 13 { 14 songs data; 15 struct Sqlist *next; 16 }; 17 typedef struct sang //点歌链表 18 { 19 songs data; 20 struct sang *next; 21 }; 22 struct sang *que,*str2,*s2,*s22; 23 struct Sqlist *r,*str1,*s1,*s11; 24 int manager_na[20],manager_pa[20],len1; //管理员信息 25 void init() //导入文件内容 26 { 27 int i=0; 28 char ch; 29 FILE *fp=NULL; 30 fp=fopen("songs.txt","a+"); 31 str1=r; 32 s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 33 while(fscanf(fp,"%s%s%s%s",s1->data.name,s1->data.author,s1->data.style,s1->data.belong)!=EOF) 34 { 35 s1->data.is=0; 36 s1->next=NULL; 37 str1->next=s1; 38 str1=str1->next; 39 s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 40 } 41 fclose(fp); 42 43 fp=fopen("sangs.txt","a+"); 44 str2=que; 45 s2=(struct sang *)malloc(sizeof(struct sang)*1); 46 while(fscanf(fp,"%s%s%s%s",s2->data.name,s2->data.author,s2->data.style,s2->data.belong)!=EOF) 47 { 48 s2->data.is=0; 49 s2->next=NULL; 50 str2->next=s2; 51 str2=str2->next; 52 s2=(struct sang *)malloc(sizeof(struct sang)*1); 53 } 54 fclose(fp); 55 } 56 int Create() //为各链表分配空间 57 { 58 r=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 59 que=(struct sang *)malloc(sizeof(struct sang)*1); 60 que->next=NULL; 61 r->next=NULL; 62 init(); 63 return 1; 64 } 65 void Add() //增添曲库内歌曲 66 { 67 system("cls"); 68 str1=r; 69 while(str1->next!=NULL) 70 { 71 str1=str1->next; 72 } 73 s1=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 74 printf("请按顺序输入以下内容\n"); 75 printf("歌名:作者:曲风:语种:\n"); 76 scanf("%s%s%s%s",s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 77 s1->data.is=0; 78 s1->next=NULL; 79 str1->next=s1; 80 printf("添加成功\n"); 81 system("pause"); 82 } 83 void Show() 84 { 85 86 int i; 87 system("cls"); 88 printf("编号:歌名:作者:曲风:语种:\n"); 89 str1=r; 90 i=0; 91 while(str1->next!=NULL) 92 { 93 str1=str1->next; 94 printf("%d %s %s %s %s\n",i++,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 95 } 96 97 system("pause"); 98 } 99 void Delete() //删除曲库内歌曲 100 { 101 102 int i,id; 103 system("cls"); 104 printf("输入你要删除歌曲的编号\n"); 105 scanf("%d",&id); 106 printf("编号:歌名:作者:曲风:语种:\n"); 107 str1=r; 108 i=0; 109 while(str1->next!=NULL) 110 { 111 112 if(i==id) 113 { 114 s1=str1->next; 115 printf("%d %s %s %s %s\n",i,s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 116 printf("你确定要删除吗?确定输入1,否则输入0\n"); 117 scanf("%d",&id); 118 if(id) 119 { 120 str1->next=s1->next; 121 free(s1); 122 } 123 break; 124 } 125 str1=str1->next; 126 i++; 127 } 128 system("pause"); 129 } 130 void Modify() //修改曲库 131 { 132 133 int i,id; 134 system("cls"); 135 printf("输入你要修改歌曲的编号\n"); 136 scanf("%d",&id); 137 printf("编号:歌名:作者:曲风:语种:\n"); 138 str1=r; 139 i=0; 140 while(str1->next!=NULL) 141 { 142 143 if(i==id) 144 { 145 s1=str1->next; 146 printf("%d %s %s %s %s\n",i,s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 147 printf("你确定要修改吗?确定输入1,否则输入0\n"); 148 scanf("%d",&id); 149 if(id) 150 { 151 printf("依次输入歌名:作者:曲风:语种:\n"); 152 scanf("%s%s%s%s",&s1->data.name,&s1->data.author,&s1->data.style,s1->data.belong); 153 } 154 break; 155 } 156 str1=str1->next; 157 i++; 158 } 159 system("pause"); 160 } 161 void Show_style() 162 { 163 164 int i,id; 165 char s[50]; 166 system("cls"); 167 printf("请输入你找查找的曲风\n"); 168 scanf("%s",s); 169 printf("编号:歌名:作者:曲风:语种:\n"); 170 str1=r; 171 i=0; 172 while(str1->next!=NULL) 173 { 174 str1=str1->next; 175 if(strcmp(s,str1->data.style)==0) 176 { 177 printf("%d %s %s %s %s\n",i,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 178 179 } 180 i++; 181 } 182 system("pause"); 183 } 184 void zengjia() //删除曲库内歌曲 185 { 186 187 int i,id; 188 system("cls"); 189 printf("输入你要移动歌曲的编号(会移动至点歌列表表首)\n"); 190 scanf("%d",&id); 191 printf("编号:歌名:作者:曲风:语种:\n"); 192 str1=r; 193 i=0; 194 while(str1->next!=NULL) 195 { 196 197 if(i==id) 198 { 199 s1=str1->next; 200 printf("%d %s %s %s %s\n",i,s1->data.name,s1->data.author,s1->data.style,s1->data.belong); 201 printf("你确定要添加到点歌列表吗?确定输入1,否则输入0\n"); 202 scanf("%d",&id); 203 if(id) 204 { 205 str2=que->next; 206 s2=(struct Sqlist *)malloc(sizeof(struct Sqlist)*1); 207 strcpy(s2->data.name,str1->data.name); 208 strcpy(s2->data.author,str1->data.author); 209 strcpy(s2->data.belong,str1->data.belong); 210 strcpy(s2->data.style,str1->data.style); 211 s2->data.is=0; 212 s2->next=str2; 213 que->next=s2; 214 printf("添加成功\n"); 215 } 216 } 217 str1=str1->next; 218 i++; 219 } 220 system("pause"); 221 } 222 void Show_name() 223 { 224 225 int i,id; 226 char s[50]; 227 system("cls"); 228 printf("请输入你找查找的曲风\n"); 229 scanf("%s",s); 230 printf("编号:歌名:作者:曲风:语种:\n"); 231 str1=r; 232 i=0; 233 while(str1->next!=NULL) 234 { 235 str1=str1->next; 236 if(strcmp(s,str1->data.name)==0) 237 { 238 printf("%d %s %s %s %s\n",i,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 239 240 } 241 i++; 242 } 243 system("pause"); 244 } 245 void Show_author() 246 { 247 248 int i,id; 249 char s[50]; 250 system("cls"); 251 printf("请输入你找查找的曲风\n"); 252 scanf("%s",s); 253 printf("编号:歌名:作者:曲风:语种:\n"); 254 str1=r; 255 i=0; 256 while(str1->next!=NULL) 257 { 258 str1=str1->next; 259 if(strcmp(s,str1->data.author)==0) 260 { 261 printf("%d %s %s %s %s\n",i,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 262 263 } 264 i++; 265 } 266 system("pause"); 267 } 268 void Show_belong() 269 { 270 int i,id; 271 char s[50]; 272 system("cls"); 273 printf("请输入你找查找的曲风\n"); 274 scanf("%s",s); 275 printf("编号:歌名:作者:曲风:语种:\n"); 276 str1=r; 277 i=0; 278 while(str1->next!=NULL) 279 { 280 str1=str1->next; 281 if(strcmp(s,str1->data.belong)==0) 282 { 283 printf("%d %s %s %s %s\n",i,str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 284 285 } 286 i++; 287 } 288 system("pause"); 289 } 290 void Del() //删除曲库内歌曲 291 { 292 293 int i,id; 294 system("cls"); 295 printf("编号:歌名:作者:曲风:语种:\n"); 296 str2=que; 297 i=0; 298 while(str2->next!=NULL) 299 { 300 str2=str2->next; 301 printf("%d %s %s %s %s\n",i++,str2->data.name,str2->data.author,str2->data.style,str2->data.belong); 302 } 303 printf("输入你要删除歌曲的编号(输入-1则退出)\n"); 304 scanf("%d",&id); 305 if(id==-1) return; 306 printf("编号:歌名:作者:曲风:语种:\n"); 307 str2=que; 308 i=0; 309 while(str2->next!=NULL) 310 { 311 312 if(i==id) 313 { 314 s2=str2->next; 315 printf("%d %s %s %s %s\n",i,s2->data.name,s2->data.author,s2->data.style,s2->data.belong); 316 printf("你确定要删除吗?确定输入1,否则输入0\n"); 317 scanf("%d",&id); 318 if(id) 319 { 320 str2->next=s2->next; 321 free(s2); 322 } 323 break; 324 } 325 str2=str2->next; 326 i++; 327 } 328 system("pause"); 329 } 330 void Quit() //导出数据 331 { 332 int i; 333 FILE *fp=NULL; 334 system("cls"); 335 fp=fopen("songs.txt","w"); 336 str1=r; 337 while(str1->next!=NULL) 338 { 339 str1=str1->next; 340 fprintf(fp,"%s %s %s %s\n",str1->data.name,str1->data.author,str1->data.style,str1->data.belong); 341 } 342 fclose(fp); 343 344 fp=fopen("sangs.txt","w"); 345 str2=que; 346 while(str2->next!=NULL) 347 { 348 str2=str2->next; 349 fprintf(fp,"%s %s %s %s\n",str2->data.name,str2->data.author,str2->data.style,str2->data.belong); 350 } 351 fclose(fp); 352 printf("文件保存成功,程序运行结束\n"); 353 system("pause"); 354 } 355 void iiinit() //初始化管理员信息 356 { 357 //管理员信息 358 len1=1; 359 manager_na[0]=123456; 360 manager_pa[0]=123456; 361 } 362 int login() //身份证注册 363 { 364 int i,j,x,na,pa; 365 char name[20],pass[20]; 366 system("cls"); 367 printf("管理员登陆输入2,输入其他直接登录\n"); 368 scanf("%d",&x); 369 if(x==2) 370 { 371 printf("输入用户名\n"); 372 scanf("%d",&na); 373 for(i=0; i<len1; ++i) 374 { 375 if(manager_na[i]==na) 376 { 377 printf("输入密码\n"); 378 scanf("%d",&pa); 379 if(manager_pa[i]==pa) 380 { 381 printf("登陆成功"); 382 system("pause"); 383 return 2; 384 } 385 } 386 } 387 printf("没有这个用户\n"); 388 } 389 printf("登陆成功"); 390 return 0; 391 system("pause"); 392 } 393 void Menu() 394 { 395 int flag; 396 int x; 397 int y=Create(); 398 if(!y) return; 399 iiinit(); 400 flag=login(); 401 while(1) 402 { 403 system("cls"); 404 if(flag==2) 405 { 406 printf ( " \n"); 407 printf ( " \n"); 408 printf ( " \n"); 409 printf ("-------------------------------------- \n"); 410 printf ("--------------------------------------\n"); 411 printf ("--------丨[0]查看所有曲库 丨---\n"); 412 printf ("--------丨[1]删除曲库歌曲 丨---\n"); 413 printf ("--------丨[2]修改曲库歌曲 丨---\n"); 414 printf ("--------丨[3]增加曲库歌曲 丨---\n"); 415 printf ("--------丨[4]查找歌曲(歌名) 丨---\n"); 416 printf ("--------丨[5]查找歌曲(演唱者) 丨---\n"); 417 printf ("--------丨[6]查找歌曲(曲风) 丨---\n"); 418 printf ("--------丨[7]查找歌曲(语种) 丨---\n"); 419 printf ("--------丨[8]修改点歌列表顺序 丨---\n"); 420 printf ("--------丨[9]删除点歌列表中歌曲 丨---\n"); 421 printf ("--------丨[10]结束 丨---\n"); 422 printf ("----------输入相应数字----------------\n"); 423 printf ("--------------------------------------- \n"); 424 printf ( " \n"); 425 printf ( " \n"); 426 scanf("%d",&x); 427 if(x==0) 428 { 429 Show(); 430 } 431 else if(x==1) 432 { 433 Delete(); 434 } 435 else if(x==2) 436 { 437 Modify(); 438 } 439 else if(x==3) 440 { 441 Add(); 442 } 443 else if(x==4) 444 { 445 Show_name(); 446 } 447 else if(x==5) 448 { 449 Show_author(); 450 } 451 else if(x==6) 452 { 453 Show_style(); 454 } 455 else if(x==7) 456 { 457 Show_belong(); 458 } 459 else if(x==8) 460 { 461 zengjia(); 462 } 463 else if(x==9) 464 { 465 Del(); 466 } 467 else if(x==10) 468 { 469 Quit(); 470 return; 471 } 472 } 473 else 474 { 475 printf ( " \n"); 476 printf ( " \n"); 477 printf ( " \n"); 478 printf ("-------------------------------------- \n"); 479 printf ("--------------------------------------\n"); 480 printf ("--------丨[0]查看所有曲库 丨---\n"); 481 printf ("--------丨[1]查找歌曲(歌名) 丨---\n"); 482 printf ("--------丨[2]查找歌曲(演唱者) 丨---\n"); 483 printf ("--------丨[3]查找歌曲(曲风) 丨---\n"); 484 printf ("--------丨[4]查找歌曲(语种) 丨---\n"); 485 printf ("--------丨[5]修改点歌列表顺序 丨---\n"); 486 printf ("--------丨[6]删除点歌列表中歌曲 丨---\n"); 487 printf ("--------丨[7]结束 丨---\n"); 488 printf ("----------输入相应数字----------------\n"); 489 printf ("--------------------------------------- \n"); 490 printf ( " \n"); 491 printf ( " \n"); 492 scanf("%d",&x); 493 if(x==0) 494 { 495 Show(); 496 } 497 else if(x==1) 498 { 499 Show_name(); 500 } 501 else if(x==2) 502 { 503 Show_author(); 504 } 505 else if(x==3) 506 { 507 Show_style(); 508 } 509 else if(x==4) 510 { 511 Show_belong(); 512 } 513 else if(x==5) 514 { 515 zengjia(); 516 } 517 else if(x==6) 518 { 519 Del(); 520 } 521 else if(x==7) 522 { 523 Quit(); 524 return; 525 } 526 } 527 } 528 return; 529 } 530 int main() 531 { 532 Menu(); 533 return 0; 534 }