class的概念
一、我们为什么要用到class类?
因为通过class类来创建对象,使得开发者不必写重复的代码,以达到代码复用的目的。它基于的逻辑是,两个或多个对象的结构功能类似,可以抽象出一个模板,
依照模板复制出多个相似的对象。就像汽车制造商一遍一遍地复用相同的模板来制造大量的汽车车,我们暂且把class理解为一个模板。
但是为什么我们不用function函数用来重复使用呢?
因为funcion声明是需要状态提升的,而class不是,class需要先声明再使用。
二、class类的语法
1 class Student{ 2 constructor(name,age,sex){ 3 this.name = name 4 this.age= age 5 this.sex = sex 6 } 7 8 read(){console.log(this.name+this.age+this.sex)} 9 } 10 var Tom =new Student('tom',21,'男') 11 Tom.read()
Tom是通过类Student实例化出来的对象。对象Tom是按照Student这个模板,实例化出来的对象。实例化出来的对象拥有预先定制好的结构和功能。
2.1 constructor 构造方法
constructor方法是一个特殊的方法,用来创建并初始化一个对象。在一个class中只能有一个命名为constructor的特殊方法,如果包含多个将会报错。
constructor中可以通过super关键字,调用父类的constructor方法。
2.2 static (静态方法)
通过static关键字为一个class创建静态方法
1 class student{ 2 //静态属性 3 static p = 2; 4 //构造方法 5 constructor(name,age,sex){ 6 this.name=name 7 this.age=age 8 this.sex=sex 9 } 10 //实例方法 dream(){console.log('月薪过万。')} 12 } 13 // 静态属性调用 14 console.log(student.p)
2.3 类的继承 extends
1 class A { 2 constructor(){ 3 this.name = 'Marry' 4 this.age= 18 5 } 6 read(){console.log(this.name+this.age)} 7 } 8 class B extends A { 9 constructor(props) { 10 super(props) 11 } 12 s(){ 13 console.log(this.name+this.age) 14 } 15 } 16 var b = new B(); 17 b.s()
当实例 b 调用 s 方法时,b 本身没有 name和age,会根据继承找到A
2.4“this”指向问题
class中的this指向实例时的对象。
扫描二维码关注公众号,回复:
8233833 查看本文章
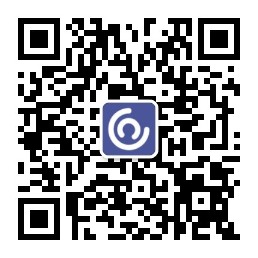
2.5 super( )关键字
关键字super用于调用父类相应的方法,这是相较于原型继承的一个好处
三.总体的写法
1 // 创建一个类存放特有的属性和方法,用来实例对象。 2 class Student{ 3 // 静态属性只属于Student的属性 4 static s = "180"; 5 // 静态方法只属于Student的方法 6 static m(){ 7 console.log("静态方法") 8 } 9 // 构造方法 10 constructor(props){ 11 //实例属性 12 this.name=props.name; 13 this.age=props.age; 14 this.sex=props.sex; 15 } 16 // 实例方法 17 students(){ 18 console.log(this.name+this.age+this.sex) 19 20 } 21 } 22 // 静态属性调用 23 console.log(Student.s) 24 // 实例化对象 25 var S1 = new Student('tom',21,'男'); 26 // 调用方法 27 S1.students()
以上纯属个人理解,如有疑问欢迎留言