检测map容器是否为空:
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 //检测容器是否为空 8 map<string, string>mapText; 9 cout << mapText.empty() << endl; // mapText.empty()==0 空 mapText.empty()!=0 非空 10 11 mapText["小A"] = "A"; //赋值 12 mapText["小B"] = "B"; //赋值 13 mapText["小C"] = "C"; //赋值 14 cout << mapText.empty() << endl; // mapText.empty()==0 空 mapText.empty()!=0 非空 15 16 system("pause"); 17 return 0; 18 }
重复赋值,值被替换:
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 mapText["小A"] = "B"; //重复赋值 10 cout << mapText["小A"] << endl; 11 12 system("pause"); 13 return 0; 14 }
判断键是否存在,如果不存在再赋值:
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 //先检测键是否存在,如果存在则不赋值 10 if (mapText.count("小A") == 0) //count==0不存在 count==1存在 11 { 12 mapText["小A"] = "B"; //重复赋值 13 } 14 cout << mapText["小A"] << endl; 15 16 system("pause"); 17 return 0; 18 }
map循环遍历:
map.begin()指向map的第一个元素
map.end()指向map的最后一个元素之后的地址
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 mapText["小B"] = "B"; //赋值 10 mapText["小C1"] = "C"; //赋值 11 mapText["小C2"] = "C"; //赋值 12 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 13 { 14 cout << "key = " << itor->first << ", value = " << itor->second << endl; 15 16 } 17 system("pause"); 18 return 0; 19 }
map 通过“键”删除键值对:
扫描二维码关注公众号,回复:
8227341 查看本文章
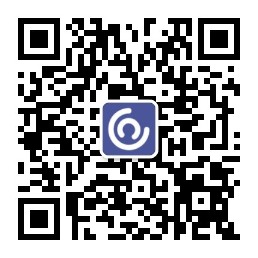
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 mapText["小B"] = "B"; //赋值 10 mapText["小C1"] = "C"; //赋值 11 mapText["小C2"] = "C"; //赋值 12 cout << "删除前:" << endl; 13 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 14 { 15 cout << "key = " << itor->first << ", value = " << itor->second << endl; 16 17 } 18 //删除小B 19 mapText.erase("小B"); 20 cout << "删除后:" << endl; 21 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 22 { 23 cout << "key = " << itor->first << ", value = " << itor->second << endl; 24 25 } 26 mapText.erase("小B"); //小B不存在,erase也不会报错 27 system("pause"); 28 return 0; 29 }
map 通过“值”删除键值对,先写一个错误用法,这里要注意:
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 mapText["小B"] = "B"; //赋值 10 mapText["小C1"] = "C"; //赋值 11 mapText["小C2"] = "C"; //赋值 12 cout << "删除前:" << endl; 13 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 14 { 15 cout << "key = " << itor->first << ", value = " << itor->second << endl; 16 17 } 18 //删除值为C的元素 19 //错误用法: 20 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 21 { 22 if ((itor->second) == "C") 23 { 24 mapText.erase(itor); 25 } 26 } 27 28 cout << "删除后:" << endl; 29 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 30 { 31 cout << "key = " << itor->first << ", value = " << itor->second << endl; 32 33 } 34 system("pause"); 35 return 0; 36 }
错误原因:itor指针在元素被删除后失效了,回到for语句中与mapText.end()进行比较出现错误。
map 通过“值”删除键值对,正确的用法:
1 #include <iostream> 2 #include<map> 3 #include<string> 4 using namespace std; 5 int main() 6 { 7 map<string, string>mapText; 8 mapText["小A"] = "A"; //赋值 9 mapText["小B"] = "B"; //赋值 10 mapText["小C1"] = "C"; //赋值 11 mapText["小C2"] = "C"; //赋值 12 cout << "删除前:" << endl; 13 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 14 { 15 cout << "key = " << itor->first << ", value = " << itor->second << endl; 16 17 } 18 //删除值为C的元素 19 //正确用法: 20 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); /*++itor*/) 21 { 22 if ((itor->second) == "C") 23 { 24 itor = mapText.erase(itor); 25 } 26 else 27 { 28 ++itor; 29 } 30 } 31 32 33 cout << "删除后:" << endl; 34 for (map<string, string>::iterator itor = mapText.begin(); itor != mapText.end(); ++itor) 35 { 36 cout << "key = " << itor->first << ", value = " << itor->second << endl; 37 38 } 39 system("pause"); 40 return 0; 41 }
删除map的第一个元素
mapText.erase(mapText.begin());