租车信息:
输出结果:
代码:
1、先定义抽象类(汽车类:Moto)
1 package cn.aura.demo01; 2 3 public abstract class Moto { 4 //公共属性 5 private String id;//车牌号 6 private String brand;//品牌 7 private int preRent;//日租金 8 //构造方法 9 public Moto(String id, String brand, int preRent) { 10 this.id = id; 11 this.brand = brand; 12 this.preRent = preRent; 13 } 14 //set和get方法 15 public String getId() { 16 return id; 17 } 18 public void setId(String id) { 19 this.id = id; 20 } 21 public String getBrand() { 22 return brand; 23 } 24 public void setBrand(String brand) { 25 this.brand = brand; 26 } 27 public int getPreRent() { 28 return preRent; 29 } 30 public void setPreRent(int preRent) { 31 this.preRent = preRent; 32 } 33 //计算租金方法 34 public abstract double calRent(int days); 35 36 @Override 37 public String toString() { 38 return "Moto [id=" + id + ", brand=" + brand + ", preRent=" + preRent + "]"; 39 } 40 41 }
2.定义轿车类继承汽车类
1 package cn.aura.demo01; 2 /** 3 * 轿车类 4 */ 5 public class Car extends Moto{ 6 //私有属性 7 private String type;//型号 8 //set和get方法 9 public String getType() { 10 return type; 11 } 12 public void setType(String type) { 13 this.type = type; 14 } 15 //构造方法 16 public Car(String id, String brand, String type,int preRent) { 17 super(id, brand, preRent); 18 this.type = type; 19 } 20 21 //重写计算租金的算法 22 @Override 23 public double calRent(int days) { 24 if (days > 7 && days <= 30) { 25 //days大于7天,9折 26 return getPreRent()*days*0.9; 27 }else if (days > 30 && days <= 150) { 28 //days大于30天,8折 29 return getPreRent()*days*0.8; 30 }else if (days > 150) { 31 //days大于150天,7折 32 return getPreRent()*days*0.7; 33 } 34 return 0; 35 } 36 }
3.定义客车类
扫描二维码关注公众号,回复:
7982176 查看本文章
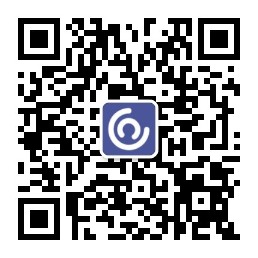
1 package cn.aura.demo01; 2 /** 3 * 客车类 4 */ 5 public class Bus extends Moto{ 6 //私有属性 7 private int seatCount;//座位数 8 //set和get方法 9 public int getSeatCount() { 10 return seatCount; 11 } 12 public void setSeatCount(int seatCount) { 13 this.seatCount = seatCount; 14 } 15 //构造方法 16 public Bus(String id, String brand, int seatCount, int preRent) { 17 super(id, brand, preRent); 18 this.seatCount = seatCount; 19 } 20 //重写计算租金的算法 21 @Override 22 public double calRent(int days) { 23 if (days >= 3 && days < 7) { 24 //days大于3天,9折 25 return getPreRent()*days*0.9; 26 }else if (days >= 7 && days <30) { 27 //days大于3天,8折 28 return getPreRent()*days*0.8; 29 }else if (days >= 30 && days <150) { 30 //days大于30天,7折 31 return getPreRent()*days*0.7; 32 }else if (days >= 150) { 33 //days大于150天,6折 34 return getPreRent()*days*0.6; 35 } 36 return 0; 37 } 38 }
4.定义汽车业务类
1 package cn.aura.demo01; 2 /** 3 * 定义汽车业务类 4 */ 5 public class MotoOperation { 6 //初始化数组 7 public static Moto[] motos = new Moto[8]; 8 //创建8个汽车对象 9 public void init() { 10 motos[0] = new Car("京NY28558", "宝马","X6", 800); 11 motos[1] = new Car("京CNY3284", "宝马","550i", 600); 12 motos[2] = new Car("京NT37465", "别克","林荫大道", 300); 13 motos[3] = new Car("京NT96968", "别克","GL8", 600); 14 motos[4] = new Bus("京6566754", "金杯", 16,800); 15 motos[5] = new Bus("京8696997", "金龙",16, 800); 16 motos[6] = new Bus("京9696996", "金杯", 34,1500); 17 motos[7] = new Bus("京8696998", "金龙",34, 1500); 18 } 19 //租赁汽车的方法 20 public static Moto motoLeaseOut(String brand,String type,int seat) { 21 Moto che = null; 22 //for循环遍历数组motos 23 for(int i=0;i<motos.length;i++){ 24 //判断Moto类的motos[i]的类型是否和Car一样 25 if(motos[i] instanceof Car){ 26 //Moto类的motos[i]向下转型变成子类Car 27 Car car = (Car)motos[i]; 28 if(car.getBrand().equals(brand) && car.getType().equals(type)){ 29 che=car; 30 break; 31 } 32 }else{ 33 //Moto类的motos[i]向下转型变成子类Bus 34 Bus bus = (Bus)motos[i]; 35 if(bus.getBrand().equals(brand) && bus.getSeatCount() == seat){ 36 che=bus; 37 break; 38 } 39 } 40 } 41 return che; 42 } 43 }
5.定义测试类
1 package cn.aura.demo01; 2 3 import java.util.Scanner; 4 5 /** 6 * 汽车租赁管理类 7 */ 8 public class TestRent { 9 //主函数 10 public static void main(String[] args) { 11 MotoOperation motoOperation = new MotoOperation(); 12 //初始化汽车数组 13 motoOperation.init(); 14 //提示信息 15 System.out.println("***********欢迎光临腾飞汽车租赁公司***********"); 16 System.out.println("1、轿车 2、客车"); 17 System.out.println("请输入你要租赁的汽车类型:"); 18 String brand = "";//品牌 19 String type = "";//型号 20 int seat = 0;//座位数 21 //获取用户输入 22 Scanner scanner = new Scanner(System.in); 23 int flag = scanner.nextInt(); 24 if (flag == 1) { 25 Car car =null; 26 System.out.println("请选择你要租赁的汽车品牌:1、别克 2、宝马"); 27 int flag1 = scanner.nextInt(); 28 if (flag1 == 1) { 29 System.out.println("请选择你要租赁的汽车类型:1、林荫大道 2、GL8"); 30 int flag2 = scanner.nextInt(); 31 brand = "别克"; 32 if (flag2 == 1) { 33 type = "林荫大道"; 34 }else if (flag2 == 2) { 35 type = "GL8"; 36 } 37 }else if (flag1 ==2) { 38 brand = "宝马"; 39 System.out.println("请选择你要租赁的汽车类型:1、X6 2、550i"); 40 int flag2 = scanner.nextInt(); 41 if (flag2 == 1) { 42 type = "X6"; 43 }else if (flag2 == 2) { 44 type = "550i"; 45 } 46 } 47 }else if (flag ==2) { 48 Bus bus = null; 49 System.out.println("请选择你要租赁的汽车品牌:1、金龙 2、金杯"); 50 int flag1 = scanner.nextInt(); 51 if (flag1 == 1) { 52 brand = "金龙"; 53 System.out.println("请选择你要租赁的汽车座位数:1、16座 2、34座"); 54 int flag2 = scanner.nextInt(); 55 if (flag2 == 1) { 56 seat = 16; 57 }else if (flag2 == 2) { 58 seat = 34; 59 } 60 }else if (flag1 ==2) { 61 brand = "金杯"; 62 System.out.println("请选择你要租赁的汽车座位数:1、16座 2、34座"); 63 int flag2 = scanner.nextInt(); 64 if (flag2 == 1) { 65 seat = 16; 66 }else if (flag2 == 2) { 67 seat = 34; 68 } 69 } 70 } 71 //租车 72 Moto che = MotoOperation.motoLeaseOut(brand,type,seat); 73 //获取用户租车天数 74 System.out.println("请输入您的租车天数:"); 75 int days = scanner.nextInt(); 76 //计算租车钱 77 double money = che.calRent(days); 78 System.out.println("分配给您的汽车牌号是:"+che.getId()); 79 System.out.println("您需要支付的租赁费用是:"+money+"元"); 80 } 81 82 83 }