接口测试数据框架结构说明
conf
--environment.properties中配置服务的IP、端口、版本等信息
src.main
--java.sutoTest.qa
----BaseTest.java接口测试的基类,继承AbstractTestNGSpringContextTests类,另外需要通过@ContextConfiguration引入applicationContext.xml、dubboSource/*.xml、dataSource/*.xml等
--resources
----config
------dataSource文件夹下放测试数据,文件为.xml文件
------dubboSource文件夹下放置测试接口的api、url、version等信息,一般每个服务一个.xml文件
--------dubbo-application-name.xml:dubbo接口测试的基础配置信息
--------car-service-dubbo-consumer.xml:car-service服务的接口信息
----applicationContext.xml:spring启动相关配置
src.test.java.interfaceAutoTest
--car_service每个服务一个文件夹,方便管理
----CompanyInfoApi每个api一个子文件夹,方便管理
------FindCompanyInfo.java每个接口一个测试类,里面包含若干个test
pom.xml:中需引入需要测试的jar包
开始第一个接口测试
1、pom中引入需要测试的jar包
<!--测试接口的jar包-->
<dependency>
<groupId>com.caocao.zhuanche</groupId>
<artifactId>car-service-api</artifactId>
<version>1.8.20.BETA1</version>
</dependency>
2、配置dubbo-application-name.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<!-- - Copyright 1999-2011 Alibaba Group. - - Licensed under the Apache License,
Version 2.0 (the "License"); - you may not use this file except in compliance
with the License. - You may obtain a copy of the License at - - http://www.apache.org/licenses/LICENSE-2.0
- - Unless required by applicable law or agreed to in writing, software -
distributed under the License is distributed on an "AS IS" BASIS, - WITHOUT
WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. - See the
License for the specific language governing permissions and - limitations
under the License. -->
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<dubbo:application name="auto-test"/>
<dubbo:consumer check="false" timeout="10000"/>
</beans>
3、配置car-service-dubbo-consumer.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd
http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd" default-lazy-init="true">
<dubbo:reference id="carRemoteService"
interface="com.caocao.carservice.api.service.CarRemoteService"
version="${car-service.dubbo.version}" timeout="6000" url="${car-service.dubbo.url}"/>
<dubbo:reference id="CompanyInfoRemoteService"
interface="com.caocao.carservice.api.service.CompanyInfoRemoteService"
version="${car-service.dubbo.version}" timeout="6000" url="${car-service.dubbo.url}"/>
<dubbo:reference id="CompanyInfoApi"
interface="com.caocao.carservice.api.service.CompanyInfoApi"
version="${car-service.dubbo.version}" timeout="6000" url="${car-service.dubbo.url}"/>
</beans>
4、environmentA.properties中配置url和version
car-service.dubbo.version=daily
car-service.dubbo.url=dubbo://116.62.44.31:31581
5、编写BaseTest.java接口测试的基类
package autoTest;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.testng.AbstractTestNGSpringContextTests;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import java.io.IOException;
@ContextConfiguration(locations = {
"classpath*:applicationContext.xml",
// "classpath*:/config/dataSource/*.xml",
"classpath*:/config/dubboSource/*.xml"
})
//其实基类的主要作用就是引入spring的配置文件
public abstract class BaseTest extends AbstractTestNGSpringContextTests {
@BeforeClass
public void beforeClass() throws IOException {
}
@AfterClass
public void afterClass() {
}
}
6、编写接口的测试类
package interfaceAutoTest.car_service.Test_CompanyInfoApi;
import autoTest.BaseTest;
import com.caocao.carservice.api.model.CompanyInfoDTO;
import com.caocao.carservice.api.model.Result;
import com.caocao.carservice.api.service.CompanyInfoApi;
import org.testng.Assert;
import org.testng.annotations.Test;
import javax.annotation.Resource;
import java.util.List;
public class FindCompanyInfo extends BaseTest {
//接口测试类找那个必须用@Resource注入api,否则会找不到接口而报错
@Resource
private CompanyInfoApi companyInfoApi;
//@Test是testng测试对测试case的标注
@Test(description = "根据公司类型和城市查询租赁商信息,正常用例:传入入参为公司类型平台")
public void Test_type1(){
Integer type = 1;
Result<List<CompanyInfoDTO>> rpcResult = companyInfoApi.findCompanyInfo(type,null);
Assert.assertEquals(rpcResult.getCode().toString(),"1");
List<CompanyInfoDTO> companyInfoDTOList = rpcResult.getData();
Assert.assertEquals(companyInfoDTOList.get(0).getType().toString(),"1");
}
@Test(description = "根据公司类型和城市查询租赁商信息,正常用例:传入入参为公司类型租赁商")
public void Test_type2(){
Integer type = 2;
Result<List<CompanyInfoDTO>> rpcResult = companyInfoApi.findCompanyInfo(type,null);
Assert.assertEquals(rpcResult.getCode().toString(),"0");
List<CompanyInfoDTO> companyInfoDTOList = rpcResult.getData();
Assert.assertEquals(companyInfoDTOList.get(0).getType().toString(),"2");
}
}
7、运行它
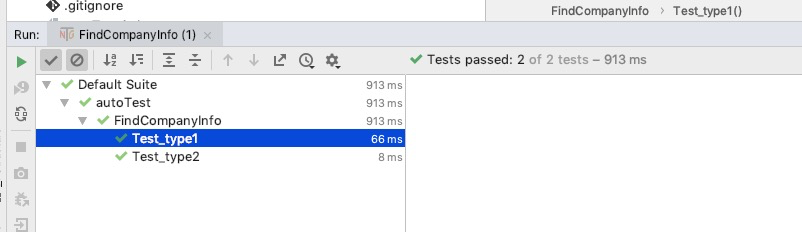
你会发现,测试结果为通过
然而你会发现打印台一个日志页没有,下一节我们来说说,如何显示接口调用的日志