文章出自https://www.cnblogs.com/MakeView660/p/6644838.html
前言
在VC++开发过程中,经常需要用到一些路径操作,比如拼需要的文件路径,搜索路径中的内容等等。Windows提供了一套关于路径操作的API帮助我们更好的执行这些操作。
路径截断与合并API
PathRemoveArgs 去除路径后面的参数
PathRemoveBackslash* 去除路径最后的反斜杠“\”
PathAddBackslash* 在路径最后加上反斜杠“\”
PathRemoveBlanks* 去除路径前后的空格
PathAddExtension* 在文件路径后面加上扩展名
PathRemoveExtension* 去除文件路径扩展名
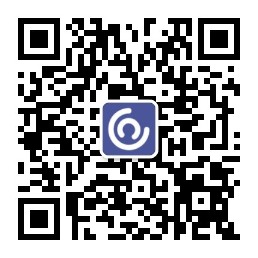
PathRenameExtension* 更改文件路径扩展名
PathRemoveFileSpec* 去除文件名,得到目录
PathUnquoteSpaces* 去除路径中的首尾开始的引号
PathQuoteSpaces 判断路径中是否有空格,有就是用双引号把整个路径包起来
PathAppend 将一个路径追加到另一个路径后面
PathCombine 合并两个路径
PathSkipRoot 去掉路径中的磁盘符或UNC部分
PathStripPath 去掉路径中的目录部分,得到文件名
PathStripToRoot 去掉路径的文件部分,得到根目录
PathCompactPath* 根据像素值生成符合长度的路径
如原始路径: C:\path1\path2\sample.txt
根据120像素截断后为: C:\pat...\sample.txt
根据25像素截断后为: ...\sample.txt
PathCompactPathEx* 根据字符个数来生成符合长度的路径
PathSetDlgItemPath 将路径数据设置到对话框的子控件上
PathUndecorate 去除路径中的修饰
PathUnExpandEnvStrings 将路径中部分数据替换为系统环境变量格式
路径查找比较API
PathFindOnPath 从路径中查找路径
PathFindExtension* 查找路径的扩展名
PathFindFileName* 获取路径的文件名
PathFindNextComponent 查找匹配路径,除去盘符之外
PathFindSuffixArray 查找给定的文件名是否有给定的后缀
PathGetArgs 获取路径参数
PathGetCharType 获取路径字符类型
PathGetDriveNumber 根据逻辑盘符返回驱动器序号
路径转换API
PathRelativePathTo 创建一个路径到另一个路径的相对路径。
PathResolve 将一个相对路径或绝对路径转换为一个合格的路径
PathCanonicalize 规范化路径,将格式比较乱的路径整理成规范的路径格式
PathBuildRoot 根据给定的磁盘序号创建根目录路径
CreateDirectory 创建目录
GetShortPathName* 将长路径转为短路径格式
GetLongPathName* 将短路径格式转为长路径
PathGetShortPath* 将长路径转为短路径格式(在后新版本Windows中不可用!)
PathCreateFromUrl 将URL路径转为MS-DOS格式
PathMakePretty* 把路径全部转为小写,增加可读性
PathMakeSystemFolder 给路径增加系统属性
PathUnmakeSystemFolder 去除路径中的系统属性
PathMakeUniqueName 从模板创建统一的路径格式
PathProcessCommand 生成一个可执行的路径这在ShellExecute中比较有用
路径验证API
PathCleanupSpec 去除路径中不合法的字符
PathCommonPrefix 比较并提取两个路径相同的前缀
PathFileExists 验证路径是否存在
PathMatchSpec 判断路径是否匹配制定的扩展名
PathIsDirectory 判断路径是否是一个有效的目录
PathIsFileSpec 验证路径是否一个文件名(有可能是一个路径)
PathIsExe 验证路径是否是可执行文件。
PathIsRoot 路径是否为根路径
PathIsRelative 判断路径是否是相对路径
PathIsContentType 检测文件是否为制定类型。
PathIsContentType(“hello.txt” , “text/plain”) 返回TRUE
PathIsContentType(“hello.txt” , “image/gif”) 返回FALSE
PathIsHTMLFile 判断路径是否是html文件类型——根据系统注册类型判断
PathIsLFNFileSpec 判断路径是否是长路径格式
PathIsNetworkPath 判断路径是否是一个网络路径。
PathIsPrefix 判断路径是否含有指定前缀
PathIsSameRoot 判断路径是否有相同根目录
PathIsSlow 判断路径是否是一个高度延迟的网络连接
PathIsSystemFolder 判断路径是否有系统属性(属性可以自己设定)
PathIsUNC 路径是否是UNC格式(网络路径)
PathIsUNCServer 路径是否是UNC服务器
PathIsUNCServerShare 路径是否仅仅是UNC的共享路径格式
PathIsURL 路径是否是http格式
PathYetAnotherMakeUniqueName 基于已存在的文件,自动创建一个唯一的文件名。比如存在“新建文件”,此函数会创建文件名“新建文件(2)”
1 #include <windows.h> 2 #include <iostream> 3 #include <Shlwapi.h> 4 #include <shlobj.h> 5 #pragma comment(lib, "shlwapi.lib"); 6 using namespace std; 7 8 void main( void ) 9 { 10 // PathRemoveArgs 11 char buffer_0[ ] = "c:\\a\\b\\FileA Arg1 Arg2"; 12 char *lpStr0; 13 lpStr0 = buffer_0; 14 15 cout << "Path before calling \"PathRemoveArgs\": " << lpStr0 << endl; 16 PathRemoveArgs(lpStr0); 17 cout << "Path before calling \"PathRemoveArgs\": " << lpStr0 << endl; 18 19 20 // PathRemoveBackslash 21 char buffer_1[ ] = "c:\\a\\b\\File\\"; 22 char *lpStr1; 23 lpStr1 = buffer_1; 24 25 cout << "Path before calling \"PathRemoveBackslash\": " << lpStr1 << endl; 26 PathRemoveBackslash(lpStr1); 27 cout << "Path after calling \"PathRemoveBackslash\": " << lpStr1 << endl; 28 29 // PathAddBackslash 30 char buffer_2[MAX_PATH] = "c:\\a\\b\\File"; 31 char *lpStr2; 32 lpStr2 = buffer_2; 33 34 cout << "Path before calling \"PathAddBackslash\": " << lpStr2 << endl; 35 PathAddBackslash(lpStr2); 36 cout << "Path after calling \"PathAddBackslash\": " << lpStr2 << endl; 37 38 // PathRemoveBlanks 39 char buffer_3[ ] = " c:\\TEST\\File1\\File2 "; 40 char *lpStr3; 41 lpStr3 = buffer_3; 42 43 cout << "Path before calling \"PathRemoveBlanks\": " << lpStr3 << endl; 44 PathRemoveBlanks(lpStr3); 45 cout << "Path after calling \"PathRemoveBlanks\": " << lpStr3 << endl; 46 47 // PathAddExtension 48 char buffer_4[MAX_PATH] = "file"; 49 char *lpStr4; 50 lpStr4 = buffer_4; 51 char *lpExt = ".txt"; 52 53 cout << "Path before calling \"PathAddExtension\": " << lpStr4 << endl; 54 PathAddExtension(lpStr4, lpExt); 55 cout << "Path after calling \"PathAddExtension\": " << lpStr4 << endl; 56 57 // PathRemoveExtension 58 char buffer_5[ ] = "C:\\TEST\\sample.txt"; 59 char *lpStr5; 60 lpStr5 = buffer_5; 61 62 cout << "Path before calling \"PathRemoveExtension\": " << lpStr5 << endl; 63 PathRemoveExtension(lpStr5); 64 cout << "Path after calling \"PathRemoveExtension\": " << lpStr5 << endl; 65 66 // PathRenameExtension 67 char buffer_6[ ] = "C:\\TEST\\sample.txt"; 68 char *lpStr6; 69 lpStr6 = buffer_6; 70 char *lpReExt = ".doc"; 71 72 cout << "Path before calling \"PathRenameExtension\": " << lpStr6 << endl; 73 PathRenameExtension(lpStr6, lpReExt); 74 cout << "Path after calling \"PathRenameExtension\": " << lpStr6 << endl; 75 76 // PathRemoveFileSpec 77 char buffer_7[ ] = "C:\\TEST\\sample.txt"; 78 char *lpStr7; 79 lpStr7 = buffer_7; 80 81 cout << "Path before calling \"PathRemoveFileSpec\": " << lpStr7 << endl; 82 PathRemoveFileSpec(lpStr7); 83 cout << "Path after calling \"PathRemoveFileSpec\": " << lpStr7 << endl; 84 85 // PathUnquoteSpaces 86 char buffer_8[ ] = "\"C:\\path1\\path2\""; 87 char *lpStr8; 88 lpStr8 = buffer_8; 89 90 cout << "Path before calling \"PathUnquoteSpaces\": " << lpStr8 << endl; 91 PathUnquoteSpaces(lpStr8); 92 cout << "Path after calling \"PathUnquoteSpaces\": " << lpStr8 << endl; 93 94 // PathQuoteSpaces 95 char buffer_9[MAX_PATH] = "C:\\sample_one\\sample two"; 96 char *lpStr9; 97 lpStr9 = buffer_9; 98 99 cout << "Path before calling \"PathQuoteSpaces \": " << lpStr9 << endl; 100 PathQuoteSpaces (lpStr9); 101 cout << "Path after calling \"PathQuoteSpaces \": " << lpStr9 << endl; 102 103 104 // PathAppend 105 /* 106 BOOL PathAppend( 107 LPTSTR pszPath, 108 LPCTSTR pszMore 109 ); 110 */ 111 112 // PathCombine 113 /* 114 LPTSTR PathCombine( 115 LPTSTR lpszDest, 116 LPCTSTR lpszDir, 117 LPCTSTR lpszFile 118 ); 119 */ 120 121 // PathStripPath 122 TCHAR szPath1[] = TEXT("c:\\dir1\\file.txt"); 123 cout << "Path before calling \"PathQuoteSpaces \": " << szPath1 << endl; 124 PathStripPath(szPath1); 125 cout << "Path after calling \"PathQuoteSpaces \": " << szPath1 << endl; 126 127 // PathCompactPath 128 char buffer_10[MAX_PATH] = "C:\\path1\\path2\\sample.txt"; 129 char *lpStr10; 130 lpStr10 = buffer_10; 131 HDC hdc = GetDC(NULL); 132 133 cout << "The un-truncated path is " << lpStr10 << endl; 134 PathCompactPath(hdc, lpStr10, 125); 135 cout << "The truncated path at 125 pixels is : " << lpStr10 << endl; 136 137 // PathCompactPathEx 138 char buffer_dst[MAX_PATH] = ""; 139 char *lpStrDst; 140 lpStrDst = buffer_dst; 141 142 char buffer_Org[MAX_PATH] = "C:\\path1\\path2\\sample.txt"; 143 char *lpStrOrg; 144 lpStrOrg = buffer_Org; 145 146 cout << "Path before calling \"PathCompactPathEx \": " << lpStrOrg << endl; 147 PathCompactPathEx(lpStrDst, lpStrOrg, 10, 0); 148 cout << "Path after calling \"PathCompactPathEx \": " << lpStrDst << endl; 149 150 151 // PathFindExtension 152 char buffer_Rev[MAX_PATH] = ""; 153 char *lpStrRev; 154 lpStrRev = buffer_Rev; 155 156 char buffer_11[] = "C:\\www.txt"; 157 char *lpStr11; 158 lpStr11 = buffer_11; 159 160 cout << "Path before calling \"PathFindExtension \": " << lpStr11 << endl; 161 lpStrRev = PathFindExtension(lpStr11); 162 cout << "Path after calling \"PathFindExtension \": " << lpStrRev << endl; 163 164 // PathFindFileName 165 cout << "Path before calling \"PathFindFileName \": " << lpStr11 << endl; 166 lpStrRev = PathFindFileName(lpStr11); 167 cout << "Path after calling \"PathFindFileName \": " << lpStrRev << endl; 168 169 // PathFindNextComponent 170 char buffer_12[ ] = "c:\\path1\\path2\\test"; 171 char *lpStr12; 172 lpStr12 = buffer_12; 173 174 cout << "Search a path for the next path component after the root " << lpStr1 << endl; 175 cout << "Return the next path component: \"" << PathFindNextComponent(lpStr1) << "\"" << endl; 176 177 // PathFindSuffixArray (case-sensitive ) 178 char* pszFilePath = "c:\\path1\\path2\\test.rtl"; 179 LPCTSTR FILE_EXT_NAME[] = {".mp3", ".doc", ".rtl", ".ogg"}; 180 const LPCTSTR* suffix_array = FILE_EXT_NAME; 181 int iArraySize = 4; 182 LPCTSTR lpStrRevStr = PathFindSuffixArray(pszFilePath, suffix_array, iArraySize); 183 184 cout << "Path before calling \"PathFindSuffixArray \": " << pszFilePath << endl; 185 cout << "Path after calling \"PathFindSuffixArray \": " << lpStrRevStr << endl; 186 187 // GetLongPathName 188 // GetShortPathName 189 // PathGetShortPath() It might be altered or unavailable in subsequent versions of Windows. 190 191 // PathMakePretty 192 char buffer_13[ ] = "C:\\TEST\\FILE"; 193 char *lpStr13; 194 lpStr13 = buffer_13; 195 196 char buffer_14[ ] = "c:\\test\\file"; 197 char *lpStr14; 198 lpStr14 = buffer_14; 199 200 cout << "The content of the unconverted path is : " << lpStr13 << endl; 201 cout << "The \"PathMakePretty\" function returns the value " 202 << PathMakePretty(lpStr13) << " = TRUE & converts" << endl; 203 cout << "The content of the converted path is : " << lpStr13 << endl; 204 205 cout << "The content of the unconverted path is : " << lpStr14 << endl; 206 cout << "The \"PathMakePretty\" function returns the value " 207 << PathMakePretty(lpStr4) << " = FALSE & no conversion" << endl; 208 cout << "The content of the converted path is : " << lpStr14 << endl; 209 210 211 // PathYetAnotherMakeUniqueName(Unicode String) 212 WCHAR* pszUniqueName = new WCHAR[MAX_PATH]; 213 memset(pszUniqueName, 0, MAX_PATH); 214 WCHAR* pszPath = L"C:\\"; 215 WCHAR* pszShort = NULL; 216 WCHAR* pszFileSpec = L"www.txt"; 217 218 wcout << "Path before calling \"PathYetAnotherMakeUniqueName \": " << pszUniqueName << endl; 219 BOOL bRet = PathYetAnotherMakeUniqueName(pszUniqueName, pszPath, pszShort, pszFileSpec); 220 wcout << "Path after calling \"PathYetAnotherMakeUniqueName \": " << pszUniqueName << endl; 221 222 if (pszUniqueName) 223 { 224 delete pszUniqueName; 225 pszUniqueName = NULL; 226 } 227 }
运行:
1 Path before calling "PathRemoveArgs": c:\a\b\FileA Arg1 Arg2 2 Path before calling "PathRemoveArgs": c:\a\b\FileA 3 Path before calling "PathRemoveBackslash": c:\a\b\File\ 4 Path after calling "PathRemoveBackslash": c:\a\b\File 5 Path before calling "PathAddBackslash": c:\a\b\File 6 Path after calling "PathAddBackslash": c:\a\b\File\ 7 Path before calling "PathRemoveBlanks": c:\TEST\File1\File2 8 Path after calling "PathRemoveBlanks": c:\TEST\File1\File2 9 Path before calling "PathAddExtension": file 10 Path after calling "PathAddExtension": file.txt 11 Path before calling "PathRemoveExtension": C:\TEST\sample.txt 12 Path after calling "PathRemoveExtension": C:\TEST\sample 13 Path before calling "PathRenameExtension": C:\TEST\sample.txt 14 Path after calling "PathRenameExtension": C:\TEST\sample.doc 15 Path before calling "PathRemoveFileSpec": C:\TEST\sample.txt 16 Path after calling "PathRemoveFileSpec": C:\TEST 17 Path before calling "PathUnquoteSpaces": "C:\path1\path2" 18 Path after calling "PathUnquoteSpaces": C:\path1\path2 19 Path before calling "PathQuoteSpaces ": C:\sample_one\sample two 20 Path after calling "PathQuoteSpaces ": "C:\sample_one\sample two" 21 Path before calling "PathQuoteSpaces ": c:\dir1\file.txt 22 Path after calling "PathQuoteSpaces ": file.txt 23 The un-truncated path is C:\path1\path2\sample.txt 24 The truncated path at 125 pixels is : C:\pa...\sample.txt 25 Path before calling "PathCompactPathEx ": C:\path1\path2\sample.txt 26 Path after calling "PathCompactPathEx ": ...\sa... 27 Path before calling "PathFindExtension ": C:\www.txt 28 Path after calling "PathFindExtension ": .txt 29 Path before calling "PathFindFileName ": C:\www.txt 30 Path after calling "PathFindFileName ": www.txt 31 Search a path for the next path component after the root c:\a\b\File 32 Return the next path component: "a\b\File" 33 Path before calling "PathFindSuffixArray ": c:\path1\path2\test.rtl 34 Path after calling "PathFindSuffixArray ": .rtl 35 The content of the unconverted path is : C:\TEST\FILE 36 The "PathMakePretty" function returns the value 1 = TRUE & converts 37 The content of the converted path is : C:\test\file 38 The content of the unconverted path is : c:\test\file 39 The "PathMakePretty" function returns the value 0 = FALSE & no conversion 40 The content of the converted path is : c:\test\file 41 Path before calling "PathYetAnotherMakeUniqueName ": 42 Path after calling "PathYetAnotherMakeUniqueName ": C:\www (2).txt 43 请按任意键继续. . .
希望大家能把自己的所学和他人一起分享,不要去鄙视别人索取时的贪婪,因为最应该被鄙视的是不肯分享时的吝啬。