示例
// 字符串类型string的用法
package main
import (
"fmt"
"unsafe"
)
func main() {
// 字符串类型 str1
var str1 string = "上海 北京 12345 西安"
fmt.Printf("str1 的值为 %s, %v, %q, 类型为 %T, 占 %d 个字节\n", str1, str1, str1, str1, unsafe.Sizeof(str1)) // str1 的值为 上海 北京 12345 西安, 上海 北京 12345 西安, "上海 北京 12345 西安", 类型为 string, 占 16 个字节
var str2, str3 string = "hello ", "world"
fmt.Printf("str2 = %q, %v, %s, str3 = %q, %v, %s\n", str2, str2, str2, str3, str3, str3) // str2 = "hello ", hello , hello , str3 = "world", world, world
// 错误:cannot assign to str2[1]
// go中的字符串是不可变的,一旦被赋值,不能再修改
// str2[1] = 'k'
// fmt.Printf("str2 = %v\n", str2)
// 双引号会识别转义字符
// 中文字符串
str4 := "你好\000北京\t上海济南武汉\007天津\x7f"
fmt.Printf("str4 = %v\n", str4) // str4 = 你好北京 上海济南武汉天津
fmt.Printf("str4 = %s\n", str4) // str4 = 你好北京 上海济南武汉天津
// %v和%s一起格式化字符串时,输出的结果不一样
fmt.Printf("str4 = %v, %s\n", str4, str4) // str4 = 你好北京 上海济南武汉天., 你好北京 上海济南武汉天津
// 英文字符串
str5 := "hello\000world\tgood\007yellow\x7f"
fmt.Printf("str5 = %v\n", str5) // str5 = helloworld goodyellow
fmt.Printf("str5 = %s\n", str5) // str5 = helloworld goodyellow
// %v和%s一起格式化字符串时,输出的结果不一样
fmt.Printf("str5 = %v, %s\n", str5, str5) // str5 = helloworld goodyello, helloworld goodyellow
// 反引号,以字符串的原生形式输出,包括换行和特殊字符
// 下面用反引号原样输出一段示例代码
var str6 = `
// bool布尔类型的用法
package main
import (
"fmt"
"unsafe"
)
func main() {
// bool类型
b1 := false
fmt.Printf("b1的值为 %t, 类型为 %T, 占 %d 个字节\n", b1, b1, unsafe.Sizeof(b1)) // b1的值为 false, 类型为 bool, 占 1 个字节
// b1 = 1 会报错: cannot use 1 (type int) as type bool in assignment
// 因为b1是bool类型,只能取值true或者false
// 不可以用0或者非0的整数替代false或者true,这点与C语言不同
// n1 := 1
// 错误:non-bool n1 (type int) used as if condition
// if n1 {
// fmt.Printf("n1 = %d\n", n1)
// }
// 错误:cannot use 0 (type int) as type bool in assignment
// var b2 bool = 0
// fmt.Printf("b2 = %t\n", b2)
}
`
fmt.Printf("\t示例代码: \n%v\n", str6)
// 以上代码会输出:
//
// 示例代码:
// bool布尔类型的用法
// package main
// import (
// "fmt"
// "unsafe"
// )
// func main() {
// // bool类型
// b1 := false
// fmt.Printf("b1的值为 %t, 类型为 %T, 占 %d 个字节\n", b1, b1, unsafe.Sizeof(b1)) // b1的值为 false, 类型为 bool, 占 1 个字节
// // b1 = 1 会报错: cannot use 1 (type int) as type bool in assignment
// // 因为b1是bool类型,只能取值true或者false
// // 不可以用0或者非0的整数替代false或者true,这点与C语言不同
// // n1 := 1
// // 错误:non-bool n1 (type int) used as if condition
// // if n1 {
// // fmt.Printf("n1 = %d\n", n1)
// // }
// // 错误:cannot use 0 (type int) as type bool in assignment
// // var b2 bool = 0
// // fmt.Printf("b2 = %t\n", b2)
// }
// 用加号 '+' 进行拼接
str7, str8, str9 := "你好", " 中国 ", "北京"
// 加号两边必须都是字符串才可以拼接
newStr1 := str7 + str8 + str9
fmt.Printf("拼接之后的新串为 %q, %v\n", newStr1, newStr1) // 拼接之后的新串为 "你好 中国 北京", 你好 中国 北京
// 字符串过长时用 '+' 进行分行,然后拼接
// 加号必须放在分行的最右边
var str10 string = "剑阁峥嵘而崔嵬,一夫当关,万夫莫开。" +
"所守或匪亲,化为狼与豺。朝避猛虎,夕避长蛇;磨牙吮血," +
"杀人如麻。锦城虽云乐,不如早还家。蜀道之难,难于上青天," +
"侧身西望长咨嗟!"
fmt.Printf("str10的内容为:%v\n", str10) // str10的内容为:剑阁峥嵘而崔嵬,一夫当关,万夫莫开。所守或匪亲,化为狼与豺。朝避猛虎,夕避长蛇;磨牙吮血,杀人如麻。锦城虽云乐,不如早还家。蜀道之难,难于上青天,侧身西望长咨嗟!
}
查看源代码
总结
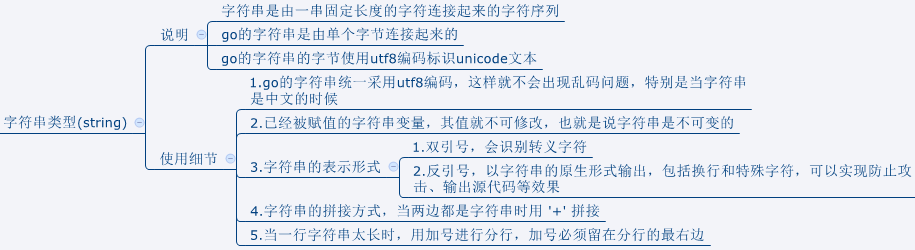