这个就是vue官网的例子,只是记录一下熟悉的过程:
一、绑定 HTML Class
(一)、对象语法
1 <template> 2 <div> 3 <div class="basic" v-bind:class="{ active: isActive,'text-danger': hasError}">样式测试</div> 4 <div class="basic" v-bind:class="classObject">样式测试</div> 5 </div> 6 </template> 7 <script> 8 export default { 9 data(){ 10 return { 11 isActive:true, 12 hasError:false, 13 error:true, 14 } 15 }, 16 computed:{ 17 classObject: function () { 18 return { 19 active: this.isActive, 20 'text-danger': this.error 21 } 22 } 23 } 24 } 25 </script> 26 <style scoped> 27 .basic{ 28 width:200px; 29 height:20px; 30 margin-bottom:10px; 31 } 32 .active{ 33 background-color: deepskyblue; 34 } 35 .text-danger{ 36 color: red; 37 } 38 </style>
(二)、数组语法
1 <template> 2 <div> 3 <div class="basic" v-bind:class="[activeClass, errorClass]">样式测试</div> 4 <div class="basic" v-bind:class="[isActive ? activeClass : '', errorClass]">样式测试</div> 5 <div class="basic" v-bind:class="[{ active: isAct }, errorClass]">样式测试</div> 6 </div> 7 </template> 8 <script> 9 export default { 10 data(){ 11 return { 12 activeClass:'active', 13 errorClass:'text-danger', 14 isActive:true,//为truthy值时 15 isAct:false,//假值 16 } 17 } 18 } 19 </script> 20 <style scoped> 21 .basic{ 22 width:200px; 23 height:20px; 24 margin-bottom:10px; 25 } 26 .active{ 27 background-color: deepskyblue; 28 } 29 .text-danger{ 30 color: red; 31 } 32 </style>
truthy值扩展:https://developer.mozilla.org/zh-CN/docs/Glossary/Truthy
二、绑定内联样式
(一)、对象语法
1 <template> 2 <div> 3 <div class="basic" v-bind:style="{ 'background-color': backColor, fontSize: fontSize + 'px',color: activeColor}">样式测试</div> 4 <div class="basic" v-bind:style="styleObject">样式测试</div> 5 </div> 6 </template> 7 <script> 8 export default { 9 data(){ 10 return { 11 backColor:'deepskyblue', 12 activeColor:'red', 13 fontSize:16 14 } 15 }, 16 computed:{ 17 styleObject() { 18 let style = {}; 19 if(this.backColor) { 20 style['background-color'] = this.backColor; 21 } 22 if(this.activeColor) { 23 style.color = this.activeColor; 24 } 25 if(this.fontSize) { 26 style['font-size'] = `${this.fontSize}px`; 27 } 28 return style; 29 } 30 } 31 32 } 33 </script> 34 <style scoped> 35 .basic{ 36 width:200px; 37 height:20px; 38 margin-bottom:10px; 39 } 40 </style>
扫描二维码关注公众号,回复:
7767400 查看本文章
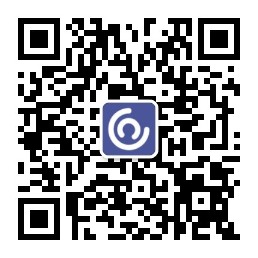
(二)、数组语法
1 <template> 2 <div> 3 <div class="basic" v-bind:style="[colorStyles, fontStyles]">样式测试</div> 4 </div> 5 </template> 6 <script> 7 export default { 8 data(){ 9 return { 10 colorStyles:{ 'background-color': 'deepskyblue',color: 'red'}, 11 fontWeight:'bold', 12 fontSize:15 13 } 14 }, 15 computed:{ 16 fontStyles() { 17 let style = {}; 18 if(this.fontSize) { 19 style['font-size'] = `${this.fontSize}px`; 20 } 21 if(this.fontWeight){ 22 style['font-weight'] = this.fontWeight; 23 } 24 return style; 25 } 26 } 27 } 28 </script> 29 <style scoped> 30 .basic{ 31 width:200px; 32 height:20px; 33 margin-bottom:10px; 34 } 35 </style>