package com.easecom.zhwg.ctrl.scene.wirelesindex; import java.io.InputStream; import java.io.UnsupportedEncodingException; import java.net.HttpURLConnection; import java.net.URL; import java.net.URLEncoder; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Calendar; import java.util.Collections; import java.util.HashMap; import java.util.HashSet; import java.util.Iterator; import java.util.List; import java.util.Map; import java.util.Set; import android.app.Activity; import android.app.AlertDialog; import android.app.AlertDialog.Builder; import android.app.Dialog; import android.app.ProgressDialog; import android.content.Context; import android.content.DialogInterface; import android.content.Intent; import android.graphics.Typeface; import android.os.AsyncTask; import android.os.Bundle; import android.os.Handler; import android.os.Message; import android.util.Log; import android.view.GestureDetector; import android.view.GestureDetector.OnGestureListener; import android.view.Gravity; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuInflater; import android.view.MenuItem; import android.view.MotionEvent; import android.view.View; import android.view.View.OnTouchListener; import android.view.ViewGroup; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.Button; import android.widget.EditText; import android.widget.LinearLayout; import android.widget.ListView; import android.widget.SimpleAdapter; import android.widget.TextView; import android.widget.Toast; import com.easecom.widget.OnWheelChangedListener; import com.easecom.widget.OnWheelScrollListener; import com.easecom.widget.WheelView; import com.easecom.widget.adapters.ArrayWheelAdapter; import com.easecom.widget.adapters.NumericWheelAdapter; import com.easecom.zhwg.R; import com.easecom.zhwg.app.ApplicationStack; import com.easecom.zhwg.app.WGApp; import com.easecom.zhwg.ctrl.scene.station.ListViewDialogAdapter; import com.easecom.zhwg.util.StrUtils; import com.easecom.zhwg.util.URLUtil; public class TwoGWirelessindex extends Activity implements OnTouchListener, OnGestureListener { private Button top_back; private TextView top_text; private Button mButton_right; private Button mButton_left; private TextView mText_tilte; // 显示数据列表 private ListView listView; private SimpleAdapter mInfo;; private List<Map<String, String>> n_list; // 判断显示第几个 private int number = 0; // 判断有多少数据 private int data_num = 0; private String mStartTime = ""; // 判断是否为选择时间查询 private boolean isSelectDate = false; // 存放获取的数据 private List<TwoGWirelesDao> mTwoGWirelesDao; // 存放上一次的数据(避免 读取网络数据为空时给mTwoGWirelesDao赋空值) private static List<TwoGWirelesDao> mTwoGWirelesDaoOld=null; private WGApp app; private StringBuffer url; private ProgressDialog progressDialog; //日期控件使用 private int startYear; private int startMonth; private int startDay; // private int startHour; // private int startMinute; private int endYear; private int endMonth; private int endDay; // private int endHour; // private int endMinute; private ApplicationStack applicationStack; // private String start_time = ""; // private String end_time = ""; //标题触控事件 private LinearLayout report_wangyou_line; GestureDetector mGestureDetector; private static final int FLING_MIN_DISTANCE = 50; private static final int FLING_MIN_VELOCITY = 0; private LayoutInflater mInflater; String start_time = ""; String end_time = ""; boolean[] selected_zb = new boolean[]{false,false,false,false,false,false,false,false,false,false,false,false,false,false,false}; boolean[] selected_cellname ; boolean[] selected_bsc ; private static ArrayList cellnameList=null; private static ArrayList bscList=null; /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.report_wireless2g_list); mGestureDetector = new GestureDetector(this); mTwoGWirelesDao = new ArrayList<TwoGWirelesDao>(); app=(WGApp)this.getApplication();; top_back = (Button) findViewById(R.id.detail_top_back); top_back.setVisibility(View.VISIBLE); top_back.setOnClickListener(listener); top_text = (TextView) findViewById(R.id.detail_top_text); top_text.setText(""); mText_tilte = (TextView) findViewById(R.id.report_wangyou_title); mText_tilte.setText("小区ID"); listView = (ListView) findViewById(R.id.wangyou_list); //listView.setOnTouchListener(this); //新的触控事件 report_wangyou_line = (LinearLayout)findViewById(R.id.report_wangyou_line); report_wangyou_line.setOnTouchListener(this); mButton_left = (Button) findViewById(R.id.report_wangyou_btleft); mButton_left.setOnClickListener(listener); mButton_left.setBackgroundResource(R.drawable.body_leftgray); mButton_right = (Button) findViewById(R.id.report_wangyou_btright); mButton_right.setOnClickListener(listener); progressDialog = ProgressDialog.show(TwoGWirelessindex.this, null, "正在获取数据,请稍后...", true, true); mInflater = (LayoutInflater) this.getSystemService(LAYOUT_INFLATER_SERVICE); Calendar c = Calendar.getInstance(); c.add(Calendar.DAY_OF_MONTH, -1); startYear = c.get(Calendar.YEAR); startMonth = c.get(Calendar.MONTH); startDay = c.get(Calendar.DAY_OF_MONTH); endYear = c.get(Calendar.YEAR); endMonth = c.get(Calendar.MONTH); endDay = c.get(Calendar.DAY_OF_MONTH); SimpleDateFormat date_format = new SimpleDateFormat("yyyy-MM-dd"); String mDateTime = date_format.format(c.getTime()); String mTime = mDateTime.substring(0, 10); start_time = mTime; end_time = mTime; url = new StringBuffer(); url.append(URLUtil.WIRELES_TWOG); url.append("?citys=&startTime=&endTime=&baseStations=&bscNames="); initUrl(); } private void initUrl(){ new Thread(new Runnable() { @Override public void run() { Message msg = new Message(); msg.what = 1; handler.sendMessage(msg); } }).start(); } // 获取服务器数据 /* private List<TwoGWirelesDao> getData(String string) { List<TwoGWirelesDao> TwoGWirelesDaos = null; try { URL url = new URL(string); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("GET"); conn.setConnectTimeout(5 * 1000); conn.setReadTimeout(5 * 1000); InputStream inStream = conn.getInputStream(); TwoGWirelesDaos = new PullNPOReport().getNPOReportList(inStream); inStream.close(); conn.disconnect(); data_num = TwoGWirelesDaos.size(); Log.i("size()===", "" + TwoGWirelesDaos.size()); } catch (Exception e) { e.printStackTrace(); } return TwoGWirelesDaos; }*/ // 事件响应 private View.OnClickListener listener = new View.OnClickListener() { @Override public void onClick(View v) { switch (v.getId()) { case R.id.detail_top_back: TwoGWirelessindex.this.finish(); break; case R.id.report_wangyou_btleft: if (number > 0) { number = number - 1; mButton_right.setBackgroundResource(R.drawable.button_right); } if (number == 0) { mButton_left.setBackgroundResource(R.drawable.body_leftgray); } switch (number) { case 0: mText_tilte.setText("小区ID"); break; case 15: mText_tilte.setText("切换成功率"); break; } getListInfo(); mInfo.notifyDataSetChanged(); break; case R.id.report_wangyou_btright: if (number < 15) { number = number + 1; mButton_left.setBackgroundResource(R.drawable.button_left); } if (number == 15) { mButton_right.setBackgroundResource(R.drawable.body_rightgray); } switch (number) { case 0: mText_tilte.se break; case 15: mText_tilte.setT break; } getListInfo(); mInfo.notifyDataSetChanged(); break; } } }; // 菜单选项 public boolean onCreateOptionsMenu(Menu menu) { super.onCreateOptionsMenu(menu); MenuInflater inflater = getMenuInflater(); inflater.inflate(R.menu.querry_date, menu); return true; } // 菜单按钮事件 public final boolean onOptionsItemSelected(MenuItem item) { boolean result = false; switch (item.getItemId()) { case R.id.day_menu_cout: result = true; isSelectDate = true; new MyDialogSearch(TwoGWirelessindex.this).setDisplay(); break; default: result = false; break; } return result; } // 加载listview public void getListInfo() { listView.setOnItemClickListener(new OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { /* * Intent intent = new Intent(SearchJobResultActivity.this, * PositionActivity.class); intent.putExtra("Job_number", * positions.get(position) .getJob_number()); * Log.e("Job_number", "Job_number1 = " + * positions.get(position) .getJob_number()); * startActivity(intent); */ Bundle bundle = new Bundle(); bundle.putSerializable("g2_detail", mTwoGWirelesDao.get(position)); Intent intent = new Intent(TwoGWirelessindex.this, TwoGWirelessDetail.class); intent.putExtras(bundle); TwoGWirelessindex.this.startActivity(intent); } }); getDaoData(); mInfo = new SimpleAdapter(this, n_list, R.layout.report_wireless2g_list_row, new String[] { "n_date", "n_location", "n_title" }, new int[] { R.id.wangyou_item_date, R.id.wangyou_item_location, R.id.wangyou_item_title }); listView.setAdapter(mInfo); } /* * // 获取listview数据 public void getListData() { TwoGWirelesDao dao1 = new * TwoGWirelesDao(); for (int i = 0; i < 10; i++) { dao1.setmDate("2012-03-20"); * dao1.setmLocation("济南"); dao1.setInter_ho_rate("97.7%"); * dao1.setTch_call_rate("93.4%"); dao1.setOutcs_rate("96.7%"); * dao1.setChangesys_rate("92.1%"); dao1.setGsm_wxsys_rate("99.4%"); * dao1.setWcdma_wxsys_rate("93.8%"); mTwoGWirelesDao.add(dao1); } * * } */ // 存放dao数据 public void getDaoData() { /* 注意data_num的值 */ n_list = new ArrayList<Map<String, String>>(data_num); for (int i = 0; i < mTwoGWirelesDao.size(); i++) { Map<String, String> map = new HashMap<String, String>(); TwoGWirelesDao twogwirelesdao = mTwoGWirelesDao.get(i); if (isSelectDate) { if (mStartTime.equals(twogwirelesdao.getTongji_time())) { map.put("n_date", twogwirelesdao.getTongji_time()); map.put("n_location", ToDBC(twogwirelesdao.getCell_name())); switch (number) { case 0: map.put("n_title", twogwirelesdao.getCell_id() + ""); break; case 1: map.put("n_title", ToDBC(twogwirelesdao.getRelated_bts()) + ""); break; case 15: map.put("n_title", StrUtils.float2string(StrUtils.null2Zero(twogwirelesdao.getSwitchrate())) + "%"); break; } } } else { map.put("n_date", twogwirelesdao.getTongji_time()); map.put("n_location", ToDBC(twogwirelesdao.getCell_name())); switch (number) { case 0: map.put("n_title", twogwirelesdao.getCell_id() + ""); break; case 1: map.put("n_title", ToDBC(twogwirelesdao.getRelated_bts()) + ""); break; case 15: map.put("n_title", StrUtils.float2string(StrUtils.null2Zero(twogwirelesdao.getSwitchrate())) + "%"); break; } } n_list.add(map); } } /** * 半角转换为全角 * * @param input * @return */ public static String ToDBC(String input) { char[] c = input.toCharArray(); for (int i = 0; i < c.length; i++) { if (c[i] == 12288) { c[i] = (char) 32; continue; } if (c[i] > 65280 && c[i] < 65375) c[i] = (char) (c[i] - 65248); } return new String(c); } private Handler handler = new Handler() { @Override public void handleMessage(Message msg) { switch (msg.what) { case 0: if (null != progressDialog) progressDialog.dismiss(); break; case 1: new QueryWYTask().execute(url.toString()); break; case 2: break; case 3: break; } super.handleMessage(msg); } }; class QueryWYTask extends AsyncTask<String, Void, List<TwoGWirelesDao>> { @Override protected void onPostExecute(final List<TwoGWirelesDao> wyReports) { super.onPostExecute(wyReports); progressDialog.dismiss(); if (null == wyReports) { Toast toast = Toast.makeText(getApplicationContext(), "暂无信息!",Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER, 0, 0); toast.show(); return; } mTwoGWirelesDao = (List<TwoGWirelesDao>) wyReports; cellnameList=new ArrayList(); bscList=new ArrayList(); for (TwoGWirelesDao twogwirelesdao : mTwoGWirelesDao) { cellnameList.add(twogwirelesdao.getCell_name()); bscList.add(twogwirelesdao.getRelated_bsc()); } // Collections.sort(mTwoGWirelesDao); if ((mTwoGWirelesDao != null) && (mTwoGWirelesDao.size() > 0)) { mTwoGWirelesDaoOld=mTwoGWirelesDao; getListInfo(); }else{ mTwoGWirelesDao=mTwoGWirelesDaoOld; Toast toast = Toast.makeText(getApplicationContext(), "暂无数据!", Toast.LENGTH_SHORT); toast.setGravity(Gravity.CENTER, 0, 0); toast.show(); } Message msg1 = new Message(); msg1.what = 0; handler.sendMessage(msg1); } @Override protected List<TwoGWirelesDao> doInBackground(String... params) { // System.out.println("doInBackground in"); List<TwoGWirelesDao> otherposition = null; try { URL url = new URL(params[0]); HttpURLConnection conn = (HttpURLConnection) url.openConnection(); conn.setRequestMethod("GET"); conn.setConnectTimeout(URLUtil.CONNECT_TIME * 1000); conn.setReadTimeout(URLUtil.CONNECT_TIME * 1000); conn.connect(); InputStream inStream =conn.getInputStream(); otherposition = new TwoGWirelesService().getTwoGWirele(inStream); inStream.close(); } catch (Exception e) { e.printStackTrace(); } return otherposition; } } class MyDialogSearch extends Dialog implements View.OnClickListener { private Button button1_date; private Button button2_date; private Button button_cellname; private Button button_zhibiao; private EditText start_time; private EditText end_time; private Button cancelButton;// 取消按钮 private Button okButton;// 确定按钮 private EditText mSpinner_cellname;// cellname查询 private EditText mSpinner_parameter;// 指标 private ListViewDialogAdapter mAdapter; private Context context; private String citysString=""; //CellName参数 private String bscNamesString=""; //bscName参数 public MyDialogSearch(Context context) { super(context); this.context=context; } public void setDisplay() { setContentView(R.layout.report_wireless2g_dialog);// 设置对话框的布局 button1_date = (Button) findViewById(R.id.button1_date);// 开始日期按钮 button2_date = (Button) findViewById(R.id.button2_date);// 结束日期按钮 button_cellname=(Button)findViewById(R.id.button_cellname);//CellName按钮 button_zhibiao=(Button)findViewById(R.id.button_zhibiao);//指标按钮 start_time = (EditText) findViewById(R.id.start_time);// 开始时间文本框 end_time = (EditText) findViewById(R.id.end_time);// 结束时间文本框 cancelButton = (Button) findViewById(R.id.no_query);// 取消按钮 okButton = (Button) findViewById(R.id.yes_query);// 确定按钮 mSpinner_cellname = (EditText) findViewById(R.id.spinner_cellname);// cellname查询 mSpinner_parameter = (EditText) findViewById(R.id.spinner_zhibiao);// 指标 button1_date.setOnClickListener(listener); button2_date.setOnClickListener(listener); button_zhibiao.setOnClickListener(listener); button_cellname.setOnClickListener(listener); okButton.setOnClickListener(listener); cancelButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { dismiss(); } }); setTitle("查询条件");// 设定对话框的标题 start_time.setText(new StringBuilder().append(startYear) .append("-").append(format(startMonth + 1)).append("-") .append(format(startDay)) .append(" ").append("00:00:00")); end_time.setText(new StringBuilder().append(endYear) .append("-").append(format(endMonth + 1)).append("-") .append(format(endDay)) .append(" ").append("23:59:59")); show();// 显示对话框 } private View.OnClickListener listener = new View.OnClickListener() { @Override public void onClick(View v) { Dialog dialog = null; Builder builder = new AlertDialog.Builder(TwoGWirelessindex.this); switch (v.getId()) { case R.id.button1_date: initstarttime(start_time,1); break; case R.id.button2_date: initstarttime(end_time,2); break; case R.id.button_cellname: mSpinner_cellname.setText(""); citysString=""; Set someSet = new HashSet(cellnameList); Iterator iterator = someSet.iterator(); ArrayList tempList = new ArrayList(); while(iterator.hasNext()){ tempList.add(iterator.next().toString()); } Collections.sort(tempList); //给List排序 cellnameList=tempList; //去掉重复的重新付给cellnameList String[] items = new String[cellnameList.size()]; selected_cellname = new boolean[cellnameList.size()]; for(int i=0;i<selected_cellname.length;i++){ selected_cellname[i]=false; items[i]=(String) cellnameList.get(i); } builder.setTitle("选择指标"); DialogInterface.OnMultiChoiceClickListener mutiListener1 = new DialogInterface.OnMultiChoiceClickListener() { @Override public void onClick(DialogInterface dialogInterface, int which, boolean isChecked) { selected_cellname[which] = isChecked; } }; // builder.setMultiChoiceItems(R.array.user_name, selected_bsc, mutiListener2); //以前的例子 builder.setMultiChoiceItems(items, selected_cellname, mutiListener1); DialogInterface.OnClickListener btnListener1 = new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int which) { StringBuffer selectedStr = new StringBuffer(); StringBuffer selectedValue=new StringBuffer(); for(int i=0; i<selected_cellname.length; i++) { if(selected_cellname[i] == true) { selectedStr.append((String)cellnameList.get(i)); selectedStr.append(","); selectedValue.append((String)cellnameList.get(i)); selectedValue.append(","); /*selectedStr.append(getResources().getStringArray(R.array.user_name)[i]); selectedStr.append(","); selectedValue.append(getResources().getStringArray(R.array.int_value)[i]); selectedValue.append(",");*/ } } System.out.println("selectedStr:"+selectedStr); System.out.println("selectedValue:"+selectedValue); if(!(selectedStr==null||selectedStr.toString().equals(""))){ mSpinner_cellname.setText(selectedStr.substring(0, selectedStr.length()-1)); citysString=selectedValue.substring(0, selectedValue.length()-1); } } }; builder.setPositiveButton("确定", btnListener1); dialog = builder.create(); dialog.show(); break; case R.id.button_zhibiao: mSpinner_parameter.setText(""); bscNamesString=""; Set someSet1 = new HashSet(bscList); Iterator iterator1 = someSet1.iterator(); ArrayList tempList1 = new ArrayList(); while(iterator1.hasNext()){ tempList1.add(iterator1.next().toString()); } Collections.sort(tempList1); //给List排序 bscList=tempList1; //去掉重复的重新付给bscList String[] items1 = new String[bscList.size()]; selected_bsc = new boolean[bscList.size()]; for(int i=0;i<selected_bsc.length;i++){ selected_bsc[i]=false; items1[i]=(String) bscList.get(i); } builder.setTitle("选择指标"); DialogInterface.OnMultiChoiceClickListener mutiListener2 = new DialogInterface.OnMultiChoiceClickListener() { @Override public void onClick(DialogInterface dialogInterface, int which, boolean isChecked) { selected_bsc[which] = isChecked; } }; // builder.setMultiChoiceItems(R.array.user_name, selected_bsc, mutiListener2); //以前的例子 builder.setMultiChoiceItems(items1, selected_bsc, mutiListener2); DialogInterface.OnClickListener btnListener2 = new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int which) { StringBuffer selectedStr = new StringBuffer(); StringBuffer selectedValue=new StringBuffer(); for(int i=0; i<selected_bsc.length; i++) { if(selected_bsc[i] == true) { selectedStr.append((String)bscList.get(i)); selectedStr.append(","); selectedValue.append((String)bscList.get(i)); selectedValue.append(","); /*selectedStr.append(getResources().getStringArray(R.array.user_name)[i]); selectedStr.append(","); selectedValue.append(getResources().getStringArray(R.array.int_value)[i]); selectedValue.append(",");*/ } } System.out.println("selectedStr:"+selectedStr); System.out.println("selectedValue:"+selectedValue); if(!(selectedStr==null||selectedStr.toString().equals(""))){ mSpinner_parameter.setText(selectedStr.substring(0, selectedStr.length()-1)); bscNamesString=selectedValue.substring(0, selectedValue.length()-1); } } }; builder.setPositiveButton("确定", btnListener2); dialog = builder.create(); dialog.show(); break; case R.id.yes_query: dismiss(); progressDialog = ProgressDialog.show(TwoGWirelessindex.this, "请等待...", "正在加载信息,请稍后...",true, true); String path2 = ""; try { path2 = URLUtil.WIRELES_TWOG +"?citys="+ citysString +"&startTime="+ URLEncoder.encode(start_time.getText().toString(),"utf-8") + "&endTime="+ URLEncoder.encode(end_time.getText().toString(),"utf-8") +"&baseStations="+"&bscNames="+ bscNamesString; System.out.println("path2:::::"+path2); } catch (UnsupportedEncodingException e) { // TODO Auto-generated catch block e.printStackTrace(); } url = new StringBuffer(); url.append(path2); initUrl(); mInfo.notifyDataSetChanged(); mStartTime = ""; isSelectDate = false; break; default: break; } } }; private String format(int x) { String s = "" + x; if (s.length() == 1) s = "0" + s; return s; } @Override public void onClick(View v) { } } private String format(int x) { String s = "" + x; if (s.length() == 1) s = "0" + s; return s; } public boolean onTouch(View v, MotionEvent event) { // TODO Auto-generated method stub Log.i("touch", "touch"); return mGestureDetector.onTouchEvent(event); } @Override public boolean onDown(MotionEvent e) { // TODO Auto-generated method stub return true; } @Override public boolean onFling(MotionEvent e1, MotionEvent e2, float velocityX, float velocityY) { Log.e("TAG", "touch "); // TODO Auto-generated method stub if (e1.getX() - e2.getX() > FLING_MIN_DISTANCE && Math.abs(velocityX) > FLING_MIN_VELOCITY) { Log.e("TAG", "touch right"); if (number < 15) { number = number + 1; mButton_left.setBackgroundResource(R.drawable.button_left); } if (number == 15) { mButton_right.setBackgroundResource(R.drawable.body_rightgray); } switch (number) { case 0: mText_tilte.setText("小区ID"); break; case 15: mText_tilte.setText("切换成功率"); break; } getListInfo(); mInfo.notifyDataSetChanged(); } else if (e2.getX() - e1.getX() > FLING_MIN_DISTANCE && Math.abs(velocityX) > FLING_MIN_VELOCITY) { // Fling right Log.e("TAG", "touch left"); // Fling left if (number > 0) { number = number - 1; mButton_right.setBackgroundResource(R.drawable.button_right); } if (number == 0) { mButton_left.setBackgroundResource(R.drawable.body_leftgray); } switch (number) { case 0: mText_tilte.setText("小区ID"); break; case 15: mText_tilte.setText("切换成功率"); break; } getListInfo(); mInfo.notifyDataSetChanged(); } return false; } @Override public void onLongPress(MotionEvent e) { // TODO Auto-generated method stub } @Override public boolean onScroll(MotionEvent e1, MotionEvent e2, float distanceX, float distanceY) { // TODO Auto-generated method stub return false; } @Override public void onShowPress(MotionEvent e) { // TODO Auto-generated method stub } @Override public boolean onSingleTapUp(MotionEvent e) { // TODO Auto-generated method stub return false; } /** * Updates day wheel. Sets max days according to selected month and year */ void updateDays(WheelView year, WheelView month, WheelView day) { Calendar calendar = Calendar.getInstance(); calendar.set(Calendar.YEAR, calendar.get(Calendar.YEAR) + year.getCurrentItem()); calendar.set(Calendar.MONTH, month.getCurrentItem()); int maxDays = calendar.getActualMaximum(Calendar.DAY_OF_MONTH); day.setViewAdapter(new DateNumericAdapter(this, 1, maxDays, calendar .get(Calendar.DAY_OF_MONTH) - 1)); int curDay = Math.min(maxDays, day.getCurrentItem() + 1); day.setCurrentItem(curDay - 1, true); } /** * Adapter for numeric wheels. Highlights the current value. */ private class DateNumericAdapter extends NumericWheelAdapter { // Index of current item int currentItem; // Index of item to be highlighted int currentValue; /** * Constructor */ public DateNumericAdapter(Context context, int minValue, int maxValue, int current) { super(context, minValue, maxValue); this.currentValue = current; setTextSize(16); } public DateNumericAdapter(Context context, int minValue, int maxValue, int current, String format) { super(context, minValue, maxValue, format); this.currentValue = current; setTextSize(16); } @Override protected void configureTextView(TextView view) { super.configureTextView(view); if (currentItem == currentValue) { view.setTextColor(0xFF0000F0); } view.setTypeface(Typeface.SANS_SERIF); } @Override public View getItem(int index, View cachedView, ViewGroup parent) { currentItem = index; return super.getItem(index, cachedView, parent); } } /** * Adapter for string based wheel. Highlights the current value. */ private class DateArrayAdapter extends ArrayWheelAdapter<String> { // Index of current item int currentItem; // Index of item to be highlighted int currentValue; /** * Constructor */ public DateArrayAdapter(Context context, String[] items, int current) { super(context, items); this.currentValue = current; setTextSize(16); } @Override protected void configureTextView(TextView view) { super.configureTextView(view); if (currentItem == currentValue) { view.setTextColor(0xFF0000F0); } view.setTypeface(Typeface.SANS_SERIF); } @Override public View getItem(int index, View cachedView, ViewGroup parent) { currentItem = index; return super.getItem(index, cachedView, parent); } } /** * 时间控件 * */ private void initstarttime(final TextView sTextView,final int flag) { LayoutInflater inflater=LayoutInflater.from(this); final View dialog=inflater.inflate(R.layout.date_layout_dialog, null); final Calendar calendar = Calendar.getInstance(); final WheelView day = (WheelView) dialog.findViewById(R.id.day); final WheelView month = (WheelView) dialog.findViewById(R.id.month); final WheelView year = (WheelView) dialog.findViewById(R.id.year); final WheelView hours = (WheelView) dialog.findViewById(R.id.hour); final WheelView mins = (WheelView) dialog.findViewById(R.id.mins); final WheelView seconds = (WheelView) dialog.findViewById(R.id.seconds); AlertDialog.Builder builder=new AlertDialog.Builder(TwoGWirelessindex.this); builder.setTitle("选择时间"); builder.setView(dialog); //关键 builder.setPositiveButton("确定", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { sTextView.setText(calendar.get(Calendar.YEAR) + year.getCurrentItem()+"-" +((month.getCurrentItem()+1<10)?("0"+(month.getCurrentItem()+1)):month.getCurrentItem()+1)+"-" +(day.getCurrentItem() + 1) +" "+hours.getCurrentItem()+":" +((mins.getCurrentItem() < 10) ? ("0" + mins.getCurrentItem()) : mins.getCurrentItem())+":" +((seconds.getCurrentItem() < 10) ? ("0" + seconds.getCurrentItem()) : seconds.getCurrentItem())); if(flag==1){ start_time=calendar.get(Calendar.YEAR) + year.getCurrentItem()+"-" +((month.getCurrentItem()+1<10)?("0"+(month.getCurrentItem()+1)):month.getCurrentItem()+1)+"-" +(day.getCurrentItem() + 1) +" "+hours.getCurrentItem()+":" +((mins.getCurrentItem() < 10) ? ("0" + mins.getCurrentItem()) : mins.getCurrentItem())+":" +((seconds.getCurrentItem() < 10) ? ("0" + seconds.getCurrentItem()) : seconds.getCurrentItem()); }else if (flag==2) { end_time=calendar.get(Calendar.YEAR) + year.getCurrentItem()+"-" +((month.getCurrentItem()+1<10)?("0"+(month.getCurrentItem()+1)):month.getCurrentItem()+1)+"-" +(day.getCurrentItem() + 1) +" "+hours.getCurrentItem()+":" +((mins.getCurrentItem() < 10) ? ("0" + mins.getCurrentItem()) : mins.getCurrentItem())+":" +((seconds.getCurrentItem() < 10) ? ("0" + seconds.getCurrentItem()) : seconds.getCurrentItem()); } } }); builder.setNegativeButton("取消",new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialog, int which) { dialog.dismiss(); } }); OnWheelChangedListener listener = new OnWheelChangedListener() { public void onChanged(WheelView wheel, int oldValue, int newValue) { updateDays(year, month, day); } }; OnWheelScrollListener scrollListener = new OnWheelScrollListener() { public void onScrollingStarted(WheelView wheel) {} public void onScrollingFinished(WheelView wheel) {} }; // year int curYear = calendar.get(Calendar.YEAR); year.setViewAdapter(new DateNumericAdapter(this, curYear, curYear + 10,0)); year.setCurrentItem(curYear); year.addChangingListener(listener); year.addScrollingListener(scrollListener); // month int curMonth = calendar.get(Calendar.MONTH); String months[] = new String[] { "1月", "2月", "3月", "4月", "5月", "6月","7月", "8月", "9月", "10月", "11月", "12月" }; month.setViewAdapter(new DateArrayAdapter(this, months, curMonth)); month.setCurrentItem(curMonth); month.addChangingListener(listener); month.addScrollingListener(scrollListener); // day updateDays(year, month, day); day.setCurrentItem(calendar.get(Calendar.DAY_OF_MONTH) - 1); day.addScrollingListener(scrollListener); // hour int curHours = calendar.get(Calendar.HOUR_OF_DAY); hours.setViewAdapter(new DateNumericAdapter(this, 0, 23, curHours)); hours.setCurrentItem(curHours); hours.addScrollingListener(scrollListener); // minus int curMinutes = calendar.get(Calendar.MINUTE); mins.setViewAdapter(new DateNumericAdapter(this, 0, 59, curMinutes,"%02d")); mins.setCyclic(true); mins.setCurrentItem(curMinutes); mins.addScrollingListener(scrollListener); //second int curSeconds=calendar.get(Calendar.SECOND); seconds.setViewAdapter(new DateNumericAdapter(this, 0, 59, curSeconds,"%02d")); seconds.setCurrentItem(curSeconds); seconds.setCyclic(true); seconds.addScrollingListener(scrollListener); builder.create().show(); } }
report_wireless2g_list_row
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/list_mid_background" android:gravity="center_vertical" android:orientation="horizontal" > <!-- 时间 --> <!-- <TextView --> <!-- android:id="@+id/wangyou_item_date" --> <!-- android:layout_width="wrap_content" --> <!-- android:layout_height="wrap_content" --> <!-- android:gravity="left" --> <!-- android:text="@string/wangyou_date" --> <!-- android:textColor="@color/black" --> <!-- android:textSize="14sp" /> --> <!-- <com.easecom.zhwg.ctrl.scene.dzcity.MarqueeText --> <!-- android:id="@+id/wangyou_item_date" --> <!-- android:layout_width="100dip" --> <!-- android:layout_height="wrap_content" --> <!-- android:layout_marginLeft="15dip" --> <!-- android:layout_toRightOf="@id/imgicons" --> <!-- android:ellipsize="marquee" --> <!-- android:focusable="true" --> <!-- android:focusableInTouchMode="true" --> <!-- android:gravity="center|center_vertical|left" --> <!-- android:lines="1" --> <!-- android:marqueeRepeatLimit="marquee_forever" --> <!-- android:scrollHorizontally="true" --> <!-- android:textColor="@color/black" /> --> <com.easecom.zhwg.ctrl.scene.dzcity.MarqueeText android:id="@+id/wangyou_item_date" android:layout_width="95dip" android:layout_height="wrap_content" android:ellipsize="marquee" android:focusableInTouchMode="true" android:gravity="center|center_vertical|left" android:lines="1" android:marqueeRepeatLimit="marquee_forever" android:scrollHorizontally="true" android:textColor="@color/black" android:textSize="14sp" /> <!-- 网元 --> <TextView android:id="@+id/wangyou_item_location" android:layout_width="120dip" android:layout_height="wrap_content" android:layout_marginLeft="2dip" android:gravity="left" android:text="@string/wangyou_location" android:textColor="@color/black" android:textSize="14sp" /> <!-- 选项 --> <TextView android:id="@+id/wangyou_item_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="2dip" android:gravity="left" android:textColor="@color/black" android:textSize="14sp" /> </LinearLayout>
report_wireless2g_dialog
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/bg00" android:orientation="vertical" > <ScrollView android:layout_width="fill_parent" android:layout_height="fill_parent" > <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="vertical" > <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:orientation="horizontal" > <EditText android:id="@+id/start_time" android:layout_width="120dip" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1.03" android:enabled="false" android:hint="@string/start_time" /> <Button android:id="@+id/button1_date" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_weight="0.52" android:text="" /> </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="5dip" android:orientation="horizontal" > <EditText android:id="@+id/end_time" android:layout_width="120dip" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1.03" android:enabled="false" android:hint="@string/end_time" /> <Button android:id="@+id/button2_date" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_weight="0.52" android:text="" > </Button> </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="5dip" android:orientation="horizontal" > <EditText android:id="@+id/spinner_cellname" android:layout_width="120dip" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1.03" android:enabled="false" android:hint="" android:singleLine="true" /> <Button android:id="@+id/button_cellname" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_weight="0.52" android:text="" > </Button> </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="5dip" android:orientation="horizontal" > <EditText android:id="@+id/spinner_zhibiao" android:layout_width="120dip" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1.03" android:enabled="false" android:hint="" android:singleLine="true" /> <Button android:id="@+id/button_zhibiao" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_weight="0.52" android:text="" > </Button> </LinearLayout> <LinearLayout android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_marginTop="10dip" android:orientation="horizontal" > <Button android:id="@+id/yes_query" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1" android:text="@string/query_yes" > </Button> <Button android:id="@+id/no_query" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="5dip" android:layout_marginRight="5dip" android:layout_weight="1" android:text="@string/query_no" > </Button> </LinearLayout> </LinearLayout> </ScrollView> </LinearLayout>
date_layout_dialog
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:layout_marginTop="12dp" android:background="@drawable/layout_bg" android:orientation="vertical" > <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:paddingLeft="12dp" android:paddingRight="12dp" android:paddingTop="30dp" > <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/y" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="年" /> <com.easecom.widget.WheelView android:id="@+id/year" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/y" /> </RelativeLayout> <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/m" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="月" /> <com.easecom.widget.WheelView android:id="@+id/month" android:layout_width="45dp" android:layout_height="wrap_content" android:layout_below="@id/m" /> </RelativeLayout> <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/r" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="日" /> <com.easecom.widget.WheelView android:id="@+id/day" android:layout_width="45dp" android:layout_height="wrap_content" android:layout_below="@id/r" /> </RelativeLayout> <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/s" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="时" /> <com.easecom.widget.WheelView android:id="@+id/hour" android:layout_width="45dp" android:layout_height="wrap_content" android:layout_below="@id/s" /> </RelativeLayout> <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/f" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="分" /> <com.easecom.widget.WheelView android:id="@+id/mins" android:layout_width="45dp" android:layout_height="wrap_content" android:layout_below="@id/f" /> </RelativeLayout> <RelativeLayout android:layout_width="wrap_content" android:layout_height="wrap_content" > <TextView android:id="@+id/se" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:text="秒" /> <com.easecom.widget.WheelView android:id="@+id/seconds" android:layout_width="45dp" android:layout_height="wrap_content" android:layout_below="@id/se" /> </RelativeLayout> </LinearLayout> <!-- <TextView android:id="@+id/value" android:layout_width="wrap_content" android:layout_height="wrap_content" > </TextView> --> </LinearLayout>
report_wireless2g_list
扫描二维码关注公众号,回复:
774218 查看本文章
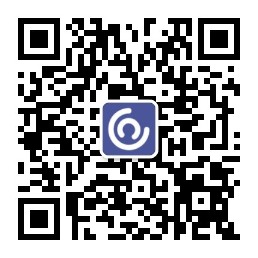
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/list_bg" android:orientation="vertical" > <include android:id="@+id/page_header" layout="@layout/detail_header" /> <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <LinearLayout android:layout_width="fill_parent" android:layout_height="60dip" android:background="@drawable/list_mind" android:gravity="center_vertical" android:orientation="horizontal" android:id="@+id/report_wangyou_line" > <!-- 左侧按钮 --> <Button android:id="@+id/report_wangyou_btleft" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="3dip" android:background="@drawable/button_left" /> <!-- 时间 --> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="2" android:gravity="center_horizontal" android:text="统计时间" android:textColor="@color/black" android:textSize="16sp" /> <!-- 网元 --> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="2.4" android:gravity="center_horizontal" android:text="小区名称" android:textColor="@color/black" android:textSize="16sp" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_weight="2.6" android:gravity="center_vertical" android:orientation="horizontal" > <!-- 选项 --> <TextView android:id="@+id/report_wangyou_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center_horizontal" android:textColor="@color/black" android:layout_weight="1" android:ellipsize="end" android:maxWidth="50dip" android:textSize="16sp" /> <!-- 右侧按钮 --> <Button android:id="@+id/report_wangyou_btright" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="3dip" android:layout_marginRight="3dip" android:background="@drawable/button_right" /> </LinearLayout> </LinearLayout> <ListView android:id="@+id/wangyou_list" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@color/white" android:cacheColorHint="@color/transparent_background" android:divider="@null" > </ListView> </LinearLayout> </LinearLayout>