Given a linked list, return the node where the cycle begins. If there is no cycle, return null
.
To represent a cycle in the given linked list, we use an integer pos
which represents the position (0-indexed) in the linked list where tail connects to. If pos
is -1
, then there is no cycle in the linked list.
Note: Do not modify the linked list.
Example 1:
Input: head = [3,2,0,-4], pos = 1 Output: tail connects to node index 1 Explanation: There is a cycle in the linked list, where tail connects to the second node.
Example 2:
Input: head = [1,2], pos = 0 Output: tail connects to node index 0 Explanation: There is a cycle in the linked list, where tail connects to the first node.
Example 3:
Input: head = [1], pos = -1 Output: no cycle Explanation: There is no cycle in the linked list.
扫描二维码关注公众号,回复:
7724850 查看本文章
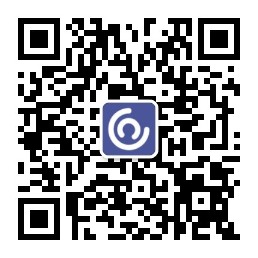
Follow-up:
Can you solve it without using extra space?
Solution:
使用快慢指针,若快慢指针能重合上,那就有环
然后快指针从头移动,与此同时慢指针一起先后移动,当两个指针再次重合时,则就是环的入口!
1 class Solution { 2 public: 3 ListNode *detectCycle(ListNode *head) { 4 if (head == nullptr || head->next == nullptr)return nullptr; 5 ListNode *slow, *fast; 6 slow = fast = head; 7 while (fast && fast->next) 8 { 9 slow = slow->next; 10 fast = fast->next->next; 11 if (fast == slow) 12 break; 13 } 14 if (fast != slow)return nullptr; 15 slow = head; 16 while (slow != fast) 17 { 18 slow = slow->next; 19 fast = fast->next; 20 } 21 return fast; 22 } 23 };