版权声明:本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明。
注: 本文仅用于学习交流分享,[若有不妥之处,请指正,感谢]
关键词:【python】【tkinter】
最后面有 程序 分享
用到的工具有:
- Python 3.6
实现的小功能有:
实现简易幸运转盘式抽奖界面
一、相关函数方法介绍
Tkinter 是 Python 的标准 GUI 库。Python 使用 Tkinter 可以快速的创建 GUI 应用程序。
Tkinter 是 Python 的标准 GUI 库。Python 使用 Tkinter 可以快速的创建 GUI 应用程序。由于 Tkinter 是内置到 python 的安装包中、只要安装好 Python 之后就能 import Tkinter 库。
1.创建窗口【Tk】
- ①导入 Tkinter 模块
- ②创建控件
- ③指定这个控件的 master, 即这个控件属于哪一个
#示例
from tkinter as tk # 导入tk库
root = Tk() #初始化Tk() 建立一个窗口
root.mainloop() #进入消息循环,时刻刷新窗口
2.创建标签【Label】
用法:
Label(根对象, [属性列表])
属性 | 可选项 & 描述 |
---|---|
text | 标签文本 |
bg | 背景颜色 |
font | 字体(颜色, 大小) |
width | 控件宽度 |
height | 控件高度 |
#示例
#创建标签label1
label1 = tk.Label(root, text='谢谢惠顾', bg='yellow', font=('Arial', 50))
#设置标签位置及大小
label1.place(x=0, y=600, width=390, height=250)
3.创建按钮【Button】
用法:
Button (根对象, [属性列表])
属性 | 可选项 & 描述 |
---|---|
activebackground | 当鼠标放上去时,按钮的背景色 |
activeforeground | 当鼠标放上去时,按钮的前景色 |
bg | 按钮的背景色 |
font | 文本字体(颜色, 大小) |
justify | 显示多行文本的时候,设置不同行之间的对齐方式,可选项包括LEFT, RIGHT, CENTER |
height | 按钮的高度 |
image | 按钮上要显示的图片 |
padx | 按钮在x轴方向上的内边距(padding),是指按钮的内容与按钮边缘的距离 |
pady | 按钮在y轴方向上的内边距(padding) |
relief | 边框样式,设置控件3D效果,可选的有:FLAT、SUNKEN、RAISED、GROOVE、RIDGE。默认为 FLAT。 |
width | 按钮的宽度,如未设置此项,其大小以适应按钮的内容(文本或图片的大小) |
height | 控件高度 |
#示例
# 设置启动按键 背景文本为“RUN” 底色为“天蓝” 字体“Arial” 字体大小“50” 回调函数command 为【滚动函数】
btn1 = tk.Button(root, text='RUN', bg='lightSkyBlue', font=('Arial', 50), command=round)
#设置按键坐标及大小
btn1.place(x=800, y=850, width=200, height=200)
二、简易滚动抽奖界面代码
import time
import threading
from PIL import Image
import tkinter as tk # 导入 tk库 模块
import random # 导入 随机库 模块
root = tk.Tk() #初始化Tk() 建立一个窗口
root.title('集大电协简易抽奖') # 设置标题
root.minsize(1000, 700)
photo = tk.PhotoImage(file="ETA.png") # file:图片路径
imgLabel = tk.Label(root, image=photo) # 把图片整合到标签类中
imgLabel.pack(side=tk.RIGHT) # 右对齐
label1 = tk.Label(root, text='谢谢惠顾', bg='yellow', font=('Arial', 50))
label1.place(x=0, y=600, width=390, height=250)
label2 = tk.Label(root, text='简易四轴', bg='yellow', font=('Arial', 50))
label2.place(x=0, y=10, width=390, height=250)
label3 = tk.Label(root, text='谢谢惠顾', bg='yellow', font=('Arial', 50))
label3.place(x=390, y=10, width=390, height=250)
label4 = tk.Label(root, text='mini光立方', bg='yellow', font=('Arial', 50))
label4.place(x=780, y=10, width=390, height=250)
label5 = tk.Label(root, text='再来一次', bg='yellow', font=('Arial', 50))
label5.place(x=1170, y=10, width=390, height=250)
label6 = tk.Label(root, text='谢谢惠顾', bg='yellow', font=('Arial', 50))
label6.place(x=1560, y=10, width=390, height=250)
label7 = tk.Label(root, text='幸运转盘PCB', bg='yellow', font=('Arial', 50))
label7.place(x=1560, y=600, width=390, height=250)
label8 = tk.Label(root, text='谢谢惠顾', bg='yellow', font=('Arial', 50))
label8.place(x=1170, y=600, width=390, height=250)
label9 = tk.Label(root, text='51核心板', bg='yellow', font=('Arial', 50))
label9.place(x=780, y=600, width=390, height=250)
label10 = tk.Label(root, text='再来一次', bg='yellow', font=('Arial', 50))
label10.place(x=390, y=600, width=390, height=250)
label11 = tk.Label(root, text='最终解释权归【集美大学学·生电子技术协会】所有', bg='white', font=('Arial', 20))
label11.place(x=1250, y=900, width=700, height=100)
# 将所有抽奖选项添加到列表
things = [label1, label2, label3, label4, label5, label6, label7, label8, label9, label10]
# 获取列表的最大索引值
maxvalue = len(things) - 1
# 设置起始值为随机整数
starts = random.randint(0, 6)
# 是否停止标志
notround = False
# 定义滚动函数
def round():
t = threading.Thread(target=startup) #启动start
t.start()
# 定义开始函数
def startup():
global starts
global notround
while True:
# 检测停止按钮是否被按下
if notround == True:
notround = False
return starts
# 程序延时
time.sleep(0.017)
# 在所有抽奖选项中循环滚动
for i in things:
i['bg'] = 'lightSkyBlue' #开始时 底色变成天蓝
things[starts]['bg'] = 'red' #滚动框为 红色
starts += 1
if starts > maxvalue:
starts = 0
# 定义停止函数
def stops():
global notround # notround 为全局变量
global starts
notround = True #停止标志位
if starts == 1: # 如果抽中“简易四轴”就跳转为“谢谢惠顾”【奸商^_^】
starts = 2
# 设置启动按键 背景文本为“RUN” 底色为“天蓝” 字体“Arial” 字体大小“50” 回调函数command 为【滚动函数】
btn1 = tk.Button(root, text='RUN', bg='lightSkyBlue', font=('Arial', 50), command=round)
#设置按键坐标
btn1.place(x=800, y=850, width=200, height=200)
# 设置停止按键 背景文本为“RUN” 底色为“红色” 字体“Arial” 字体大小“50” 回调函数command 为【停止函数】
btn2 = tk.Button(root, text='STOP', bg='red', font=('Arial', 50), command=stops)
#设置按键坐标
btn2.place(x=1000, y=850, width=200, height=200)
# 循环,时刻刷新窗口
root.mainloop()
三、界面展示
抽奖测试
![]() |
![]() |
附【Download】:
Dwfish 淹死的鱼 2019.1.22
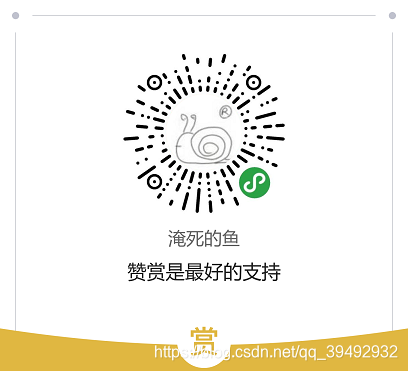