android 程序猿搭建一个简单的服务器后台:
1、环境配置:
(1)、Eclipse (纯净版需安装ee插件)
提示:Help->Install new software->work with: Juno - http://download.eclipse.org/releases/juno(取消PHP相关的勾选)
(2)、配置Web.xml
新增:
1、环境配置:
(1)、Eclipse (纯净版需安装ee插件)
提示:Help->Install new software->work with: Juno - http://download.eclipse.org/releases/juno(取消PHP相关的勾选)
(2)、安装Apache-tomact
2、新建Web项目:
提示:File->new->other->Dynamic Web Project->Next
(1)、填写项目名
(2)、Target Runtime 点击->Apache Tomcat v7.0 ->DownLoad and Install->finish
项目生成如图:
3、新建服务器Java类:
(1)、Java Resources目录下新建:MyHttpServlet
public class MyHttpServlet extends HttpServlet { public MyHttpServlet() { super(); } public void destroy() { super.destroy(); } @Override public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { String username=request.getParameter("username"); String password=request.getParameter("password"); username=new String (username.getBytes("ISO8859-1"),"UTF-8"); password=new String (password.getBytes("ISO8859-1"),"UTF-8"); System.out.println("姓名"+username); System.out.println("密码"+password); if(username.equals("GR")&&password.equals("8888")){ //默认情况下使用的是iso8859——1编码,但是如果发现码表中没有当前字符,会使用当前系统下默认编码:GBK response.getOutputStream().write("登录成功".getBytes("utf-8")); }else{ response.getOutputStream().write("登录失败".getBytes("UTF-8")); } } @Override public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException { this.doGet(request, response); } }
(2)、配置Web.xml
新增:
<servlet> <servlet-name>test</servlet-name> <servlet-class>io.gr.ServerDemo.MyHttpServlet</servlet-class>//文件路径 </servlet> <servlet-mapping> <servlet-name>test</servlet-name> <url-pattern>/Servers</url-pattern>//这里可以自定义 </servlet-mapping>
(3)、双击 Tomcat v7.0 Server at localhost :
扫描二维码关注公众号,回复:
732982 查看本文章
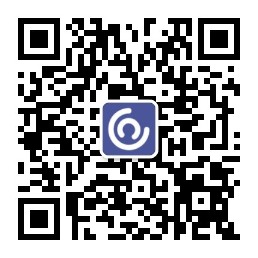
(4)、右键 Tomcat v7.0 Server at localhost,点击Add and Remove —— Add——Finish
(5)、运行测试:
点击:
运行结果:
(6)、请求测试:
打开浏览器,输入:
本地IP+端口号+/项目名+/映射名(即Web.xml中的映射名)+?+参数。。。。
例如:http://127.0.0.1:8080/SimpleAndroidServerDemo/Servers?username=GR&password=8888
请求成功:
Eclipse服务器接收:
4、创建Android项目:
这里就不过多介绍,代码如下:
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.HttpURLConnection;
import java.net.URL;
/**
* Created by GR on 2017/4/7.
*/
public class NetUilts {
/*
* 用post方式登录
* @param username
* @param password
* @return 登录状态
* */
public static String loginofPost(String username,String password){
HttpURLConnection conn=null;
try {
URL url=new URL("http://192.168.1.55:8080/SimpleAndroidServerDemo/Servers");
conn=(HttpURLConnection) url.openConnection();
conn.setRequestMethod("POST");//设置请求方式
conn.setConnectTimeout(10000);//设置连接超时时间
conn.setReadTimeout(5000);//设置读取超时时间
//POST请求的参数
OutputStream out=conn.getOutputStream();//获得输出流对象,用于向服务器写数据
String data="username="+username+"&"+"password="+password;
out.write(data.getBytes());//向服务器写数据;
out.close();//关闭输出流
conn.connect();//开始连接
int responseCode=conn.getResponseCode();//获取响应吗
if(responseCode==200){
//访问成功
InputStream is=conn.getInputStream();//得到InputStream输入流
String state=getstateFromInputstream(is);
return state;
}else{
//访问失败
}
} catch (Exception e) {
e.printStackTrace();
}finally{
if(conn!=null){//如果conn不等于空,则关闭连接
conn.disconnect();
}
}
return null;
}
/*
* 使用GET的方式登录
* @param username
* @param password
* @return 登录状态
* */
public static String loginOfGet(String username,String password){
HttpURLConnection conn=null;
try {
String data="username="+username+"&"+"password="+password;
URL url=new URL("http://192.168.1.55:8080/SimpleAndroidServerDemo/Servers?"+data);
conn=(HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");//设置请求方式
conn.setConnectTimeout(10000);//设置连接超时时间
conn.setReadTimeout(5000);//设置读取超时时间
conn.connect();//开始连接
int responseCode=conn.getResponseCode();//获取响应吗
if(responseCode==200){
//访问成功
InputStream is=conn.getInputStream();//得到InputStream输入流
String state=getstateFromInputstream(is);
Logger.i("请求结果:"+state);
return state;
}else{
//访问失败
Logger.i("访问失败!");
}
} catch (Exception e) {
e.printStackTrace();
Logger.i("访问异常!");
}finally{
if(conn!=null){//如果conn不等于空,则关闭连接
conn.disconnect();
}
}
return null;
}
private static String getstateFromInputstream(InputStream is) throws IOException {
ByteArrayOutputStream baos=new ByteArrayOutputStream();//定义一个缓存流
byte[] buffer=new byte[1024];//定义一个数组,用于读取is
int len=-1;
while((len =is.read(buffer)) != -1){//将字节写入缓存
baos.write(buffer,0,len);
}
is.close();//关闭输入流
String state =baos.toString();//将缓存流中的数据转换成字符串
baos.close();
return state;
}
}
外部调用该方法即可。。。。(记得添加网络权限)
创建服务器主要是为了方便学习和开发自己App的功能调试