AsyncTask可以轻松容易的跟新UI,而开发者需要做的是在不同的回调函数中嵌入代码逻辑即可。关于Android中的线程相关内容,可以参考开发者网站:developer.android.com/guide/components/processes-and-threads.html
开发者网站对AsyncTask的说明已经很明确了,参考地址:http://developer.android.com/reference/android/os/AsyncTask.html,下面从代码角度看看实现过程:
- 继承AsyncTask,定义自己的异步任务;
- 在自定义AsyncTask中的回调函数中嵌入操作逻辑;
- 创建AsyncTask并执行;
主要代码:
import android.app.Activity; import android.os.AsyncTask; import android.os.Bundle; import android.view.View; import android.view.Window; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.TextView; public class RefreshUIActivity extends Activity implements OnClickListener { private TextView textView; private Button refreshButton; /** * 标识异步任务 */ private int identifier; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_PROGRESS); setProgressBarVisibility(true); setContentView(R.layout.main); textView = (TextView) findViewById(R.id.textView1); refreshButton = (Button) findViewById(R.id.button1); refreshButton.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.button1: // 创建异步任务 CustomizedAsyncTask task = new CustomizedAsyncTask(++identifier); task.execute("0", "25", "50", "75", "100"); task = null; break; default: break; } } class CustomizedAsyncTask extends AsyncTask<String, Integer, String> { private String identifier; public CustomizedAsyncTask(int id) { identifier = "[" + id + "]"; } @Override protected void onCancelled() { super.onCancelled(); textView.append(identifier + "onCancelled\n"); } @Override protected void onPostExecute(String result) { super.onPostExecute(result); textView.append(identifier + "onPostExecute result:" + result + "\n"); } @Override protected void onPreExecute() { super.onPreExecute(); textView.append(identifier + "onPreExecute\n"); } @Override protected void onProgressUpdate(Integer... values) { super.onProgressUpdate(values); setProgress(values[0] * 100); textView.append(identifier + "onProgressUpdate Value: " + values[0] + "%\n"); } @Override protected String doInBackground(String... params) { for (String param : params) { try { // 模拟费时操作 Thread.sleep(2 * 1000); // 触发onProgressUpdate方法 publishProgress(Integer.valueOf(param)); } catch (Exception e) { e.printStackTrace(); } System.out.println(param); } System.out.println(identifier + "doInBackground"); return "Finished"; } } }
说明:
- 关于AsyncTask的各个回调函数就不做说明,参考开发者文档即可; 在自定义的AsyncTask的回调函数中,可以更新UI的函数内都出现了对textView的更新操作;还有其它方法更新UI,只要可以达到操作要求,又能让代码易于理解,哪种方式倒是无所谓;
布局文件:
<?xml version="1.0" encoding="utf-8"?> <ScrollView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" > <LinearLayout android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <Button android:id="@+id/button1" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="RefreshUI" /> </LinearLayout> </ScrollView>
一次点击运行效果:
四次点击运行结果:
多说一句:千万不要在protected String doInBackground(String... params)方法中操作UI哦!:)
扫描二维码关注公众号,回复:
722560 查看本文章
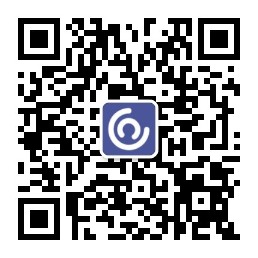