Q:
Given a string containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.
An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Note that an empty string is also considered valid.
Example 1:
Input: "()" Output: true
Example 2:
Input: "()[]{}" Output: true
Example 3:
Input: "(]" Output: false
Example 4:
Input: "([)]" Output: false
Example 5:
Input: "{[]}" Output: true
A:
/** * @param {string} s * @return {boolean} */ var isValid = function(s) { var sl = s.length; while (sl > 0) { s = s.replace('{}', '') s = s.replace('[]', '') s = s.replace('()', '') sl--; } return s == ''; };
Appendix:
Tricks about regex:
- Most common flags :
- i : case insensitive
- g : global (doesn't stop after first match)
- m : multi-line - Most common anchors :
- ^ : Start of string
- $ : End of string
- \A : Start of string (not affected by multi-line content)
- \Z : End of string (not affected by multi-line content) - Most common quantifiers :
- {n} : Exactly n times
- {n,} : At least n times
- {m,n} : Between m and n times
- ? : Zero or one time
- + : One or more times
- * : Zero, one or more times - Most common meta sequences :
- . : Any character but \n and \r
- \w | \W : Any word character | Any non-word character
- \d | \D : Any digit character | Any non-digit character
- \s | \S : Any whitespace character | Any non-whitespace character - Character set :
— [abc] : Will match either a, b or c
- [1-9] : Will match any digit from 1 to 9
- [a-zA-Z] : Will match any letter - Match any character but :
- [^abc] : Matches anything but a, b or c
- Escape a character :
- \character (example : escaping + => \+)
- Refer to a group (also used for capturing groups, look further):
— (group of characters) (example : /(he)+/ will match 'hehehe'
- One group or another :
- | : /^h((ello)|(ola))$/ will match both 'hello' and 'hola'
扫描二维码关注公众号,回复:
7150482 查看本文章
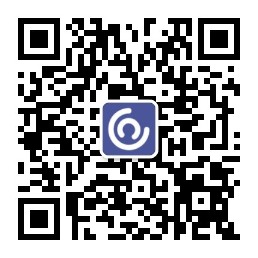
—— END ——
“做一个有趣的人,比做一位物理学家更难。” —— 理查德·费曼