1:需求:将excel中的数据获取出来,转化为json格式,之后输出到.json文件中。
2:步骤:(1): 将excel中的数据获取出来,使用jsonObject转化为json格式字符串
(2):使用输出流将json字符串输出到json文件中
3:需要的依赖:
<dependency> <groupId>org.apache.poi</groupId> <artifactId>poi</artifactId> <version>3.10-FINAL</version> </dependency> <dependency> <groupId>org.apache.poi</groupId> <artifactId>poi-ooxml</artifactId> <version>3.10-FINAL</version> </dependency> <dependency> <groupId>net.sf.json-lib</groupId> <artifactId>json-lib</artifactId> <classifier>jdk15</classifier> <version>2.2.3</version> </dependency> |
4:代码
public class ExcelTojson { //json的key private static String[] titleArray = { "userName", "phoneNum"}; public static void main(String[] args) { InputStream is = null; String excelToJson = excelToJson(is); try { createJsonFile(excelToJson); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } public static String excelToJson(InputStream is) { JSONObject jsonRow = new JSONObject(); try { // 获取文件 is = new FileInputStream("C:\\Users\\Administrator\\Desktop\\新建 XLSX 工作表.xlsx"); // 创建excel工作空间 Workbook workbook = WorkbookFactory.create(is); // 获取sheet页的数量 int sheetCount = workbook.getNumberOfSheets(); // 遍历每个sheet页 for (int i = 0; i < sheetCount; i++) { // 获取每个sheet页 Sheet sheet = workbook.getSheetAt(i); // 获取sheet页的总行数 int rowCount = sheet.getPhysicalNumberOfRows(); // 遍历每一行 for (int j = 0; j < rowCount; j++) { //记录每一行的一个唯一标识作为json的key String key=""; // 获取列对象 Row row = sheet.getRow(j); // 获取总列数 int cellCount = row.getPhysicalNumberOfCells(); Map<String, String> map = new HashMap<String, String>(); //除去第一行的标题 if (j > 0) { // 遍历所有数据 for (int n = 0; n < cellCount; n++) { // 获取每一个单元格 Cell cell = row.getCell(n); // 在读取单元格内容前,设置所有单元格中内容都是字符串类型 cell.setCellType(Cell.CELL_TYPE_STRING); // 按照字符串类型读取单元格内数据 String cellValue = cell.getStringCellValue(); if(n==1) { key=cellValue; } map.put(titleArray[n], cellValue); } } if (map.size() != 0) { jsonRow.put(key,map); } }
扫描二维码关注公众号,回复:
7078791 查看本文章
} System.out.println(jsonRow.toString()); } catch (Exception e) { } return jsonRow.toString(); } public static void createJsonFile(String jsonData) throws IOException { File file = new File("C:\\Users\\Administrator\\Desktop\\用户.json"); //连接流 FileOutputStream fos=null; //链接流 BufferedOutputStream bos =null; try { fos = new FileOutputStream(file); bos = new BufferedOutputStream(fos); bos.write(jsonData.getBytes());; bos.flush(); } catch (FileNotFoundException e) { // TODO Auto-generated catch block e.printStackTrace(); }finally { if(null!=bos) { bos.close(); } if(null!=fos) { fos.close(); } } } } |
4:效果
excel文件数据:
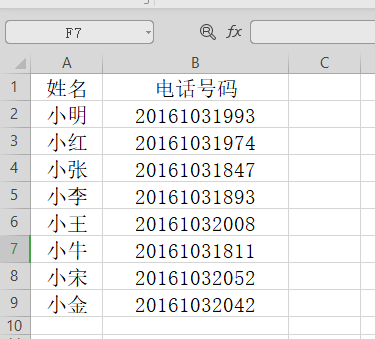
json文件
{"20161031993":{"phoneNum":"20161031993","userName":"小明"},"20161031974":{"phoneNum":"20161031974","userName":"小红"},"20161031847":{"phoneNum":"20161031847","userName":"小张"},"20161031893":{"phoneNum":"20161031893","userName":"小李"},"20161032008":{"phoneNum":"20161032008","userName":"小王"},"20161031811":{"phoneNum":"20161031811","userName":"小牛"},"20161032052":{"phoneNum":"20161032052","userName":"小宋"},"20161032042":{"phoneNum":"20161032042","userName":"小金"}} |
5:改进
可以查找java代码,将输出的json格式化,使其更加美观。