#include<iostream>
using namespace std;
typedef struct{
char data[100];
int length;
} SqString;
//串赋值
void assign(SqString &s,char a[]){
int i=0;
while(a[i]!='\0'){
s.data[i]=a[i];
i++;
}
s.length=i;
}
//串复制
void strcpy(SqString &s,SqString t){
for(int i=0;i<t.length;i++){
s.data[i]=t.data[i];
}
s.length=t.length;
}
//求串长
int getLength(SqString s){
return s.length;
}
//判断串相等
int isEquel(SqString s,SqString t){
if(s.length!=t.length){
return 0;
}else{
for(int i=0;i<s.length;i++){
if(s.data[i]!=t.data[i]){
return 0;
}
}
return 1;
}
}
//串连接
SqString concat(SqString s,SqString t){
SqString rst;
for(int i=0;i<s.length;i++){
rst.data[i]=s.data[i];
}
for(int j=0;j<t.length;j++){
rst.data[j+s.length]=t.data[j];
}
rst.length=s.length+t.length;
return rst;
}
//求子串
SqString getSon(SqString &s,int i,int l){
SqString rst;
if(i<1||l<1||l>s.length||i+l-1>s.length){
rst.length=0;
}else{
for(int j=i-1;j<i+l-1;j++){
rst.data[j-i+1]=s.data[j];
}
rst.length=l;
}
return rst;
}
//查找定位位置
int index(SqString s,SqString t){
int i=0;
int j=0;
while(i<s.length&&j<t.length){
if(s.data[i]==t.data[j]){
i++;
j++;
}else{
i=i-j+1;
j=0;
}
} //此处返回的逻辑位置有点难理解
if(j>=t.length){
return i-t.length+1;//返回子串的实际开始位置 i-t.length得到逻辑开始位置
}else{
return 0;
}
}
//子串插入
/*
思路:
先将第i个位置开始的元素后移动t.length个位置
再将t的值插入
*/
int insStr(SqString &s,SqString t,int i){
for(int j=s.length-1;j>=i-1;j--){
s.data[j+t.length]=s.data[j];//注意是空出t.length个位置
}
for(int j=0;j<t.length;j++){
s.data[i-1+j]=t.data[j];
}
s.length=s.length+t.length;
}
//子串删除
/*
从第i-1个位置开始删除l个元素
应该将i+l-1开始的元素向前移动l个位置
直到到末尾
*/
int delStr(SqString &s,int i,int l){
if(i<1||l<1||l>s.length||i+l-1>s.length){
return 0;
}else{
for(int j=i+l-1;j<s.length;j++){
s.data[j-l]=s.data[j];
}
s.length=s.length-l;
return 1;
}
}
//显示
void disStr(SqString s){
cout<<endl;
for(int i=0;i<s.length;i++){
cout<<s.data[i]<<" ";
}
cout<<endl;
}
//子串替换
void replaceStr(SqString &s,SqString t,SqString r){
int i = index(s,t);//获取位置
while(i!=0){
delStr(s,i,t.length);
insStr(s,r,i);
disStr(s);
i = index(s,t);
}
}
int main(){
/*
字符串的基本运算
串赋值 assign
串复制 strcpy
求串长 getLength
判断串相等 isEquel
串连接 concat
求子串 getSon
查找定位位置 index
子串插入 insStr
子串删除 delStr
子串替换 replaceStr
输出串 disStr
*/
SqString s,s2,rst,r;
char a[]="world",b[]="helloworldsdfworld",c[]="l";
assign(s,a);
assign(r,c);
assign(s2,b);
cout<<"s2长度"<<getLength(s2)<<endl;
disStr(s2);
strcpy(rst,s);
disStr(rst);
disStr(concat(s2,s));
disStr(s);
if(isEquel(s,s2)){
cout<<"相等";
}else{
cout<<"不相等";
}
delStr(s,4,3);
disStr(s2);
disStr(s);
disStr(r);
replaceStr(s2,s,r);
disStr(s2);
assign(s,a);
assign(r,c);
assign(s2,b);
cout<<"s的长度"<<getLength(s)<<"如下"<<endl;
disStr(s);
cout<<"r的长度"<<getLength(r)<<"如下"<<endl;
disStr(r);
cout<<"s2的长度"<<getLength(s2)<<"如下"<<endl;
disStr(s2);
cout<<index(s2,s);
replaceStr(s2,s,r);
disStr(s2);
return 0;
}
结果:
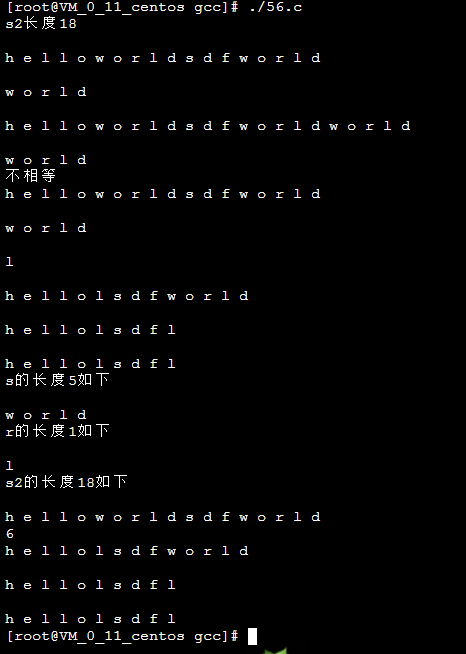