记录下thinkphp5自定义底层基类、内部类函数使用笔记 大部分笔记来自tp手册。
底层常用代码的封装
在控制器中基类的起着至关重要的作用,整个项目的代码安全,复杂程度,易读性都要看你项目的基类架构的.
比如api中都需要某一个功能函数,我们就可以写在基类里。
贴上一个基类返回错误码的例子:
<?php
namespace app\member\controller; class Base extends \app\base\controller\Base { static public function showReturnCode($code = '', $data = [], $msg = '') { $return_data = [ 'code' => '500', 'msg' => '未定义消息', 'data' => $code == 1001 ? $data : [], ]; if (empty($code)) return $return_data; $return_data['code'] = $code; if(!empty($msg)){ $return_data['msg'] = $msg; }else if (isset(ReturnCode::$return_code[$code]) ) { $return_data['msg'] = ReturnCode::$return_code[$code]; } return $return_data; } static public function showReturnCodeWithOutData($code = '', $msg = '') { return self::showReturnCode($code,[],$msg); } }
基类:
<?php namespace app\base\controller; class ReturnCode { static public $return_code = [ '1001' => '操作成功', '1002' => '你想做什么呢', //非法的请求方式 非ajax '1003' => '请求参数错误', //如参数不完整,类型不正确 '1004' => '请先登陆再访问', //未登录 或者 未授权 '1005' => '请求授权不符', ////非法的请求 无授权查看 '1006' => '数据加载失败', // '1010' => '数据不存在', // '1020' => '验证码输入不正确', // '1021' => '用户账号或密码错误', // '1022' => '用户账号被禁用', // '1030' => '数据操作失败', // ]; }
然后就可以调用了
private function getUid(){ //数据库字段 网页字段转换 $param = [ 'userid' => 'userid', 'userpwd' => 'userpwd', 'mobile' => 'mobile', ]; $param_data = $this->buildParam($param); if (empty($param_data['userid'])&&empty($param_data['mobile'])) return self::showReturnCodeWithOutData(1003); $check_login = $this->doModelAction($param_data, 'base/Member.login', 'base/Member', 'checkLogin'); if (!isset($check_login['code'])) $this->showReturnCodeWithSaveLog(1111); if ($check_login['code'] == 1001) { } return $check_login; }
扫描二维码关注公众号,回复:
7006588 查看本文章
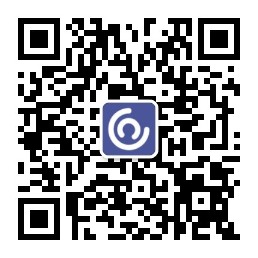
框架构造函数使用
构造函数 在类的初始化时候执行的一个方法,构造函数中不要使用return关键词。
那么我们经常在构造函数里面做做什么呢?
- 数据初始化 注入对象等
- 前置工作的处理
- 权限判断
我们看一下TP5 控制器的构造函数,他在初始化时候注入了request对象,我们继承了控制器,直接就可以使用$this->request调用,同时也封装了View类.同时对前置的beforeActionList属性的方法 进行处理.
public function __construct(Request $request = null) { if (is_null($request)) { $request = Request::instance(); } $this->view = View::instance(Config::get('template'), Config::get('view_replace_str')); $this->request = $request; // 控制器初始化 $this->_initialize(); // 前置操作方法 if ($this->beforeActionList) { foreach ($this->beforeActionList as $method => $options) { is_numeric($method) ? $this->beforeAction($options) : $this->beforeAction($method, $options); } } }
对于权限判断的例子:
public function _initialize() { parent::_initialize(); // TODO: Change the autogenerated stub $user_agent = $this->request->server('HTTP_USER_AGENT'); if (! strpos($user_agent, 'MicroMessenger') === false ) $this->isWechatBrowser = true; //判断提交方式和是否微信浏览器 if ($this->request->method() == 'GET' && $this->isWechatBrowser === true){ //未登录 重新登录 if (!$this->checkAuth()&& !$this->no_login ) $this->wxoauth(); $this->isLogin=true; //设置全局登录 $this->loginGlobal(); if(!$this->isReg){ if(!$this->checkUuidMobile()) $this->redirect('user/user_blind.html'); } } }
控制起来继承:
<?php namespace app\api\controller; class WxpayAction extends Auth { public function _initialize() { config('default_return_type','html'); parent::_initialize(); // TODO: Change the autogenerated stub } public function index($order_no='2017020453102495'){ if(!$this->isWechatBrowser){ //******* 为了演示方便 这里省略了订单的是否支付验证 另外 微信支付本身就有支付重复验证 这里并没有加入乐观锁过滤 //******* $data=controller('base/WxPay')->payByOrderNo($order_no); $assign_data=[ 'title'=>'为了家健康--订单支付', 'amount'=>$data['amount'], 'order_no'=>$order_no, "jsApiParameters" =>$data['jsApiParameters'], 'openid'=>$this->open_id, 'data_md5'=>md5($order_no.$this->open_id.$data['amount']), //md5验证( 订单号 openid 金额) ]; $this->assign($assign_data); return $this->fetch('wxpay/index'); } public function showOrdersPayOk($order_no,$order_amount,$data_md5){ //md5验证( 订单号 openid 金额) if (md5($order_no.$this->open_id.$order_amount)<>$data_md5){ $assign_data=[ 'title'=>'为了家健康--支付出错了', 'content'=>'你要支付的订单号不存在请核对后再支付!', ]; $this->assign($assign_data); return $this->fetch('wxpay/err'); }else{ $assign_data=[ 'title'=>'为了家健康--该订单已经支付成功', 'amount'=>$order_amount, 'order_no'=>$order_no, ]; $this->assign($assign_data); return $this->fetch('wxpay/ok'); } } public function jsSign($url=''){ $url= $url ? : $this->request->server('HTTP_REFERER'); return json(controller('base/WxApi')->getJsSign($url)); }
框架析构函数使用
析构函数就是一个收尾的一个方法,
例子:创建一个虚拟基类
<?php namespace app\base\controller; use think\Cache; use think\Controller; use think\Session; use think\Loader; abstract class Base extends Controller { protected $error; //出错时候的记录 protected $log=[]; //要保存的记录 protected $saveLog = false ; static public function showReturnCode($code = '', $data = [], $msg = '') { $return_data = [ 'code' => '500', 'msg' => '未定义消息', 'data' => $code == 1001 ? $data : [], ]; if (empty($code)) return $return_data; $return_data['code'] = $code; if(!empty($msg)){ $return_data['msg'] = $msg; }else if (isset(ReturnCode::$return_code[$code]) ) { $return_data['msg'] = ReturnCode::$return_code[$code]; } return $return_data; } protected function addLog($code='',$msg=''){ $this->log[] =[ 'uuid' => $this->uuid, 'url' => $this->request->url(true), 'method' => $this->request->method(), 'data' => $this->getData(), 'ip' => $this->request->ip(), 'code'=>$code, 'desc' => $msg, ]; } protected function toSaveLog(){ $this->saveLog = true ; $this->addLog(); } protected function showReturnCodeWithSaveLog($code = '', $data = [], $msg = ''){ $this->saveLog = true ; $this->addLog($code,$msg); return self::showReturnCode($code, $data, $msg); } protected function getData(){ if ($this->request->isPost()){ return $this->request->post(); }else{ return $this->request->get(); } } protected function saveLogAction(){ if (!empty($this->log)){ foreach($this->log as $value){ dump($value); } } } public function __destruct() { // TODO: Implement __destruct() method. //记录日志 if (!empty($this->log) && $this->saveLog == true){ $this->saveLogAction(); } } }
控制器继承:
<?php namespace app\member\controller; use app\base\model\Member; use think\Cache; use think\Db; class Test extends Base { public function index(){ return $this->showReturnCodeWithSaveLog(1001,'演示析构函数成功了'); } }