阅读《笨方法学python3》,归纳的知识点
习题1:安装环境+练习 print函数使用 主要区别双引号和单引号的区别
习题2:注释符号#
习题3:运算符优先级,跟C/C++, Java类似
以下运算符优先级:从下往下以此递增,同行为相同优先级
Lambda #运算优先级最低 逻辑运算符: or 逻辑运算符: and 逻辑运算符:not 成员测试: in, not in 同一性测试: is, is not 比较: <,<=,>,>=,!=,== 按位或: | 按位异或: ^ 按位与: & 移位: << ,>> 加法与减法: + ,- 乘法、除法与取余: *, / ,% 正负号: +x,-x
习题4:变量名+打印 介绍了以下这种形式的打印
print("There are", cars, "cars available.")
习题5:更多打印方式 比如 f"XXX"
my_name = 'Zed A. Shaw'
print(f"Let's talk about {my_name}")
习题6:继续使用f"XX"打印
习题7:format打印方式,可采用end=‘ ’代替换行
print("Its fleece was white as {}.".format('show')) print(end1 + end2 + end3 + end4 + end5 + end6, end=' ') print(end7 + end8 +end9 + end10 +end11 + end12) 显示如下: Its fleece was white as show. Cheese Burger
习题8:更多打印方式(这种打印方式见的较少)
formatter = '{} {} {} {}' print(formatter.format(1, 2, 3, 4)) print(formatter.format("one", "two", "three", "four")) print(formatter.format(True, False, False, True)) print(formatter.format(formatter, formatter, formatter, formatter)) # print("\n") print(formatter.format( "Try your", "Own text here", "Maybe a poem", "Or a song about fear" )) 显示如下: 1 2 3 4 one two three four True False False True {} {} {} {} {} {} {} {} {} {} {} {} {} {} {} {} Try your Own text here Maybe a poem Or a song about fear
习题9:多行打印,换行打印
扫描二维码关注公众号,回复:
6925679 查看本文章
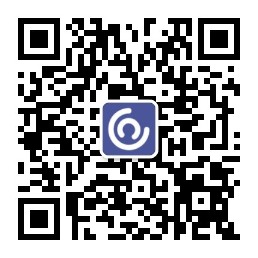
小结:打印方式
1)print("There are", cars, "cars available.") # 变量名直接打印 2)print(f"Let's talk about {my_name}") # 带有f"{}"的打印方式 3) 变量名+format() hilarious = False joke_evaluation = "Isn't that joke so funny?! {}" print(joke_evaluation.format(hilarious)) 4)print("Its fleece was white as {}.".format('show')) # 5)formatter = '{} {} {} {}' print(formatter.format(1, 2, 3, 4)) print(formatter.format("one", "two", "three", "four")) 6)print(''' There's something going on here. With the three double-quotes. We'll be able to type as much as we like. Even 4 lines if we want,or 5,or 6 ''') # 多行打印
习题10:转义字符
习题11:介绍 input()函数,并使用到习题5介绍的打印方式
print("How old are you?", end=' ') age = input() print("How tall are you?", end=' ') height = input() print("How much do you weight?", end=' ') weight = input() print(f"So,you're {age} old,{height} tall and {weight} heavy.")
习题12:介绍input()函数 加提示符
age = input("How old are you?")
print("How old are you? {}".format(input())) # 先运行后面的在运行前面的提示
习题13: 参数、解包和变量
from sys import argv # read the WYSS section for how to run this script, first, second, third = argv print("The script is called:", script) print("Your first variable is:", first) print("Your second variable is:", second) print("Your third variable is:", third) # 注: 把argv中的东西取出,解包,将所有的参数依次复制给左边的这些变量 >>python ex13.py first second third
练习14:提示与传递 主要讲解 input()函数 配合输入格式
from sys import argv ''' PS E:\dev\code\笨方法学python3> python ex14.py Zed Hi Zed,I'm the ex14.py script.' I'd like to ask ypu a few questions. Do ypu like me Zed? >yes Where do ypu live Zed? >yicheng What kind of computer do you have? >Dell ''' script , user_name = argv prompt = '>' print(f"Hi {user_name},I'm the {script} script.'") print("I'd like to ask you a few questions.") print(f"Do ypu like me {user_name}?") likes = input(prompt) print(f"Where do you live {user_name}?") lives = input(prompt) print("What kind of computer do you have?") computer = input(prompt) print(f''' Alright, so you said {likes} about liking me. You live in {lives}. Not sure where that is. And you have a {computer} computer. Nice. ''')
练习15:读取文件
txt = open(filename) print(f"Here's your file {filename}:") print(txt.read()) txt.close()
练习16:读写文件
close:关闭文件。跟你的编辑器中的“文件”->“保存”是一个意思 read:读取文件的内容 readline:只读取文本文件中的一行 truncate:清空文件,请小心使用该命令 write('stuff'):将“stuff”写入文件 seek(0): 将读写位置移动到文件开头
练习17:继续读写文件
in_file = open(from_file) indata = in_file.read() out_file = open(to_file,'w') out_file.write(indata)
练习18:函数
练习19:函数与变量
pytyon在定义函数的时候跟C/C++区别蛮大,python不需要定义参数类型,定义某一函数时 可参考如下:
def function(vailable1, vailable2,*):
练习20: 函数和文件
from sys import argv script, input_file = argv def print_all(f): print(f.read()) def rewind(f): f.seek(0)
# 带有行号的打印内容 def print_a_line(line_count,f): print(line_count, f.readline()) current_file = open(input_file) # 接受的参数,打开文件 print("First let's print the whole file:\n") print_all(current_file) # 打印该文件 print("Now let's rewind, kind of like a tape.") rewind(current_file) # 将读写位置移动到文件开头(第一个字符的前面一个位置) 方便后续读取 如不执行该操作,后续打印为空 print("Let's print three lines:") current_line = 1 print_a_line(current_line,current_file) current_line = current_line + 1 print_a_line(current_line,current_file) current_line = current_line + 1 print_a_line(current_line, current_file)