1. 题意理解:
给出两条string,让判断它们是否是同构(isomorphic).
Isomorphic: two strings are isomorphic if the characters in string s can be replaced to get string t.
2. 解题关键词:hashmap
1)首先建立一个hashmap,key 对应的是来自s的char,value 对应的是来自t 的 char,以此来记录分别来自string s 和 string t 中每个char的映射。
比如 "paper" 和 “title”:
key | val
p -> t p as a key does not exists in map, check if t as value has existed in map, if yes, return false; if not, add p as key and t as value.
a -> i a as a key does not exists in map, check if i as value has existed in map, if yes, return false; if not, add p as key and i as value.
p -> t p as a key already existed in map. check if its corresponding value equals t, if yes, skip, if not, return false.
e -> l e as a key does not exists in map, check if l as value has existed in map, if yes, return false; if not add e as key and l as value.
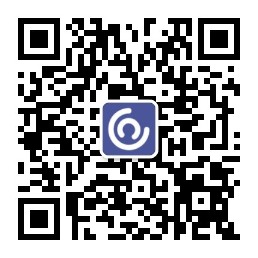
r -> e r as a key does not exists in map, check if e as value has existed in map, if yes, return fals; if not add r as key and e as value.
DONE.
3. 注意事项:多练几遍。。
4. Java 代 class Solution {
public boolean isIsomorphic (String s, String t) { Map<Character, Character> charStoCharT = new hashMap<>(); int sLength = s.length(); int tLength = t.length(); //base case: 如果两条string长度根本不同, //已经没有必要再比下去,对比的最基本条件是当两条string的长度相同。 if (sLength != tLength) { return false; } for (int i = 0; i < sLength; i++) { char fromS = s.charAt(i); char fromT = t.charAt(i); if (! charStoCharT.containsKey(fromS)) { //可能1: 如果来自s的char不在map里,首先要确定与之对应的来自t的char是否已经 //在map里。如果已经在,直接返回false。如果不在,建立新的映射。 if (charStoCharT.containsValue(fromT)) { return false; } else { charStoCharT.put(fromS, fromT); } } else {
//可能2:来自s的char已经在map,这时就要把它对应的value拎出来看看是不是现在当前的from t, 如果是,略过,如果不是,返回false. if (charStoCharT.get(fromS) != from T) return false; else { continue; } } return true; //顺利走完return true }