概述:
MySQL的SQL语句类别划分
DDL(data definition language):数据库定义语言
定义了不同的数据库,数据库表,列(字段),索引等数据库对象的定义;
常用SQL:
- create(创建): 创建库,创建表;
- drop(删除):删除库,删除表,删除字段(和alter搭配使用)
- alter(更新): 更新字段;
DML(data manipulation language):数据操控语言
用于添加,删除,变更,查询数据库记录。
常用SQL:
- insert: 添加;
- delete:删除;
- update: 更新;
- select:查询;
DCL(data control language):数据控制语言
控制不同数据库段直接访问和访问级别的语句-》给用户设置权限
常用SQL:
- grant : 允许,授予
- remove: 删除
例:
用户表(用户名,性别,地址)
张三 男 西安
需求: 增加字段(地址): DDL
将男修改为女: DML
启动数据库:
net start mysql
登陆数据库:
-u root -p
命令行大小写输入均可。
操作数据库
创建数据库:
create database 数据库名;
mysql> create database base;
Query OK, 1 row affected (0.12 sec)
查看数据库:
show databases
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| base |
| information_schema |
| mysql |
| performance_schema |
| sqldemo |
| sys |
+--------------------+
6 rows in set (0.00 sec)
删除数据库:
drop database 数据库名;
mysql> drop database base;
Query OK, 0 rows affected (0.23 sec)
使用数据库:
mysql> use sqldemo;
Database changed
查看数据库下的表
mysql> show tables;
+-------------------+
| Tables_in_sqldemo |
+-------------------+
| demo |
| demo1 |
| example0 |
| example1 |
| example2 |
| example3 |
| example4 |
| example5 |
| example6 |
| example7 |
| user |
+-------------------+
11 rows in set (0.00 sec)
操作表
操作表之前需要先进入某个数据库。
创建表:
mysql中,创建表是通过SQL语句 create table 实现的。
语法为
create table 表名(属性名 数据类型 [完整性约束条件],
属性名 数据类型 [完整性约束条件],
属性名 数据类型
);
其中表名参数表示所要创建表的名称,属性名参数表示表中字段的名称,数据类型参数指定字段的数据类型。完整性约束条件为某些特定字段的约束条件。
注意:
表名不能是SQL关键字。如create,updata,order等都不能做表名。一个表可以有一个或者多个属性。定义时,字母大小写均可,各属性之间用逗号隔开,最后一个属性不需要逗号。
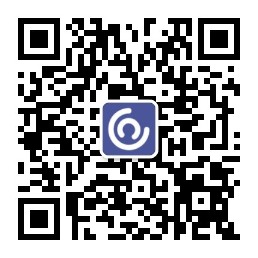
完整性约束:
完整性约束 | 说明 |
---|---|
primary key | 表示当前属性为该表的主键,可以区分不同的行记录 |
foreign key | 修饰的属性为该表的外键,是与之联系的某表主键 |
not null | 表示属性值不能为空 |
unique | 表示属性值是唯一的 |
auto_increment | 表示属性是自增的,自增类型为整型 |
default | 给属性默认值 |
创建单字段主键表:
mysql> create table example1(stu_id int primary key,
-> stu_name varchar(20),
-> stu_sex boolean
-> );
Query OK, 0 rows affected (0.53 sec)
创建多字段(联合)主键表
mysql> create table example2(stu_id int,
-> course_id int,
-> grade float,
-> primary key(stu_id,course_id)
-> );
Query OK, 0 rows affected (0.42 sec)
创建有外键的表:
字段sno是表A的属性,且依赖表B的主键。那么表B为父表,表A为子表,sno为表A的外键。通过sno字段将父表B和子表A建立关联关系。
外键的作用是建立该表与其父表的关联关系。父表中删除某条信息是,子表与之对应的信息也必须有相应的改变。
语法规则:
constraint 外键别名 foreign key(属性1.1,属性1.2,...属性1.n)
references 表名(属性2.1,属性,2.2,.......)
外键别名就是外键的代号,属性1参数列表为子表中设置的外键;
表名 参数是指父表的名称; 属性2 参数列表为父表的主键。
mysql> create table example3(
-> id int primary key,
-> stu_id int,
-> course_id int ,
-> constraint c_fk foreign key(stu_id,course_id)
-> references example2(stu_id,course_id)
-> );
Query OK, 0 rows affected (0.55 sec)
- 注意: 子表的外键必须是父表的主键,并且数据类型必须一致。
创建有非空约束的表
非空性是指字段的值不能为空值(null)。 非空约束将保证所有记录中该字段都有值。如果用户新插入值为空值,则数据库会报错。
语法规则:
属性名 数据类型 not null
例:设置id和name的非空约束 ,顺带设置了外键。
mysql> create table example4(
-> id int not null primary key,
-> name varchar(20) not null,
-> stu_id int,
-> constraint c_fk foreign key(stu_id)
-> references example1(stu_id)
-> );
创建有唯一性约束的表:
唯一性约束是指所有记录中该字段的值不能重复出现。设置表的唯一性约束是指在创建表时,为表的某些特殊字段加上unique约束条件。
语法:
属性名 数据类型 unique
例:设置stu_id的唯一性约束。
mysql> create table example5(
-> id int primary key,
-> stu_id int unique,
-> name varchar(20) not null);
Query OK, 0 rows affected (0.47 sec)
设置表的属性值自动加1的表:
auto_increment 是MYSQL中一个特殊的约束条件。其主要目的用于为表中插入的新纪录自动生成唯一的ID。一个表里面只能有一个字段使用此约束,并且该字段必须成为主键的一部分 。auto_increment约束的字段可以是任何整数类型,默认情况下,该字段的值是从1开始自增。 使用了此字段就不需要使用 not null,default ,unique 等约束条件。
语法规则:
如果是创建表时设置,此字段必须跟 primary key。
属性名 数据类型 auto_increment
例:设置id为自动增加字段
mysql> create table example6(
-> id int primary key auto_increment,
-> stu_id int unique,
-> name varchar(20) not null);
Query OK, 0 rows affected (0.42 sec)
如果插入的第一条记录设置了初值,那么新增加的记录就会从初值开始增加。
创建有默认属性的表:
创建表时可以指定表中字段的默认值,如果插入一条新纪录没有为这个字段赋值,那么系统会自动为这个字段插入默认值。
- 字段没有设置default,那么默认值为null。
语法规则:
属性名 数据类型 default 默认值
例:
mysql> create table example7(id int primary key auto_increment,
-> stu_id int unique,
-> name varchar(20) not null,
-> English varchar(20) default 'zero',
-> Math float default 0,
-> computer float default 0);
Query OK, 0 rows affected (0.46 sec)
查看表结构:
查看表基本结构:describe/desc(描述)
查看表的基本定义。其中包括 字段名,字段数据类型,是否为主键和默认值。
语法:
describe 表名;
或者
desc 表名;
例:
mysql> describe example4;
+--------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+-------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | NO | | NULL | |
| stu_id | int(11) | YES | MUL | NULL | |
+--------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
查看表详细结构 : show create table
show create table 可以查看表的详细定义,该语句可以查看表的字段名,字段的数据类型,完整性约束等信息。此外还能查看表默认的存储引擎和字符编码。
语法:
show create table 表名;
show create table 表名 \G
- 加个\G 可以使内容美观。
例:
mysql> show create table example4\G
*************************** 1. row ***************************
Table: example4
Create Table: CREATE TABLE `example4` (
`id` int(11) NOT NULL,
`name` varchar(20) NOT NULL,
`stu_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `d_fk` (`stu_id`),
CONSTRAINT `d_fk` FOREIGN KEY (`stu_id`) REFERENCES `example1` (`stu_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
1 row in set (0.00 sec)
修改表
MYSQL通过alter table 语句来修改表,修改表包括修改表名,修改字段数据类型,修改字段名,增加字段,删除字段,修改字段排列位置,修改默认存储引擎,删除表的外键约束等 。
修改表名:
语法:
alter table 旧表名 rename [to] 新表名;
to为可选参数
例:将example0修改为 example
mysql> alter table example0 rename to example;
Query OK, 0 rows affected (0.19 sec)
//查看是否还存在此表
mysql> desc example0;
ERROR 1146 (42S02): Table 'sqldemo.example0' doesn't exist
修改字段的数据类型
字段的数据类型包括整数型,浮点数型,字符串型,二进制型,日期和时间等类型。 数据类型决定了数据的存储格式,约束条件和有效范围。
语法:
alter table 表名 modify 属性名 数据类型;
数据类型指修改后新的数据类型。
例:修改name字段的数据类型 varchar->char
mysql> desc example ;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | varchar(20) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
mysql> alter table example modify name char;
Query OK, 0 rows affected (0.78 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> describe example;
+-------+------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
+-------+------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
修改字段名:
语法:
alter table 表名 change 旧属性名 新属性名 新数据类型;
- 若数据类型不需要修改,设置成原来的即可。
- 只修改字段名:
mysql> desc example1;
+----------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+----------+-------------+------+-----+---------+-------+
| stu_id | int(11) | NO | PRI | NULL | |
| stu_name | varchar(20) | YES | | NULL | |
| stu_sex | tinyint(1) | YES | | NULL | |
+----------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
mysql> alter table example1 change stu_name name varchar(20);
Query OK, 0 rows affected (0.13 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example1;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| stu_id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | YES | | NULL | |
| stu_sex | tinyint(1) | YES | | NULL | |
+---------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
- 修改字段名和字段数据类型:
mysql> desc example1;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| stu_id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | YES | | NULL | |
| stu_sex | tinyint(1) | YES | | NULL | |
+---------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
mysql> alter table example1 change stu_sex sex int(2);
Query OK, 0 rows affected (1.03 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example1;
+--------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+-------------+------+-----+---------+-------+
| stu_id | int(11) | NO | PRI | NULL | |
| name | varchar(20) | YES | | NULL | |
| sex | int(2) | YES | | NULL | |
+--------+-------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
增加字段:
语法:
alter table 表名 add 属性名1 数据类型 [完整性约束条件] [first | after 属性名2]
中括号里面的都是非必要条件, first作用是将新增字段作为表的第一个字段。after 属性名2 作用是将新增字段添加到属性名2所指的字段后。没有指定字段插入位置,默认插入末尾。
- 增加无完整性约束的字段:默认末尾添加
mysql> desc example;
+-------+------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
+-------+------------+------+-----+---------+-------+
3 rows in set (0.00 sec)
mysql> alter table example add phone varchar(20);
Query OK, 0 rows affected (0.32 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
- 增加有完整性约束的字段:
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
+-------+-------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
mysql> alter table example add age int(4) not null;
Query OK, 0 rows affected (0.29 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| age | int(4) | NO | | NULL | |
+-------+-------------+------+-----+---------+-------+
5 rows in set (0.00 sec)
- 表的第一个位置增加字段:
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| age | int(4) | NO | | NULL | |
+-------+-------------+------+-----+---------+-------+
5 rows in set (0.00 sec)
mysql> alter table example add num int(8) primary key first;
Query OK, 0 rows affected (0.53 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| age | int(4) | NO | | NULL | |
+-------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
- 表的指定位置增加字段:
mysql> desc example;
+-------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+-------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| age | int(4) | NO | | NULL | |
+-------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
mysql> alter table example add address varchar(30) not null after phone;
Query OK, 0 rows affected (0.92 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
7 rows in set (0.00 sec)
删除字段:
语法:
alter table 表名 drop 属性名;
删除字段和删除数据库使用的关键字都是 drop
例:
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| id | int(11) | YES | | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
7 rows in set (0.00 sec)
mysql> alter table example drop id;
Query OK, 0 rows affected (0.68 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
修改字段的排列位置:
语法:
alter table 表名 motify 属性名1 数据类型 first|after 属性名2;
属性名1 指需要修改位置的字段名称,数据类型 指属性名1的数据类型, first指定位置为表的第一个位置,after 属性名2 指定属性名1 插在属性名2后。
- 字段修改到第一个位置:
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| num | int(8) | NO | PRI | NULL | |
| name | char(1) | YES | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
mysql> alter table example modify name char first;
Query OK, 0 rows affected (0.60 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| name | char(1) | YES | | NULL | |
| num | int(8) | NO | PRI | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
mysql>
- 字段修改到指定位置:
mysql> desc example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| name | char(1) | YES | | NULL | |
| num | int(8) | NO | PRI | NULL | |
| sex | tinyint(1) | YES | | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
+---------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
mysql> alter table example modify sex tinyint(1) after age;
Query OK, 0 rows affected (0.58 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> describe example;
+---------+-------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+---------+-------------+------+-----+---------+-------+
| name | char(1) | YES | | NULL | |
| num | int(8) | NO | PRI | NULL | |
| phone | varchar(20) | YES | | NULL | |
| address | varchar(30) | NO | | NULL | |
| age | int(4) | NO | | NULL | |
| sex | tinyint(1) | YES | | NULL | |
+---------+-------------+------+-----+---------+-------+
6 rows in set (0.00 sec)
更改表的存储引擎:
MYSQL存储引擎有InNoDB,MyISAM,MEMORY 等 ;
语法:
alter table 表名 engine=存储引擎名;
例:先打印目前引擎,在选不同于它的引擎进行更改。
mysql> show create table example \G
*************************** 1. row ***************************
Table: example
Create Table: CREATE TABLE `example` (
`name` char(1) DEFAULT NULL,
`num` int(8) NOT NULL,
`phone` varchar(20) DEFAULT NULL,
`address` varchar(30) NOT NULL,
`age` int(4) NOT NULL,
`sex` tinyint(1) DEFAULT NULL,
PRIMARY KEY (`num`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
1 row in set (0.00 sec)
mysql> alter table example engine=MyISAM;
Query OK, 0 rows affected (0.56 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> show create table example \G
*************************** 1. row ***************************
Table: example
Create Table: CREATE TABLE `example` (
`name` char(1) DEFAULT NULL,
`num` int(8) NOT NULL,
`phone` varchar(20) DEFAULT NULL,
`address` varchar(30) NOT NULL,
`age` int(4) NOT NULL,
`sex` tinyint(1) DEFAULT NULL,
PRIMARY KEY (`num`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
1 row in set (0.00 sec)
删除表的外键约束
语法:
alter table 表名 drop foreign key 外键别名;
外键别名指创建外键时的代号,查看外键别名不能通过desc,只能通过show create table 表名;
例:
mysql> show create table example3 \G
*************************** 1. row ***************************
Table: example3
Create Table: CREATE TABLE `example3` (
`id` int(11) NOT NULL,
`stu_id` int(11) DEFAULT NULL,
`course_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `c_fk` (`stu_id`,`course_id`),
CONSTRAINT `c_fk` FOREIGN KEY (`stu_id`, `course_id`) REFERENCES `example2` (`stu_id`, `course_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
1 row in set (0.00 sec)
mysql> alter table example drop foreign key c_fk;
Query OK, 0 rows affected (0.20 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> show create table example3 \G
*************************** 1. row ***************************
Table: example3
Create Table: CREATE TABLE `example3` (
`id` int(11) NOT NULL,
`stu_id` int(11) DEFAULT NULL,
`course_id` int(11) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `c_fk` (`stu_id`,`course_id`),
CONSTRAINT `c_fk` FOREIGN KEY (`stu_id`, `course_id`) REFERENCES `example2` (`stu_id`, `course_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci
1 row in set (0.00 sec)
mysql>
删除表:
删除表时会删除表中所有数据。由于创建表时存在外键约束,因此删除这些表时要额外注意。
删除没有被关联的普通表:
语法:
drop table 表名;
例:
mysql> show tables;
+-------------------+
| Tables_in_sqldemo |
+-------------------+
| demo |
| demo1 |
| example |
| example1 |
| example2 |
| example3 |
| example4 |
| example5 |
| example6 |
| example7 |
| user |
+-------------------+
11 rows in set (0.00 sec)
mysql> drop table example5;
Query OK, 0 rows affected (0.21 sec)
mysql> show tables;
+-------------------+
| Tables_in_sqldemo |
+-------------------+
| demo |
| demo1 |
| example |
| example1 |
| example2 |
| example3 |
| example4 |
| example6 |
| example7 |
| user |
+-------------------+
10 rows in set (0.00 sec)
删除被其他表关联的表:
如果我们直接删除表是一个父表,那么用普通方法删除会报错。那么有两种处理方法:
一是先删除子表在删除父表,但是这样得不偿失,如果这个子表在是别的表的父表,那么就是一个链式反应。
二是我们直接解除子表对父表的外键依赖。这样就不会影响其它表的数据,可以保证数据库的安全。
- 直接删除会报错,错误提示我们存在子表,并且告诉我们子表和外键别名。
mysql> drop table example1;
ERROR 3730 (HY000): Cannot drop table 'example1' referenced
by a foreign key constraint 'd_fk' on table 'example4'.
- 删除example4外键别名为d_fk的外键约束,并再次删除并打印
ysql> alter table example4 drop foreign key d_fk;
Query OK, 0 rows affected (0.11 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> drop table example1;
Query OK, 0 rows affected (0.20 sec)
mysql> show tables;
+-------------------+
| Tables_in_sqldemo |
+-------------------+
| demo |
| demo1 |
| example |
| example2 |
| example3 |
| example4 |
| example6 |
| example7 |
| user |
+-------------------+
9 rows in set (0.00 sec)