打开微信商户平台的开发文档,下载php版的SDK与Demo
准备好参数:
1:微信公众号AppID,
2:微信公众号AppSecret,
微信公众平台,商户平台添加好项目授权地址等内容
首先获取用户openid(https://blog.csdn.net/u013027894/article/details/81660751)
然后前端传值必要的商品信息(商品名称、价格等)
代码实例:
父级控制器pay.php
<?php
class Pay extends HW_Controller{
const NOT_PAY_STATUS = 2;
const PAY_FINISH_STATUS = 1;
const PAY_CANCEL_STATUS = 0;
public function __construct()
{
header('Access-Control-Allow-Origin:*');
parent::__construct();
$this->load->model(array('Orders_model'));
}
//
private function random($w)
{
$n = current(unpack('L', openssl_random_pseudo_bytes(4)));
return sprintf("%0{$w}.0f", ($n & 0x7fffffff) / 0x7fffffff * (pow(10, $w) - 1));
}
//获取订单
protected function getOrder($trade_no)
{
$where = ['trade_no' => $trade_no];
return $this->Orders_model->get_row($where);
}
//获取订单
protected function getOrderByOut($out_trade_no)
{
$where = ['out_trade_no' => $out_trade_no];
return $this->Orders_model->get_row($where);
}
//付款成功
protected function paySuccess($order)
{
if($order['trade_status'] == 2)
{
$order['trade_status'] = 1;
$this->Orders_model->update($order, ['id' => $order['id']]);
$this->callback($order);
}
}
//组装固定
public function order()
{
$post = $this->input->post();
// $post = array(
// 'u_code'=>547,
// 'operators_id'=>295,
// 'trade_name'=>'微信支付测试',
// 'types'=>1,
// 'out_trade_no'=>'2019050830299131',
// 'trade_no'=>'2019050822001435491030697398',
// 'total_amount'=>'121',
// 'method'=>2,
// 'trade_status'=>1,
// 'subject_id'=>7,
// 'grade_id'=>1,
// 'course_id'=>280,
// 'course_goods_id'=>295,
// );
$order['u_code'] = intval($post['u_code']);
$order['operators_id'] = intval($post['goods_id']) ? intval($post['goods_id']) : 0;
$order['trade_name'] = $post['title'] ? $post['title'] : $post['goods_name'];
$order['types'] = intval($post['types']);
$order['total_amount'] = floatval($post['amount']);
$order['trade_status'] = self::NOT_PAY_STATUS;
$order['trade_no'] = $this->tradeNo();
$order['subject_id'] = intval($post['subject_id']);
$order['grade_id'] = intval($post['grade_id']);
$order['course_id'] = intval($post['course_id']);
$order['course_goods_id'] = intval($post['goods_id']);
return $order;
}
//保存订单
protected function saveOrder($order)
{
try{
$order_id = $this->Orders_model->save($order);
}
catch(Exception $e)
{
return "保存订单失败";
}
return $order_id;
}
//notify回调
public function notify()
{
}
//支付成功后回调
public function callback()
{
}
//获取订单号
private function tradeNo()
{
return md5(uniqid(microtime(true),true));
}
public function http_get($url)
{
$oCurl = curl_init();
if (stripos($url, "https://") !== FALSE) {
curl_setopt($oCurl, CURLOPT_SSL_VERIFYPEER, FALSE);
curl_setopt($oCurl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($oCurl, CURLOPT_SSLVERSION, 1); //CURL_SSLVERSION_TLSv1
}
curl_setopt($oCurl, CURLOPT_URL, $url);
curl_setopt($oCurl, CURLOPT_RETURNTRANSFER, 1);
$sContent = curl_exec($oCurl);
$aStatus = curl_getinfo($oCurl);
curl_close($oCurl);
if (intval($aStatus["http_code"]) == 200) {
return $sContent;
} else {
return false;
}
}
public function curl_request($url, $is_post = 0, $postdata = array())
{
$ch = curl_init();//初始化curl
curl_setopt($ch, CURLOPT_HEADER, false);
curl_setopt($ch, CURLOPT_URL, $url);//抓取指定网页
curl_setopt($ch, CURLOPT_AUTOREFERER, true);
curl_setopt($ch, CURLOPT_REFERER, $url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);//要求结果为字符串且输出到屏幕上
curl_setopt($ch, CURLOPT_BINARYTRANSFER, true);
curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); // https请求 不验证证书和hosts
curl_setopt($ch, CURLOPT_SSL_VERIFYHOST, FALSE);
if ($is_post == 1) {
curl_setopt($ch, CURLOPT_POST, $is_post);//post提交方式
curl_setopt($ch, CURLOPT_POSTFIELDS, $postdata);
}
$data = curl_exec($ch);//运行curl
curl_close($ch);
return $data;
}
}
订单信息公共方法:order
扫描二维码关注公众号,回复:
6745666 查看本文章
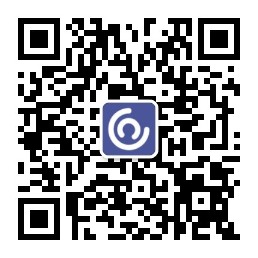
支付控制器WechatPay.php
<?php
require_once APPPATH.'controllers/web/Pay.php';
require_once APPPATH."libraries/WechatPay/notify.php";
require_once APPPATH."libraries/WechatPay/lib/WxPay.Api.php";
require_once APPPATH."libraries/WechatPay/lib/WxPay.Notify.php";
require_once APPPATH."libraries/WechatPay/WxPay.Config.php";
require_once APPPATH."libraries/WechatPay/WxPay.JsApiPay.php";
require_once APPPATH."libraries/WechatPay/log.php";
class WechatPay extends Pay
{
const OAUTH_TOKEN_PREFIX = 'https://api.weixin.qq.com/sns/oauth2';
const OAUTH_TOKEN_URL = '/access_token?';
private $_post = array();
private static $payMethod = 2;
private $appId;
private $appIdMini;
private $appSecret;
private $appSecretMini;
public function __construct()
{
parent::__construct();
$this->_api = $this->load->database('api', TRUE);
$config = new WxPayConfig();
$this->appId = $config->GetAppId();
$this->appIdMini = $config->GetAppIdMini();
$this->appSecret = $config->GetAppSecret();
$this->appSecretMini = $config->GetAppSecretMini();
$this->_post = $this->input->post();
$this->_json = array('code' => self::CODE_FAIL, 'msg' => self::MSG_FAIL, 'data' => array());
}
//下单
public function order()
{
$order = parent::order();
$order['method'] = self::$payMethod;
if(empty($order['u_code']))
{
$this->_json['code'] = self::CODE_FAIL;
$this->_json['msg'] = '无效用户';
$this->y_view($this->_json);
}
$post = $this->input->post();
// $order['out_trade_no'] = date('Ymd') . str_pad(mt_rand(1, 99999999), 8, '0', STR_PAD_LEFT);
// setcookie($post['order_id'], time() + 200);
if($post['is_balance_charge'] == 1 || $post['is_balance_charge'] == '1'){
$data_info['u_code'] = $post['u_code'];
$data_info['balance_describe'] = $post['goods_name'];
$data_info['order_no'] = $order['trade_no'];
$data_info['balance'] = $post['num'];
$data_info['is_deleted'] = 1;
$data_info['is_plus'] = 0;
// $sUrl = MSJT_ZHSSW100.'/Msjtapi/balance_report';
$sUrl = MSJT_ZHSSW100 . '/Msjtapi/balance_report?u_code=' . $data_info['u_code'] . '&balance_describe='
. $post['goods_name'] . '&is_deleted=1&balance=' . $data_info['balance'] . '&types='.$post['types'].'&is_plus=0&order_no=' . $data_info['order_no'];
$res = $this->curl_request($sUrl, 0, $data_info);
$result = json_decode($res,true);
if($result['code'] == 1 || $result['code'] == '1'){
$result == 1;
}else{
$result == '充值下单调接口失败!';
}
}else{
$result = $this->saveOrder($order);
}
if(is_string($result))
{
$this->_json['code'] = self::CODE_FAIL;
$this->_json['msg'] = $result;
$this->y_view($this->_json);
}
$order['product_id'] = $this->_post['grade_id'] . $this->_post['subject_id'] . $this->_post['course_id'] . $this->_post['goods_id'];
$order['total_amount'] = intval(100 * $order['total_amount']) ? intval(100 * $order['total_amount']) : intval(100 * $post['num']) ;
$order['openid'] = $this->_post['openid'];
return $order;
}
public function notify()
{
$this->load->model(array('Orders_model', 'Users_model'));
$post = $this->input->post();
$config = new WxPayConfig();
$notify = new PayNotifyCallBack();
$notify->Handle($config, false);
if(strtoupper($notify->GetReturn_code()) == 'SUCCESS')
{
$xml = isset($GLOBALS['HTTP_RAW_POST_DATA']) ? $GLOBALS['HTTP_RAW_POST_DATA'] : file_get_contents("php://input");
$result = WxPayResults::Init($config,$xml);
$trade_no = $result['out_trade_no'];
$order = $this->getOrder($trade_no);
if(!empty($order))
{
if($order['types'] == 1) {
$data_list['is_msjt_vip'] = 1;
$data_list['u_code'] = $order['u_code'];
$data_list['num_integral'] = intval($order['total_amount']);
$sUrl = $this->config->item('msjt_zhssw100') . '/Msjtapi/user_edit?u_code=' . $data_list['u_code'] . '&is_msjt_vip=1&types=1&num_integral=' . $data_list['num_integral'];
$res = parent::curl_request($sUrl, 0, $data_list);
}
$order['out_trade_no'] = $result['transaction_id'];
$this->paySuccess($order);
}else{
//商户订单号
$data_info['order_no'] = $trade_no;
$data_info['is_plus'] = 0;
$data_info['is_deleted'] = 0;
$sUrl = $this->config->item('msjt_zhssw100') . '/Msjtapi/update_balance?order_no=' . $data_info['order_no'] . '&is_plus=0&is_deleted=0';
$result = $this->curl_request($sUrl, 0, $data_info);
$info = json_decode($result,true);
$this->_json['code'] = $info['code'];
$this->_json['msg'] = $info['msg'];
$this->_json['data'] = $info['data'];
$this->y_view($this->_json);
}
}
}
//组装订单获取支付链接
public function create()
{
$order = $this->order();
$this->load->library('WechatPay/NativePay', '', 'native');
$input = new WxPayUnifiedOrder();
$input->SetBody($order['trade_name']);
$input->SetOut_trade_no($order['trade_no']);
$input->SetTotal_fee($order['total_amount']);
$input->SetTime_start(date("YmdHis"));
$input->SetNotify_url("https://msktapi.zhssw.com/wechatPay/notify");
$input->SetTrade_type("NATIVE");
$input->SetProduct_id($order['product_id']);
$result = $this->native->GetPayUrl($input);
$data['url'] = urlencode($result["code_url"]);
$data['trade_no'] = $order['trade_no'];
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $data;
$this->y_view($this->_json);
}
//回调成功
public function callback()
{
}
/**
* 微信JSAPI支付统一下单
* @Author TGHan
* @DateTime 2019-05-14
* @version [version]
* @return [type] [description]
*/
public function jsapi()
{
$post = $this->input->post();
$order = $this->order();
$tools = new JsApiPay();
//②、统一下单
$input = new WxPayUnifiedOrder();
$input->SetBody($order['trade_name']);
$input->SetOut_trade_no($order['trade_no']);
$input->SetTotal_fee($order['total_amount']);
$input->SetTime_start(date("YmdHis"));
$input->SetNotify_url('https://msktapi.zhssw.com/wechatPay/notify');
$input->SetTrade_type("JSAPI");
$input->SetOpenid($order['openid']);
$config = new WxPayConfig();
$orders = WxPayApi::unifiedOrder($config, $input);
$jsApiParameters = $tools->GetJsApiParameters($orders);
$data_info = json_decode($jsApiParameters,true);
$jsapiTicket = $this->getJsApiTicket();
$nonceStr = $data_info['nonceStr'];
$timestamp = $data_info['timeStamp'];
$url = $post['url'];
$string = "jsapi_ticket=$jsapiTicket&noncestr=$nonceStr×tamp=$timestamp&url=$url";
$signature['signature'] = sha1($string);
$data_list = array_merge($data_info,$signature);
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $data_list;
$this->y_view($this->_json);
}
/**
* 给客户端返回必要微信参数
* @Author TGHan
* @DateTime 2018-04-26
* @return [type] [description]
*/
public function modifyinfor() {
$post = $this->input->post();
$jsapiTicket = $this->getJsApiTicket();
// 注意 URL 一定要动态获取,不能 hardcode.
// $protocol = (!empty($_SERVER['HTTPS']) && $_SERVER['HTTPS'] !== 'off' || $_SERVER['SERVER_PORT'] == 443) ? "https://" : "http://";
// $url = "$protocol$_SERVER[HTTP_HOST]$_SERVER[REQUEST_URI]";
$url = $post['url'];
$timestamp = time();
$nonceStr = $this->createNonceStr();
// 这里参数的顺序要按照 key 值 ASCII 码升序排序
$string = "jsapi_ticket=$jsapiTicket&noncestr=$nonceStr×tamp=$timestamp&url=$url";
$signature = sha1($string);
$signPackage = array(
"appId" => $this->appId,
"nonceStr" => $nonceStr,
"timestamp" => $timestamp,
"url" => $url,
"signature" => $signature,
"rawString" => $string
);
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $signPackage;
$this->y_view($this->_json);
}
public function createNonceStr($length = 16) {
$chars ="abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
$str = "";
for ($i = 0; $i < $length; $i++) {
$str .= substr($chars, mt_rand(0, strlen($chars) - 1), 1);
}
return $str;
}
public function getJsApiTicket() {
// jsapi_ticket 应该全局存储与更新,以下代码以写入到文件中做示例
$data =json_decode(file_get_contents("jsapi_ticket.json"));
if ($data->expire_time < time()) {
$accessToken = $this->getAccessToken();
// 如果是企业号用以下 URL 获取 ticket
// $url = "https://qyapi.weixin.qq.com/cgi-bin/get_jsapi_ticket?access_token=$accessToken";
$url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?type=jsapi&access_token=$accessToken";
$res = json_decode($this->httpGet($url));
$ticket = $res->ticket;
if ($ticket) {
$data->expire_time = time() + 7000;
$data->jsapi_ticket = $ticket;
$fp = fopen("jsapi_ticket.json", "w");
fwrite($fp, json_encode($data));
fclose($fp);
}
} else {
$ticket = $data->jsapi_ticket;
}
return $ticket;
}
public function getAccessToken() {
// access_token 应该全局存储与更新,以下代码以写入到文件中做示例
$data =json_decode(file_get_contents("access_token.json"));
if ($data->expire_time < time()) {
// 如果是企业号用以下URL获取access_token
// $url = "https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=$this->appId&corpsecret=$this->appSecret";
$url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=$this->appId&secret=$this->appSecret";
$res = json_decode($this->httpGet($url));
$access_token = $res->access_token;
if ($access_token) {
$data->expire_time = time() + 7000;
$data->access_token = $access_token;
$fp = fopen("access_token.json", "w");
fwrite($fp, json_encode($data));
fclose($fp);
}
} else {
$access_token = $data->access_token;
}
return $access_token;
}
public function getJsApiTicketMini() {
// jsapi_ticket 应该全局存储与更新,以下代码以写入到文件中做示例
$data =json_decode(file_get_contents("jsapi_ticket.json"));
if ($data->expire_time < time()) {
$accessToken = $this->getAccessTokenMini();
// 如果是企业号用以下 URL 获取 ticket
// $url = "https://qyapi.weixin.qq.com/cgi-bin/get_jsapi_ticket?access_token=$accessToken";
$url = "https://api.weixin.qq.com/cgi-bin/ticket/getticket?type=jsapi&access_token=$accessToken";
$res = json_decode($this->httpGet($url));
$ticket = $res->ticket;
if ($ticket) {
$data->expire_time = time() + 7000;
$data->jsapi_ticket = $ticket;
$fp = fopen("jsapi_ticket.json", "w");
fwrite($fp, json_encode($data));
fclose($fp);
}
} else {
$ticket = $data->jsapi_ticket;
}
return $ticket;
}
public function getAccessTokenMini() {
// access_token 应该全局存储与更新,以下代码以写入到文件中做示例
$data =json_decode(file_get_contents("access_token.json"));
if ($data->expire_time < time()) {
// 如果是企业号用以下URL获取access_token
// $url = "https://qyapi.weixin.qq.com/cgi-bin/gettoken?corpid=$this->appId&corpsecret=$this->appSecret";
$url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=$this->appIdMini&secret=$this->appSecretMini";
$res = json_decode($this->httpGet($url));
$access_token = $res->access_token;
if ($access_token) {
$data->expire_time = time() + 7000;
$data->access_token = $access_token;
$fp = fopen("access_token.json", "w");
fwrite($fp, json_encode($data));
fclose($fp);
}
} else {
$access_token = $data->access_token;
}
return $access_token;
}
public function httpGet($url) {
$curl = curl_init();
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_TIMEOUT, 500);
// 为保证第三方服务器与微信服务器之间数据传输的安全性,所有微信接口采用https方式调用,必须使用下面2行代码打开ssl安全校验。
// 如果在部署过程中代码在此处验证失败,请到 http://curl.haxx.se/ca/cacert.pem 下载新的证书判别文件。
curl_setopt($curl, CURLOPT_SSL_VERIFYPEER, FALSE); // https请求 不验证证书和hosts
curl_setopt($curl, CURLOPT_SSL_VERIFYHOST, FALSE);
curl_setopt($curl, CURLOPT_URL, $url);
$res = curl_exec($curl);
curl_close($curl);
return $res;
}
public function getOpenId() {
$post = $this->input->post();
$code = $post['code'];
$url = "https://api.weixin.qq.com/sns/jscode2session?appid=$this->appIdMini&secret=$this->appSecretMini&js_code=$code&grant_type=authorization_code";
// $url = 'https://api.weixin.qq.com/sns/jscode2session?appid=wx6330ec3336582141&secret=cd67e10ce2638c3448e513237c7f491e&js_code=0616v5H32ruaCP0gCIF32TrKG326v5H0&grant_type=authorization_code';
$result = parent::http_get($url);
$data_info = json_decode($result, true);
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $data_info;
$this->y_view($this->_json);
}
public function jsapiMini()
{
$post = $this->input->post();
$order = $this->order();
$tools = new JsApiPay();
//②、统一下单
$input = new WxPayUnifiedOrder();
$input->SetBody($order['trade_name']);
$input->SetOut_trade_no($order['trade_no']);
$input->SetTotal_fee($order['total_amount']);
$input->SetTime_start(date("YmdHis"));
$input->SetNotify_url('https://msktapi.zhssw.com/wechatPay/notify');
$input->SetTrade_type("JSAPI");
$input->SetOpenid($post['openid']);
$config = new WxPayConfig();
$orders = WxPayApi::unifiedOrderMini($config, $input);
$jsApiParameters = $tools->GetJsApiParameters($orders);
$data_info = json_decode($jsApiParameters,true);
$jsapiTicket = $this->getJsApiTicketMini();
$nonceStr = $data_info['nonceStr'];
$timestamp = $data_info['timeStamp'];
$url = $post['url'];
$string = "jsapi_ticket=$jsapiTicket&noncestr=$nonceStr×tamp=$timestamp&url=$url";
$signature['signature'] = sha1($string);
$data_list = array_merge($data_info,$signature);
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $data_list;
$this->y_view($this->_json);
}
/*todo 微信网页支付用的到的*/
public function pagePay(){
header('Access-Control-Allow-Origin:*');
$get = $_GET;
if (empty($get['u_code'])) {
$get = $_POST;
}
$num = $get['num'];
$get['num'] = floatval($get['num'])*100;
$get['order_id'] = md5(uniqid(microtime(true),true));
// setcookie($get['order_id'], $get['describe'], time() + 200);
if ($get['is_balance_charge'] == 1) {
$order['u_code'] = $get['u_code'];
$order['balance_describe'] = $get['goods_name'];
$order['order_no'] = $get['order_id'];
$order['balance'] = $get['num'];
$order['is_deleted'] = 1;
$order['is_plus'] = 0;
$sUrl = MSJT_ZHSSW100 . '/Msjtapi/balance_report?u_code=' . $order['u_code'] . '&balance_describe='
. $get['goods_name'] . '&is_deleted=1&balance=' . $num . '&types=' . $get['types'] . '&is_plus=0&order_no=' . $order['order_no'];
$result = $this->curl_request($sUrl, 0, $order);
} else {
$order['u_code'] = $get['u_code'];
$order['trade_name'] = $get['goods_name'];
$order['operators_id'] = intval($get['goods_id']);
$order['out_trade_no'] = $get['order_id'];
$order['total_amount'] = $get['num'];
$order['types'] = $get['types'];
$order['education_order_describe'] = $get['describe'];
$order['education_order_describe'] .= $get['is_report'] ? '(成功送积分)' : '';
$order['education_price_id'] = intval($get['goods_id']);
$order['method'] = 2;
$order['trade_status'] = 2;
$order['subject_id'] = intval($get['subject_id']);
$order['grade_id'] = intval($get['grade_id']);
$order['course_id'] = intval($get['course_id']);
$this->load->model(array('Orders_model'));
$this->Orders_model->save($order);
}
$order1['trade_name'] = $get['title'] ? $get['title'] : $get['goods_name'];
$order1['total_amount'] = $get['num'];
$order1['trade_no'] =$get['order_id'];
// $order1['u_code'] = intval($get['u_code']);
// $order1['types'] = intval($get['types']);
// $order1['trade_status'] = self::NOT_PAY_STATUS;
// $order1['subject_id'] = intval($get['subject_id']);
// $order1['grade_id'] = intval($get['grade_id']);
// $order1['course_id'] = intval($get['course_id']);
// $order1['course_goods_id'] = intval($get['goods_id']);
$this->load->library('WechatPay/NativePay', '', 'native');
$input = new WxPayUnifiedOrder();
$input->SetBody($order1['trade_name']);
$input->SetOut_trade_no($order1['trade_no']);
$input->SetTotal_fee($order1['total_amount']);
$input->SetTime_start(date("YmdHis"));
$input->SetNotify_url("https://msktapi.zhssw.com/wechatPay/notify");
$input->SetTrade_type("NATIVE");
$input->SetProduct_id($order1['trade_no']);
$result1 = $this->native->GetPayUrl($input);
$this->load->library('WechatPay/QrcodeImg', '', 'qrcode');
$data['weixin_url'] = $result1["code_url"];
$data['trade_no'] = $order1['trade_no'];
$data['result'] = $order1;
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = self::MSG_SUCC;
$this->_json['data'] = $data;
$this->y_view($this->_json);
}
// 根据订单查询pc端充值结果
public function orderCheck()
{
$trade_no = $_POST['trade_no'] ? $_POST['trade_no'] : $_GET['trade_no'];
if(empty($trade_no)){
$this->_json['code'] = self::CODE_FAIL;
$this->_json['msg'] = '请传递交易号码';
$this->y_view($this->_json);
}
$sql1 = "SELECT * FROM api.h_balance_detail where order_no = '" . $trade_no . "'";
$user = $this->_api->query($sql1)->row();
if($user->is_deleted == 0 && !empty($user)){
$this->_json['code'] = self::CODE_SUCC;
$this->_json['msg'] = '充值成功';
$this->_json['data'] = [];
$this->y_view($this->_json);
}
$this->_json['code'] = self::CODE_FAIL;
$this->_json['msg'] = '充值失败';
$this->_json['data'] = [];
$this->y_view($this->_json);
}
//获取二维码
public function qrcode()
{
$url = $_GET["data"];
$this->load->library('WechatPay/QrcodeImg', '', 'qrcode');
$this->qrcode->getImage($url);
}
}
统一下单方法:jsapi
以及回调方法:
订单信息入库:order
前后端联调。
参考文档:
https://blog.csdn.net/weixin_33699914/article/details/87606809
https://blog.csdn.net/Honnyee/article/details/79259304
https://blog.csdn.net/zhuwcang830512/article/details/82918152
https://www.jb51.net/article/136466.htm