The caching options available in ASP.NET MVC applications don’t come from the ASP.NET MVC Framework, but from the core ASP.NET Framework.
1. Request-Scoped Caching
Every ASP.NET request begins with the ASP.NET Framework creating a new instance
of the System.Web.HttpContext object to act as the central point of interaction between
components throughout the request.
One of the many properties of the HttpContext is the HttpContext.Items property, a
dictionary that lives throughout the lifetime of the request and which any component
may manipulate.
eg:
// how to store data in the collection: HttpContext.Items["IsFirstTimeUser"] = true; // Retrieving data from the dictionary is just as easy: bool IsFirstTimeUser = (bool)HttpContext.Items["IsFirstTimeUser"];
2. User-Scoped Caching
ASP.NET session state allows you to store data that persists between multiple requests.
// store the username in a session HttpContext.Session["username"] = "Hrusi"; // retrieve and cast the untyped value: string name = (string)HttpContext.Session["username"];
<system.web>
<sessionState timeout="30" />
</system.web>
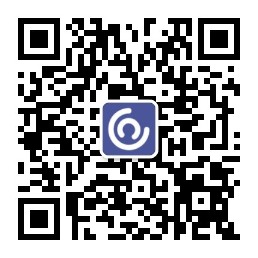
3. The ASP.NET Cache
System.Web.Cache is a key/value store
4. The Output Cache
ASP.NET provides the ability to operate at a higher level, caching the HTML that is generated as a result of a request.
[OutputCache(Duration=60, VaryByParam="none")] public ActionResult Contact() { ViewBag.Message = DateTime.Now.ToString(); return View(); }
Configuring the cache location
For example, say you want to cache a page that displays the current user’s name. If you
use the default Any setting, the name of the first person to request the page will incorrectly
be displayed to all users.
To avoid this, configure the output cache with the Location property set to Output
CacheLocation.Client and NoStore set to true so that the data is stored only in the user’s
local web browser:
[OutputCache(Duration = 3600, VaryByParam = "none", Location = OutputCacheLocation.Client, NoStore = true)] public ActionResult About() { ViewBag.Message = "The current user name is " + User.Identity.Name; return View(); }
Varying the output cache based on request parameters
For example, say you have a controller action named Details that displays the details
of an auction:
[OutputCache(Duration = int.MaxValue, VaryByParam = "id")] public ActionResult Details(string id) { var auction = _repository.Find<Auction>(id); return View("Details", auction); }
转载于:https://www.cnblogs.com/davidgu/p/3331699.html