To start, we'll create a progress bar that just lets us set the progress once. As we can see below, this is done by calling jQuery.widget()
with two parameters: the name of the plugin to create, and an object literal containing functions to support our plugin. When our plugin gets called, it will create a new plugin instance and all functions will be executed within the context of that instance. This is different from a standard jQuery plugin in two important ways. First, the context is an object, not a DOM element. Second, the context is always a single object, never a collection.
$.widget( "custom.progressbar", { _create: function() { var progress = this.options.value + "%"; this.element .addClass( "progressbar" ) .text( progress ); } });
The name of the plugin must contain a namespace, in this case we've used the custom
namespace.
You can only create namespaces that are one level deep, therefore, custom.progressbar
is a valid plugin name whereas very.custom.progressbar
is not.
We can also see that the widget factory has provided two properties for us.
this.element
is a jQuery object containing exactly one element. If our plugin is called on a jQuery object containing multiple elements, a separate plugin instance will be created for each element, and each instance will have its own this.element
.
The second property, this.options
, is a hash containing key/value pairs for all of our plugin's options. These options can be passed to our plugin as shown here.
$( "<div></div>" ) .appendTo( "body" ) .progressbar({ value: 20 });
When we call jQuery.widget()
it extends jQuery by adding a function to jQuery.fn
(the system for creating a standard plugin).
The name of the function it adds is based on the name you pass to jQuery.widget()
, without the namespace - in our case "progressbar". The options passed to our plugin are the values that get set in this.options
inside of our plugin instance. As shown below, we can specify default values for any of our options. When designing your API, you should figure out the most common use case for your plugin so that you can set appropriate default values and make all options truly optional.
$.widget( "custom.progressbar", { // Default options. options: { value: 0 }, _create: function() { var progress = this.options.value + "%"; this.element .addClass( "progressbar" ) .text( progress ); } });
Calling Plugin Methods
Now that we can initialize our progress bar, we'll add the ability to perform actions by calling methods on our plugin instance. To define a plugin method, we just include the function in the object literal that we pass to jQuery.widget()
. We can also define "private" methods by prepending an underscore to the function name.
$.widget( "custom.progressbar", { options: { value: 0 }, _create: function() { var progress = this.options.value + "%"; this.element .addClass( "progressbar" ) .text( progress ); }, // Create a public method. value: function( value ) { // No value passed, act as a getter. if ( value === undefined ) { return this.options.value; } // Value passed, act as a setter. this.options.value = this._constrain( value ); var progress = this.options.value + "%"; this.element.text( progress ); }, // Create a private method. _constrain: function( value ) { if ( value > 100 ) { value = 100; } if ( value < 0 ) { value = 0; } return value; } });
To call a method on a plugin instance, you pass the name of the method to the jQuery plugin. If you are calling a method that accepts parameters, you simply pass those parameters after the method name.
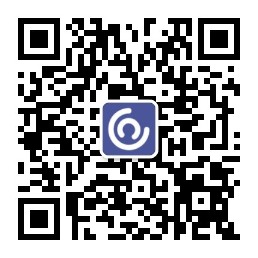
How To Use the Widget Factory
To start, we'll create a progress bar that just lets us set the progress once. As we can see below, this is done by calling jQuery.widget()
with two parameters: the name of the plugin to create, and an object literal containing functions to support our plugin. When our plugin gets called, it will create a new plugin instance and all functions will be executed within the context of that instance. This is different from a standard jQuery plugin in two important ways. First, the context is an object, not a DOM element. Second, the context is always a single object, never a collection.
1
2
3
4
5
6
7
8
|
$.widget( "custom.progressbar", {
_create: function() {
var progress = this.options.value + "%";
this.element
.addClass( "progressbar" )
.text( progress );
}
});
|
The name of the plugin must contain a namespace, in this case we've used the custom
namespace. You can only create namespaces that are one level deep, therefore, custom.progressbar
is a valid plugin name whereas very.custom.progressbar
is not.
We can also see that the widget factory has provided two properties for us. this.element
is a jQuery object containing exactly one element. If our plugin is called on a jQuery object containing multiple elements, a separate plugin instance will be created for each element, and each instance will have its own this.element
. The second property, this.options
, is a hash containing key/value pairs for all of our plugin's options. These options can be passed to our plugin as shown here.
1
2
3
|
$( "<div></div>" )
.appendTo( "body" )
.progressbar({ value: 20 });
|
When we call jQuery.widget()
it extends jQuery by adding a function to jQuery.fn
(the system for creating a standard plugin). The name of the function it adds is based on the name you pass to jQuery.widget()
, without the namespace - in our case "progressbar". The options passed to our plugin are the values that get set in this.options
inside of our plugin instance. As shown below, we can specify default values for any of our options. When designing your API, you should figure out the most common use case for your plugin so that you can set appropriate default values and make all options truly optional.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
$.widget( "custom.progressbar", {
// Default options.
options: {
value: 0
},
_create: function() {
var progress = this.options.value + "%";
this.element
.addClass( "progressbar" )
.text( progress );
}
});
|
link Calling Plugin Methods
Now that we can initialize our progress bar, we'll add the ability to perform actions by calling methods on our plugin instance. To define a plugin method, we just include the function in the object literal that we pass to jQuery.widget()
. We can also define "private" methods by prepending an underscore to the function name.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
|
$.widget( "custom.progressbar", {
options: {
value: 0
},
_create: function() {
var progress = this.options.value + "%";
this.element
.addClass( "progressbar" )
.text( progress );
},
// Create a public method.
value: function( value ) {
// No value passed, act as a getter.
if ( value === undefined ) {
return this.options.value;
}
// Value passed, act as a setter.
this.options.value = this._constrain( value );
var progress = this.options.value + "%";
this.element.text( progress );
},
// Create a private method.
_constrain: function( value ) {
if ( value > 100 ) {
value = 100;
}
if ( value < 0 ) {
value = 0;
}
return value;
}
});
|
To call a method on a plugin instance, you pass the name of the method to the jQuery plugin. If you are calling a method that accepts parameters, you simply pass those parameters after the method name.
Note: Executing methods by passing the method name to the same jQuery function that was used to initialize the plugin may seem odd. This is done to prevent pollution of the jQuery namespace while maintaining the ability to chain method calls. Later in this article we'll see alternative uses that may feel more natural.
var bar = $( "<div></div>" ) .appendTo( "body" ) .progressbar({ value: 20 }); // Get the current value. alert( bar.progressbar( "value" ) ); // Update the value. bar.progressbar( "value", 50 ); // Get the current value again. alert( bar.progressbar( "value" ) );