注:文章转自:
http://lavasoft.blog.51cto.com/62575/240268/
一、定义IDL
module HelloApp{
interface Hello{
string sayHello();
oneway void shutdown();
};
};
interface Hello{
string sayHello();
oneway void shutdown();
};
};
没有IDL的专门的开发工具,写起来容易出错,而且不美观,IDEA勉强支持语法高亮,但不支持格式化和语法校验!
二、从IDL生成存根
进入IDL文件存放目录,然后执行:idlj -fall Hello.idl
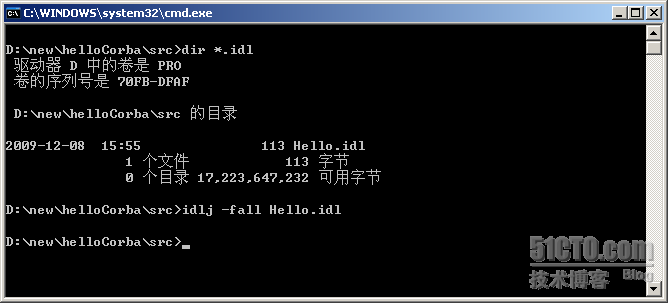
会生成一批Java文件:
helloCorba\src\HelloApp
HelloPOA.java
_HelloStub.java
HelloHolder.java
HelloHelper.java
Hello.java
HelloOperations.java
_HelloStub.java
HelloHolder.java
HelloHelper.java
Hello.java
HelloOperations.java
三、实现IDL接口,开发服务端程序
import HelloApp.Hello;
import HelloApp.HelloHelper;
import HelloApp.HelloPOA;
import org.omg.CORBA.ORB;
import org.omg.CosNaming.NameComponent;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
import org.omg.PortableServer.POA;
import org.omg.PortableServer.POAHelper;
class HelloImpl extends HelloPOA {
private ORB orb;
public void setORB(ORB orb_val) {
orb = orb_val;
}
// implement sayHello() method
public String sayHello() {
return "\nHello world !!\n";
}
// implement shutdown() method
public void shutdown() {
orb.shutdown( false);
}
}
public class HelloServer {
public static void main(String args[]) {
try {
//创建一个ORB实例
ORB orb = ORB.init(args, null);
//得到一个RootPOA的引用,并激活POAManager
POA rootpoa = POAHelper.narrow(orb.resolve_initial_references( "RootPOA"));
rootpoa.the_POAManager().activate();
// create servant and register it with the ORB
//创建一个HelloImpl实例(servant),并注册到ORB上
HelloImpl helloImpl = new HelloImpl();
helloImpl.setORB(orb);
//从服务中得到对象的引用
org.omg.CORBA.Object ref = rootpoa.servant_to_reference(helloImpl);
Hello href = HelloHelper.narrow(ref);
//得到一个根名称的上下文
org.omg.CORBA.Object objRef = orb.resolve_initial_references( "NameService");
// Use NamingContextExt which is part of the Interoperable
// Naming Service (INS) specification.
NamingContextExt ncRef = NamingContextExtHelper.narrow(objRef);
//在命名上下文中绑定这个对象
String name = "Hello";
NameComponent path[] = ncRef.to_name(name);
ncRef.rebind(path, href);
System.out.println( "HelloServer ready and waiting ...");
//启动线程服务,等待客户端的调用
orb.run();
} catch (Exception e) {
System.err.println( "ERROR: " + e);
e.printStackTrace(System.out);
}
System.out.println( "HelloServer Exiting ...");
}
}
import HelloApp.HelloHelper;
import HelloApp.HelloPOA;
import org.omg.CORBA.ORB;
import org.omg.CosNaming.NameComponent;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
import org.omg.PortableServer.POA;
import org.omg.PortableServer.POAHelper;
class HelloImpl extends HelloPOA {
private ORB orb;
public void setORB(ORB orb_val) {
orb = orb_val;
}
// implement sayHello() method
public String sayHello() {
return "\nHello world !!\n";
}
// implement shutdown() method
public void shutdown() {
orb.shutdown( false);
}
}
public class HelloServer {
public static void main(String args[]) {
try {
//创建一个ORB实例
ORB orb = ORB.init(args, null);
//得到一个RootPOA的引用,并激活POAManager
POA rootpoa = POAHelper.narrow(orb.resolve_initial_references( "RootPOA"));
rootpoa.the_POAManager().activate();
// create servant and register it with the ORB
//创建一个HelloImpl实例(servant),并注册到ORB上
HelloImpl helloImpl = new HelloImpl();
helloImpl.setORB(orb);
//从服务中得到对象的引用
org.omg.CORBA.Object ref = rootpoa.servant_to_reference(helloImpl);
Hello href = HelloHelper.narrow(ref);
//得到一个根名称的上下文
org.omg.CORBA.Object objRef = orb.resolve_initial_references( "NameService");
// Use NamingContextExt which is part of the Interoperable
// Naming Service (INS) specification.
NamingContextExt ncRef = NamingContextExtHelper.narrow(objRef);
//在命名上下文中绑定这个对象
String name = "Hello";
NameComponent path[] = ncRef.to_name(name);
ncRef.rebind(path, href);
System.out.println( "HelloServer ready and waiting ...");
//启动线程服务,等待客户端的调用
orb.run();
} catch (Exception e) {
System.err.println( "ERROR: " + e);
e.printStackTrace(System.out);
}
System.out.println( "HelloServer Exiting ...");
}
}
四、实现CORBA的客户端
import HelloApp.Hello;
import HelloApp.HelloHelper;
import org.omg.CORBA.ORB;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
public class HelloClient {
static Hello helloImpl;
public static void main(String args[]) {
try {
//创建一个ORB实例
ORB orb = ORB.init(args, null);
//获取根名称上下文
org.omg.CORBA.Object objRef = orb.resolve_initial_references( "NameService");
// Use NamingContextExt instead of NamingContext. This is
// part of the Interoperable naming Service.
NamingContextExt ncRef = NamingContextExtHelper.narrow(objRef);
//从命名上下文中获取接口实现对象
String name = "Hello";
helloImpl = HelloHelper.narrow(ncRef.resolve_str(name));
//调用接口对象的方法
System.out.println( "Obtained a handle on server object: " + helloImpl);
System.out.println(helloImpl.sayHello());
helloImpl.shutdown();
} catch (Exception e) {
System.out.println( "ERROR : " + e);
e.printStackTrace(System.out);
}
}
import HelloApp.HelloHelper;
import org.omg.CORBA.ORB;
import org.omg.CosNaming.NamingContextExt;
import org.omg.CosNaming.NamingContextExtHelper;
public class HelloClient {
static Hello helloImpl;
public static void main(String args[]) {
try {
//创建一个ORB实例
ORB orb = ORB.init(args, null);
//获取根名称上下文
org.omg.CORBA.Object objRef = orb.resolve_initial_references( "NameService");
// Use NamingContextExt instead of NamingContext. This is
// part of the Interoperable naming Service.
NamingContextExt ncRef = NamingContextExtHelper.narrow(objRef);
//从命名上下文中获取接口实现对象
String name = "Hello";
helloImpl = HelloHelper.narrow(ncRef.resolve_str(name));
//调用接口对象的方法
System.out.println( "Obtained a handle on server object: " + helloImpl);
System.out.println(helloImpl.sayHello());
helloImpl.shutdown();
} catch (Exception e) {
System.out.println( "ERROR : " + e);
e.printStackTrace(System.out);
}
}
}
五、运行
1)运行CORBA服务
orbd -ORBInitialPort 1050 -ORBInitialHost 192.168.14.117
2)运行CORBA应用的服务端
java HelloServer -ORBInitialPort 1050
输出:
HelloServer ready and waiting ...
HelloServer Exiting ...
HelloServer Exiting ...
3)运行CORBA应用的客户端
java HelloClient -ORBInitialHost 192.168.14.117 -ORBInitialPort 1050
Obtained a handle on server object: IOR:000000000000001749444c3a48656c6c6f4170702f48656c6c6f3a312e30000000000001000000000000008a000102000000000f3139322e3136382e31342e313137000006db000000000031afabcb00000000206d3bb80000000001000000000000000100000008526f6f74504f410000000008000000010000000014000000000000020000000100000020000000000001000100000002050100010001002000010109000000010001010000000026000000020002
Hello world !!
Hello world !!
orbd.exe
用法:orbd <选项>
其中,<选项> 包括:
-port 启动 ORBD 的激活端口,缺省值为 1049 (可选)
-defaultdb ORBD 文件的目录,缺省值为 "./orb.db" (可选)
-serverid ORBD 的服务器标识符,缺省值为 1 (可选)
-ORBInitialPort 初始端口(必需)
-ORBInitialHost 初始主机名称(必需)
转载于:https://my.oschina.net/u/2552902/blog/543828