1、准备工作
1): 在pom.xml添加需要的依赖
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.5</version>
</dependency>
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.2</version>
</dependency>
2):在spring-mvc.xml配置文件解析器
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="defaultEncoding" value="utf-8"/>
<property name="maxUploadSize" value="52428800"/>
</bean>
2、单文件上传
1):后台代码
@RequestMapping(value = "/upload", method = RequestMethod.POST, produces = "text/html;charset=utf-8")
public Object upload(HttpSession session, @RequestParam("uploadFile") MultipartFile file) {
boolean bool = false;
ServletContext context = session.getServletContext();
String realPath = context.getRealPath("/upload");
String fileName = UUID.randomUUID().toString().replace("-", "").substring(0, 15) + "_file_" + file.getOriginalFilename();
try {
file.transferTo(new File(realPath + "/" + fileName));
bool=true;
} catch (IOException e) {
e.printStackTrace();
}
return bool ? "success" : "fail";
}
2):前端代码
- 要上传的文件表单、enctype=“multipart/form-data”,属性必不可少
<form role="form" id="saveUploadForm" enctype="multipart/form-data">
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="uploadFile" id="uploadFile">
</div>
</form>
$.ajax({
url: "${path }/upload",
type: "POST",
data: new FormData($("#saveUploadForm")[0]),
processData: false,
contentType: false,
success: function (result) {
layer.msg(result);
},
error: function (e) {
layer.msg(e);
}
});
3、多文件上传
1):后台代码
private String uploadFile(String webPath, MultipartFile file, HttpSession session) {
ServletContext context = session.getServletContext();
String realPath = context.getRealPath(webPath);
String fileName = UUID.randomUUID().toString().replace("-", "").substring(0, 15) + "_file_" + file.getOriginalFilename();
try {
File file1 = new File(realPath);
if (!file1.exists()) {
file1.mkdirs();
}
file.transferTo(new File(realPath + "/" + fileName));
return webPath + "/" + fileName;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
@RequestMapping("/upload", method = RequestMethod.POST, produces = "text/html;charset=utf-8")
public Object upload(@RequestParam("file") MultipartFile[] file, HttpSession session) {
for (int i = 0; i < file.length; i++) {
MultipartFile multipartFile = file[i];
String uploadFilePath = uploadFile("/upload", multipartFile, session);
System.out.println(uploadFilePath );
}
return "success";
}
2):前端代码
- 要提交的文件表单、enctype=“multipart/form-data”,属性必不可少
<form role="form" id="saveFileForm" enctype="multipart/form-data">
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="file">
</div>
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="file">
</div>
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="file">
</div>
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="file">
</div>
<div class="form-group">
<label>选择文件</label>
<input type="file" class="form-control" name="file">
</div>
</form>
$.ajax({
url: "${path }/upload",
type: "POST",
data: new FormData($("#saveFileForm")[0]),
processData: false,
contentType: false,
success: function (result) {
layer.msg(result);
},
error: function (e) {
layer.msg(e);
}
});
3):文件上传带进度条
<!DOCTYPE html>
<html>
<head>
<title>Title</title>
</head>
<script type="text/javascript" src="${path}/static/plugin/jquery/jquery-2.1.1.min.js"></script>
<script type="text/javascript" src="${path}/static/plugin/bootstrap-3.3.7/js/bootstrap.min.js"></script>
<script type="text/javascript" src="${path}/static/plugin/layer-v3.1.1/layer/layer.js"></script>
<link rel="stylesheet" href="static/plugin/bootstrap-3.3.7/css/bootstrap.min.css">
<body>
<div class="container">
<div class="row">
<div class="col-sm-4"></div>
<div class="col-sm-4">
<form id="fileForm" enctype="multipart/form-data">
<label>请选择文件:</label><input type="file" name="file">
</form>
<button id="submitBtn">上传</button>
<br/>
<div class="progress">
<div class="progress-bar" role="progressbar"
style="width: 0%;">
</div>
</div>
</div>
<div class="col-sm-4"></div>
</div>
</div>
<script type="text/javascript">
$("#submitBtn").click(function () {
$.ajax({
url: "${path}/upload",
data: new FormData($("#fileForm")[0]),
processData: false,
contentType: false,
type: "POST",
dataType: "text",
xhr: function () {
var xhr = $.ajaxSettings.xhr();
xhr.upload.onprogress = function (ev) {
console.log("已经上传:" + ev.loaded);
console.log("总计:" + ev.total);
console.log("当前进度:" + (ev.loaded / ev.total) * 100 + "%");
var procent = ((ev.loaded / ev.total) * 100).toFixed(2);
$(".progress-bar").css('width', procent + "%").text(procent + "%");
};
return xhr;
},
success: function (result) {
layer.msg(result);
$("#fileForm").reset;
}
});
});
</script>
</body>
</html>
- 效果图
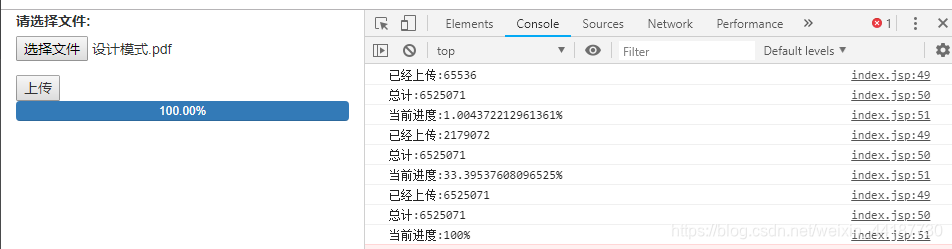