Mainly taken from official documentation:
The only difference with a regular Session is that an InteractiveSession installs itself as the default session on construction. The methods Tensor.eval() and Operation.run() will use that session to run ops.
This allows to use interactive context, like shell, as it avoids having to pass an explicit Session object to run op:
sess = tf.InteractiveSession()
a = tf.constant(5.0)
b = tf.constant(6.0)
c = a * b
# We can just use 'c.eval()' without passing 'sess'
print(c.eval())
sess.close()
It is also possible to say, that InteractiveSession
supports less typing, as allows to run variables without needing to constantly refer to the session object.
tf.InteractiveSession()加载它自身作为默认构建的session,tensor.eval()和operation.run()取决于默认的session。
tf.InteractiveSession()输入的代码少,原因就是它允许变量不需要使用session就可以产生结构。
tf.InteractiveSession():它能让你在运行图的时候,插入一些计算图,这些计算图是由某些操作(operations)构成的。这对于工作在交互式环境中的人们来说非常便利,比如使用IPython。
tf.Session():需要在启动session之前构建整个计算图,然后启动该计算图。
意思就是在我们使用tf.InteractiveSession()来构建会话的时候,我们可以先构建一个session然后再定义操作(operation),如果我们使用tf.Session()来构建会话我们需要在会话构建之前定义好全部的操作(operation)然后再构建会话。
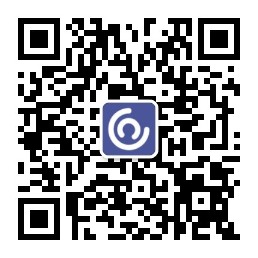
下面这三个是等价的:
sess = tf.InteractiveSession()
sess = tf.Session()
with sess.as_default():
with tf.Session() as sess:
示例:
import tensorflow as tf
import numpy as np
a=tf.constant([[1., 2., 3.],[4., 5., 6.]])
b=np.float32(np.random.randn(3,2))
c=tf.matmul(a,b)
init=tf.global_variables_initializer()
sess=tf.Session()
print (c.eval())
上面的代码编译是错误的,显示错误如下:ValueError: Cannot evaluate tensor using `eval()`: No default session is registered. Use `with sess.as_default()` or pass an explicit session to `eval(session=sess)`
import tensorflow as tf
import numpy as np
a=tf.constant([[1., 2., 3.],[4., 5., 6.]])
b=np.float32(np.random.randn(3,2))
c=tf.matmul(a,b)
init=tf.global_variables_initializer()
sess=tf.InteractiveSession()
print (c.eval())
而用InteractiveSession()就不会出错,说白了InteractiveSession()相当于:
sess=tf.Session()
with sess.as_default():
如果说想让sess=tf.Session()起到作用,一种方法是上面的with sess.as_default();另外一种方法是
sess=tf.Session()
print (c.eval(session=sess))
其实还有一种方法也是with,如下:
import tensorflow as tf
import numpy as np
a=tf.constant([[1., 2., 3.],[4., 5., 6.]])
b=np.float32(np.random.randn(3,2))
c=tf.matmul(a,b)
init=tf.global_variables_initializer()
with tf.Session() as sess:
#print (sess.run(c))
print(c.eval())
------------------------------------------------------------------------------------------------------
参考: