REST:Representational State Transfer(表述性状态转移)
REST并不是一种创新技术,它指的是一组架构约束条件和原则。符合REST的约束条件和原则的架构,就称它为RESTful架构。
RESTful核心内容
1、资源与URI
REST为表述性状态转移,那究竟指的是什么的表述呢?其实指的是资源的表述
那什么是资源呢?在系统中任何一个事务,只要有被引用的必要,那么它就是一个资源,它可以是一段文本,可以是一张图片,甚至可以是一种服务,总之是一种真实存在的资源。要想要资源被识别,就需要有唯一标识,在Web中,这个标识就是URI,其实就是资源的地址
2、资源的表述
资源在客户端和服务器之间传送就是资源的表述
3、状态转移
资源在客户端发送变迁,然后进入后续的状态。
RESTful架构特点
- 统一了客户端访问资源的接口
- url更加简洁,易于理解,便于扩展
- 有利于不同系统之间的资源共享
RESTful具体来讲就是HTTP协议的四种形式表示四种基本操作
- GET:获取资源
- POST:新建资源
- PUT:修改资源
- DELETE:删除资源
RESTful开发风格
查询课程:http://localhost:8080/id method='get'
添加课程:http://localhost:8080/course method='post'
修改课程:http://localhost:8080/id method='put'
删除课程:http://localhost:8080/id method='delete'
下面是实例
实体类:Course
package com.lzy.entity;
public class Course {
private int id;
private String name;
private double price;
public int getId(){
return id;
}
public void setId(int id){
this.id=id;
}
public String getName(){
return name;
}
public void setName(){
this.name=name;
}
public double getPrice(){
return price;
}
public void setPrice(double price){
this.price=price;
}
}
dao层
package com.lzy.dao;
import com.lzy.entity.Course;
import org.springframework.stereotype.Repository;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
@Repository
public class CourseDao {
private Map<Integer, Course>courses = new HashMap<Integer, Course>();
/**
* 新增课程
*/
public void add(Course course){
courses.put(course.getId(),course);
}
/**
* 查询全部课程
*/
public Collection<Course>getAll(){
return courses.values();
}
/**
* 通过id查询课程
*/
public Course getById(int id){
return courses.get(id);
}
/**
* 修改课程
*/
public void update(Course course){
courses.put(course.getId(),course);
}
/**
* 删除课程
*/
public void deleteById(int id){
courses.remove(id);
}
}
控制层
package com.lzy.controller;
import com.lzy.dao.CourseDao;
import com.lzy.entity.Course;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import java.util.Map;
@Controller
public class CourseController {
@Autowired
private CourseDao courseDao;
/**
* 添加课程
*/
@PostMapping(value = "/add")
public String add(Course course){
courseDao.add(course);
return "redirect:/getAll";
}
/**
* 查询全部课程
*/
@GetMapping(value = "/getAll")
public ModelAndView getAll(){
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("index");
modelAndView.addObject("courses",courseDao.getAll());
return modelAndView;
}
/**
* 通过id查询课程
*/
@GetMapping(value = "/getById/{id}")
public ModelAndView getById(@PathVariable(value = "id")int id){
ModelAndView modelAndView = new ModelAndView();
modelAndView.setViewName("edit");
modelAndView.addObject("course",courseDao.getById(id));
return modelAndView;
}
/**
* 修改课程
*/
@PutMapping(value = "/update")
public String update(Course course){
courseDao.update(course);
return "redirect:/getAll";
}
/**
* 删除课程
*/
@DeleteMapping(value = "/delete/{id}")
public String delete(@PathVariable(value = "id") int id){
courseDao.deleteById(id);
return "redirect:/getAll";
}
}
扫描二维码关注公众号,回复:
6425526 查看本文章
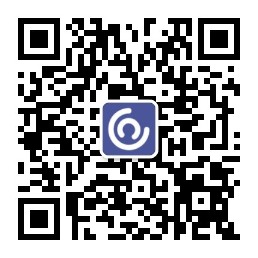