package com.jbpm.controller; import java.io.InputStream; import java.util.List; import java.util.Map; import java.util.Set; import javax.annotation.Resource; import javax.servlet.http.HttpServletRequest; import net.sf.json.JSONArray; import org.dom4j.Document; import org.jbpm.api.ExecutionService; import org.jbpm.api.HistoryService; import org.jbpm.api.ProcessDefinition; import org.jbpm.api.ProcessEngine; import org.jbpm.api.RepositoryService; import org.jbpm.api.TaskService; import org.jbpm.api.cmd.Environment; import org.jbpm.pvm.internal.env.EnvironmentFactory; import org.jbpm.pvm.internal.env.EnvironmentImpl; import org.jbpm.pvm.internal.session.RepositorySession; import org.springframework.stereotype.Controller; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.ResponseBody; import com.jbpm.service.MyDayService; import com.jbpm.workflow.service.BpmConfigParser; /** * controller�� * @author Administrator * */ @Controller @RequestMapping("flow") public class FlowController { @Resource(name="repositoryService") private RepositoryService repositoryService;//-流程管理,部署发布 @Resource(name="taskService") private TaskService taskService;//任务管理 @Resource(name="executionService") private ExecutionService executionService;//-流程实例管理 @Resource(name="myDayService") private MyDayService myDayService;//业务逻辑 @Resource(name="historyService") private HistoryService historyService;//业务逻辑 @Resource(name="jbpmParser") private BpmConfigParser jbpmParser; //jbpm解析器 @Resource(name="processEngine") private ProcessEngine processEngine; @RequestMapping("toFlow") public String toFlow(HttpServletRequest request){ return "projectFlowAdd"; } @RequestMapping("deploy") public @ResponseBody String deploy(HttpServletRequest request,String flowJson){ try{ System.out.println(flowJson); Document doc = jbpmParser.parseEditorFile(flowJson); String key = jbpmParser.getProcessKey(flowJson); String resourceName = key + ".jpdl.xml"; System.out.println(doc.asXML()+","+key); String deployId = repositoryService.createDeployment().addResourceFromString(resourceName, doc.asXML()).deploy(); }catch(Exception e){ e.printStackTrace(); } return "success"; } @RequestMapping("showFlowEdit") public String showFlowEdit(HttpServletRequest request,String flowKey){ if(flowKey == null || "".endsWith(flowKey)){ request.setAttribute("flowJsonResult", "null"); }else{ InputStream ins = this.getProcessDefCfg(flowKey); String flowName = this.getProcessDefinition(flowKey).getName(); String flowJsonResult = jbpmParser.parseBpmConfig(ins); request.setAttribute("flowJsonResult", flowJsonResult); request.setAttribute("flowKey", flowKey); request.setAttribute("flowName", flowName); } return "projectFlowEditor"; } public InputStream getProcessDefCfg(String flowKey) { EnvironmentFactory environmentFactory = (EnvironmentFactory) processEngine; Environment environment = environmentFactory.openEnvironment(); try{ RepositorySession repositorySession = environment.get(RepositorySession.class); ProcessDefinition pd = repositorySession.findProcessDefinitionByKey(flowKey); Set<String> names = repositoryService.getResourceNames(pd.getDeploymentId()); // Set<String> names = new GetDeploymentResourceNamesCmd(pd.getDeploymentId()).execute(environment); for (String n :names) { if (n.indexOf(".jpdl.xml") != -1) { InputStream in = repositoryService.getResourceAsStream(pd.getDeploymentId(), n); return in; } } }catch(Exception e){ e.printStackTrace(); }finally{ closeEnvironment(environment); } return null; } private void closeEnvironment(Environment environment){ if(environment!=null){ ((EnvironmentImpl)environment).close(); } } public ProcessDefinition getProcessDefinition(String flowKey) { EnvironmentFactory environmentFactory = (EnvironmentFactory) processEngine; Environment environment = environmentFactory.openEnvironment(); ProcessDefinition pd = null; try{ RepositorySession repositorySession = environment.get(RepositorySession.class); pd = repositorySession.findProcessDefinitionByKey(flowKey); }catch(Exception e){ e.printStackTrace(); } return pd; } }
jbpm.spring.default.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <jbpm-configuration> <process-engine-context> <repository-service /> <repository-cache /> <execution-service /> <history-service /> <management-service /> <identity-service /> <task-service /> <!-- <hibernate-configuration> <cfg resource="jbpm.hibernate.cfg.xml" /> </hibernate-configuration> <hibernate-session-factory /> --> <script-manager default-expression-language="juel" default-script-language="juel" read-contexts="execution, environment, process-engine" write-context=""> <script-language name="juel" factory="org.jbpm.pvm.internal.script.JuelScriptEngineFactory" /> </script-manager> <authentication /> <id-generator /> <types resource="jbpm.variable.types.xml" /> <address-resolver /> <business-calendar> <monday hours="9:00-12:00 and 12:30-17:00" /> <tuesday hours="9:00-12:00 and 12:30-17:00" /> <wednesday hours="9:00-12:00 and 12:30-17:00" /> <thursday hours="9:00-12:00 and 12:30-17:00" /> <friday hours="9:00-12:00 and 12:30-17:00" /> <holiday period="01/07/2008 - 31/08/2008" /> </business-calendar> <mail-template name='task-notification'> <to users="${task.assignee}" /> <subject>${task.name}</subject> <text><![CDATA[Hi ${task.assignee},Task "${task.name}" has been assigned to you. ${task.description}Sent by JBoss jBPM ]]></text> </mail-template> <mail-template name='task-reminder'> <to users="${task.assignee}" /> <subject>${task.name}</subject> <text><![CDATA[Hey ${task.assignee},Do not forget about task "${task.name}".${task.description}Sent by JBoss jBPM ]]></text> </mail-template> </process-engine-context> <transaction-context> <repository-session /> <db-session /> <message-session /> <timer-session /> <history-session /> <mail-session> <mail-server> <session-properties resource="jbpm.mail.properties" /> </mail-server> </mail-session> </transaction-context> </jbpm-configuration>
jbpm.hibernate.cfg.xml
<?xml version="1.0" encoding="utf-8"?> <!DOCTYPE hibernate-configuration PUBLIC "-//Hibernate/Hibernate Configuration DTD 3.0//EN" "http://hibernate.sourceforge.net/hibernate-configuration-3.0.dtd"> <hibernate-configuration> <session-factory> <property name="connection.driver_class">com.mysql.jdbc.Driver</property> <property name="connection.url">jdbc:mysql://localhost:3306/testjbpm</property> <property name="connection.useUnicode">true</property> <property name="connection.characterEncoding">utf-8</property> <property name="dialect">org.hibernate.dialect.MySQLInnoDBDialect</property> <!--<property name="dialect">org.hibernate.dialect.MySQLDialect</property>--> <property name="connection.username">root</property> <property name="connection.password">123456</property> <property name="show_sql">true</property> <property name="hbm2ddl.auto">update</property> <property name="formate_sql">true</property> <property name="hibernate.jdbc.batch_size">20</property> <mapping resource="jbpm.repository.hbm.xml" /> <mapping resource="jbpm.execution.hbm.xml" /> <mapping resource="jbpm.history.hbm.xml" /> <mapping resource="jbpm.task.hbm.xml" /> <mapping resource="jbpm.identity.hbm.xml" /> </session-factory> </hibernate-configuration>
扫描二维码关注公众号,回复:
641756 查看本文章
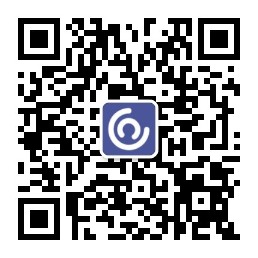
jbpm.cfg.xml
<?xml version="1.0" encoding="UTF-8"?> <jbpm-configuration> <process-engine-context> <string name="spring.cfg" value="applicationContext-core.xml" /> </process-engine-context> <import resource="jbpm.default.cfg.xml" /> <import resource="jbpm.tx.spring.cfg.xml" /> <import resource="jbpm.jpdl.cfg.xml" /> <import resource="jbpm.bpmn.cfg.xml" /> <import resource="jbpm.identity.cfg.xml" /> <import resource="jbpm.businesscalendar.cfg.xml" /> <import resource="jbpm.console.cfg.xml" /> <import resource="jbpm.spring.default.cfg.xml"/> </jbpm-configuration>
applicationContext-flow.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:task="http://www.springframework.org/schema/task" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.0.xsd"> <bean id="jbpmSpringHelper" class="org.jbpm.pvm.internal.processengine.SpringHelper" lazy-init="true" autowire="default" dependency-check="default"> <property name="jbpmCfg"> <value>jbpm.cfg.xml</value> </property> </bean> <bean id="processEngine" factory-bean="jbpmSpringHelper" factory-method="createProcessEngine" /> <bean id="repositoryService" factory-bean="processEngine" factory-method="getRepositoryService" /><!--流程管理,部署发布 --> <bean id="executionService" factory-bean="processEngine" factory-method="getExecutionService" /><!--流程实例管理 --> <bean id="taskService" factory-bean="processEngine" factory-method="getTaskService" /><!--任务管理 --> <bean id="historyService" factory-bean="processEngine" factory-method="getHistoryService" /><!--历史服务 --> <bean id="identityService" factory-bean="processEngine" factory-method="getIdentityService" /><!--身份管理 --> </beans>
applicationContext-core.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:task="http://www.springframework.org/schema/task" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.0.xsd"> <bean id="sessionFactory" class="org.springframework.orm.hibernate3.LocalSessionFactoryBean"> <property name="configLocations"> <list> <value> classpath:jbpm.hibernate.cfg.xml </value> </list> </property> </bean> <bean id="transactionManager" class="org.springframework.orm.hibernate3.HibernateTransactionManager"> <property name="sessionFactory" ref="sessionFactory" /> </bean> <bean id="transactionBese" class="org.springframework.transaction.interceptor.TransactionProxyFactoryBean" lazy-init="true"> <property name="transactionManager" ref="transactionManager" /> <property name="transactionAttributes"> <props> <prop key="add*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="update*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="save*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="insert*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="modify*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="delete*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="create*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="deploy*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="do*">PROPAGATION_REQUIRED,-Exception</prop> <prop key="*">PROPAGATION_REQUIRED,readOnly</prop> <prop key="get*">PROPAGATION_NEVER</prop> </props> </property> </bean> <bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource"> <property name="driverClassName"> <value>com.mysql.jdbc.Driver</value> </property> <property name="url"> <value>jdbc:mysql://localhost:3306/testjbpm</value> </property> <property name="username"> <value>root</value> </property> <property name="password"> <value>123456</value> </property> </bean> <bean id="simpleJdbcTemplate" class="org.springframework.jdbc.core.simple.SimpleJdbcTemplate"> <constructor-arg ref="dataSource" /> </bean> <bean id="namedParameterJdbcTemplate" class="org.springframework.jdbc.core.namedparam.NamedParameterJdbcTemplate"> <constructor-arg ref="dataSource" /> </bean> </beans>
applicationContext-bean.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:task="http://www.springframework.org/schema/task" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.0.xsd"> <context:component-scan base-package="com.jbpm"/> <mvc:annotation-driven/> </beans>