一、spring2.5整合jpa spring2.5+jpa(hibernate实现) spring2.5.6整合jpa spring2.5.6+jpa(hibernate实现)
本demo采用spring2.5.6版本,JPA的实现框架为Hibernate。
jar包来源spring-framework-2.5.6-with-dependencies.zip
hibernate-distribute-3.3.2GA-dist.rar
hibernate-annotations-3.4.0.GA.zip
本项目采用mysql数据库自行添加mysql数据库jar包
二、项目结构及jar包截图
三、根据下图结构依次贴出代码
1.UserDAO
package com.iskyshop.dao; import com.iskyshop.model.User; public interface UserDAO { public void saveUser(User user); }
2.UserDAOImpl
package com.iskyshop.dao.impl; import javax.annotation.Resource; import org.springframework.orm.jpa.JpaTemplate; import org.springframework.stereotype.Component; import com.iskyshop.dao.UserDAO; import com.iskyshop.model.User; @Component public class UserDAOImpl implements UserDAO { @Resource(name = "jt") private JpaTemplate jpaTemplate; public JpaTemplate getJpaTemplate() { return jpaTemplate; } public void setJpaTemplate(JpaTemplate jpaTemplate) { this.jpaTemplate = jpaTemplate; } @SuppressWarnings("unchecked") @Override public void saveUser(User user) { // TODO Auto-generated method stub System.out.println(jpaTemplate + "----User"); this.jpaTemplate.persist(user); } }
3.User
package com.iskyshop.model; import java.util.Date; import javax.persistence.Entity; import javax.persistence.GeneratedValue; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name = "iskyshop_user") public class User { @Id @GeneratedValue private Long id; private String username; private String password; private Date addTime; public Date getAddTime() { return addTime; } public void setAddTime(Date addTime) { this.addTime = addTime; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public Long getId() { return id; } public void setId(Long id) { this.id = id; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } }
4.UserService
扫描二维码关注公众号,回复:
638422 查看本文章
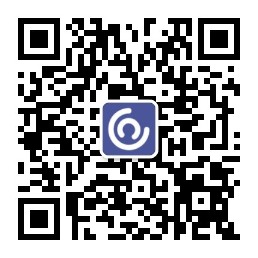
package com.iskyshop.service; import com.iskyshop.model.User; public interface UserService { public void saveUser(User user); }
5.UserServiceImpl
package com.iskyshop.service.impl; import javax.annotation.Resource; import org.springframework.stereotype.Service; import org.springframework.transaction.annotation.Transactional; import com.iskyshop.dao.UserDAO; import com.iskyshop.model.User; import com.iskyshop.service.UserService; @Service @Transactional public class UserServiceImpl implements UserService{ @Resource private UserDAO userDAO; @Override public void saveUser(User user) { // TODO Auto-generated method stub userDAO.saveUser(user); } }
6.Test
package com.iskyshop.test; import java.sql.SQLException; import java.util.Date; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.iskyshop.model.User; import com.iskyshop.service.UserService; public class Test { /** * @param ISkyShop java多用户商城(国内首家真正好用的java c2c商城系统) * 采用springmvc+jpa+velocity * 免费下载地址:www.iskyshop.com * @throws SQLException */ public static void main(String[] args) throws SQLException { // TODO Auto-generated method stub ApplicationContext ctx = new ClassPathXmlApplicationContext("beans.xml"); UserService userService = (UserService) ctx.getBean("userServiceImpl"); User user = new User(); user.setUsername("iskyshop-java多用户商城"); user.setPassword("www.iskyshop.com"); user.setAddTime(new Date()); userService.saveUser(user); } }
7.beans.xml
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:tx="http://www.springframework.org/schema/tx" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-2.5.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-2.5.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-2.5.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-2.5.xsd"> <context:annotation-config /> <context:component-scan base-package="com.iskyshop" /> <!-- 配置数据源 --> <bean class="org.springframework.beans.factory.config.PropertyPlaceholderConfigurer"> <property name="locations"> <value>classpath:jdbc.properties</value> </property> </bean> <bean id="dataSource" destroy-method="close" class="org.apache.commons.dbcp.BasicDataSource"> <property name="driverClassName" value="${jdbc.driverClassName}" /> <property name="url" value="${jdbc.url}" /> <property name="username" value="${jdbc.username}" /> <property name="password" value="${jdbc.password}" /> <property name="initialSize" value="${initialSize}" /> <property name="maxActive" value="${maxActive}" /> <property name="maxIdle" value="${maxIdle}" /> <property name="minIdle" value="${minIdle}" /> </bean> <bean id="entityManagerFactory" class="org.springframework.orm.jpa.LocalContainerEntityManagerFactoryBean"> <property name="dataSource" ref="dataSource" /> <property name="persistenceXmlLocation" value="classpath:persistence.xml" /> <property name="jpaProperties"> <props> <prop key="hibernate.dialect">org.hibernate.dialect.MySQL5Dialect</prop> <prop key="hibernate.hbm2ddl.auto">create</prop> <prop key="hibernate.show_sql">true</prop> <prop key="hibernate.format_sql">true</prop> <prop key="javax.persistence.validation.mode">none</prop> </props> </property> <!-- 用于设置JPA实现厂商的特定属性 --> <property name="jpaVendorAdapter"> <bean class="org.springframework.orm.jpa.vendor.HibernateJpaVendorAdapter"> <property name="showSql" value="true" /> <property name="generateDdl" value="true" /> <property name="database" value="MYSQL" /> </bean> </property> </bean> <bean id="jpaTxManager" class="org.springframework.orm.jpa.JpaTransactionManager"> <property name="entityManagerFactory" ref="entityManagerFactory" /> <property name="dataSource" ref="dataSource" /> </bean> <tx:annotation-driven transaction-manager="jpaTxManager" /> <bean id="jt" class="org.springframework.orm.jpa.JpaTemplate"> <property name="entityManagerFactory" ref="entityManagerFactory"> </property> </bean> </beans>
8.jdbc.properties
jdbc.driverClassName=org.gjt.mm.mysql.Driver jdbc.url=jdbc:mysql://localhost:3306/mysql?createDatabaseIfNotExist=true&useUnicode=true&characterEncoding=utf-8 jdbc.username=root jdbc.password=123456 initialSize=1 maxActive=500 maxIdle=2 minIdle=1
9.persistence.xml
<persistence xmlns="http://java.sun.com/xml/ns/persistence" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/persistence http://java.sun.com/xml/ns/persistence/persistence_1_0.xsd" version="1.0"> <persistence-unit name="springjpa" transaction-type="RESOURCE_LOCAL"> <provider>org.hibernate.ejb.HibernatePersistence</provider> </persistence-unit> </persistence>