在使用文件进行交互数据的应用来说,使用FTP服务器是一个很好的选择。本文使用Apache Jakarta Commons Net(commons-net-3.3.jar) 基于FileZilla Server服务器实现FTP服务器上文件的上传/下载/删除等操作。
关于FileZilla Server服务器的详细搭建配置过程,详情请见 FileZilla Server安装配置教程 。之前有朋友说,上传大文件(几百M以上的文件)到FTP服务器时会重现无法重命名的问题,但本人亲测上传2G的文件到FileZilla Server都没有该问题,朋友们可以放心使用该代码。
FavFTPUtil.Java
packagecom.favccxx.favsoft.util;
importjava.io.File;
importjava.io.FileInputStream;
importjava.io.FileOutputStream;
importjava.io.IOException;
importjava.io.InputStream;
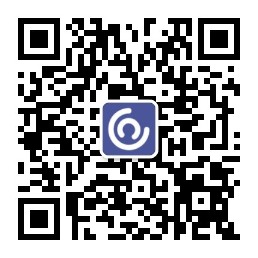
importjava.io.OutputStream;
importorg.apache.commons.net.ftp.FTPClient;
importorg.apache.commons.net.ftp.FTPFile;
importorg.apache.commons.net.ftp.FTPReply;
publicclassFavFTPUtil {
/**
* 上传文件(可供Action/Controller层使用)
* @param hostname FTP服务器地址
* @param port FTP服务器端口号
* @param username FTP登录帐号
* @param password FTP登录密码
* @param pathname FTP服务器保存目录
* @param fileName 上传到FTP服务器后的文件名称
* @param inputStream 输入文件流
* @return
*/
publicstaticbooleanuploadFile(String hostname, intport, String username, String password, String pathname, String fileName, InputStream inputStream){
booleanflag = false;
FTPClient ftpClient = newFTPClient();
ftpClient.setControlEncoding("UTF-8");
try{
//连接FTP服务器
ftpClient.connect(hostname, port);
//登录FTP服务器
ftpClient.login(username, password);
//是否成功登录FTP服务器
intreplyCode = ftpClient.getReplyCode();
if(!FTPReply.isPositiveCompletion(replyCode)){
returnflag;
}
ftpClient.setFileType(FTPClient.BINARY_FILE_TYPE);
ftpClient.makeDirectory(pathname);
ftpClient.changeWorkingDirectory(pathname);
ftpClient.storeFile(fileName, inputStream);
inputStream.close();
ftpClient.logout();
flag = true;
} catch(Exception e) {
e.printStackTrace();
} finally{
if(ftpClient.isConnected()){
try{
ftpClient.disconnect();
} catch(IOException e) {
e.printStackTrace();
}
}
}
returnflag;
}
/**
* 上传文件(可对文件进行重命名)
* @param hostname FTP服务器地址
* @param port FTP服务器端口号
* @param username FTP登录帐号
* @param password FTP登录密码
* @param pathname FTP服务器保存目录
* @param filename 上传到FTP服务器后的文件名称
* @param originfilename 待上传文件的名称(绝对地址)
* @return
*/
publicstaticbooleanuploadFileFromProduction(String hostname, intport, String username, String password, String pathname, String filename, String originfilename){
booleanflag = false;
try{
InputStream inputStream = newFileInputStream(newFile(originfilename));
flag = uploadFile(hostname, port, username, password, pathname, filename, inputStream);
} catch(Exception e) {
e.printStackTrace();
}
returnflag;
}
/**
* 上传文件(不可以进行文件的重命名操作)
* @param hostname FTP服务器地址
* @param port FTP服务器端口号
* @param username FTP登录帐号
* @param password FTP登录密码
* @param pathname FTP服务器保存目录
* @param originfilename 待上传文件的名称(绝对地址)
* @return
*/
publicstaticbooleanuploadFileFromProduction(String hostname, intport, String username, String password, String pathname, String originfilename){
booleanflag = false;
try{
String fileName = newFile(originfilename).getName();
InputStream inputStream = newFileInputStream(newFile(originfilename));
flag = uploadFile(hostname, port, username, password, pathname, fileName, inputStream);
} catch(Exception e) {
e.printStackTrace();
}
returnflag;
}
/**
* 删除文件
* @param hostname FTP服务器地址
* @param port FTP服务器端口号
* @param username FTP登录帐号
* @param password FTP登录密码
* @param pathname FTP服务器保存目录
* @param filename 要删除的文件名称
* @return
*/
publicstaticbooleandeleteFile(String hostname, intport, String username, String password, String pathname, String filename){
booleanflag = false;
FTPClient ftpClient = newFTPClient();
try{
//连接FTP服务器
ftpClient.connect(hostname, port);
//登录FTP服务器
ftpClient.login(username, password);
//验证FTP服务器是否登录成功
intreplyCode = ftpClient.getReplyCode();
if(!FTPReply.isPositiveCompletion(replyCode)){
returnflag;
}
//切换FTP目录
ftpClient.changeWorkingDirectory(pathname);
ftpClient.dele(filename);
ftpClient.logout();
flag = true;
} catch(Exception e) {
e.printStackTrace();
} finally{
if(ftpClient.isConnected()){
try{
ftpClient.logout();
} catch(IOException e) {
}
}
}
returnflag;
}
/**
* 下载文件
* @param hostname FTP服务器地址
* @param port FTP服务器端口号
* @param username FTP登录帐号
* @param password FTP登录密码
* @param pathname FTP服务器文件目录
* @param filename 文件名称
* @param localpath 下载后的文件路径
* @return
*/
publicstaticbooleandownloadFile(String hostname, intport, String username, String password, String pathname, String filename, String localpath){
booleanflag = false;
FTPClient ftpClient = newFTPClient();
try{
//连接FTP服务器
ftpClient.connect(hostname, port);
//登录FTP服务器
ftpClient.login(username, password);
//验证FTP服务器是否登录成功
intreplyCode = ftpClient.getReplyCode();
if(!FTPReply.isPositiveCompletion(replyCode)){
returnflag;
}
//切换FTP目录
ftpClient.changeWorkingDirectory(pathname);
FTPFile[] ftpFiles = ftpClient.listFiles();
for(FTPFile file : ftpFiles){
if(filename.equalsIgnoreCase(file.getName())){
File localFile = newFile(localpath + "/"+ file.getName());
OutputStream os = newFileOutputStream(localFile);
ftpClient.retrieveFile(file.getName(), os);
os.close();
}
}
ftpClient.logout();
flag = true;
} catch(Exception e) {
e.printStackTrace();
} finally{
if(ftpClient.isConnected()){
try{
ftpClient.logout();
} catch(IOException e) {
}
}
}
returnflag;
}
}
FavFTPUtilTest.java
packagecom.favccxx.favsoft.util;
importjunit.framework.TestCase;
publicclassFavFTPTest extendsTestCase {
publicvoidtestFavFTPUtil(){
String hostname = "127.0.0.1";
intport = 21;
String username = "business";
String password = "business";
String pathname = "business/ebook";
String filename = "big.rar";
String originfilename = "C:\\Users\\Downloads\\Downloads.rar";
FavFTPUtil.uploadFileFromProduction(hostname, port, username, password, pathname, filename, originfilename);
// String localpath = "D:/";
// FavFTPUtil.downloadFile(hostname, port, username, password, pathname, filename, localpath);
}
}