一、相关说明
IOC:
Inversion of Control,控制反转,是面向对象编程中的一种设计原则,可以用来减低计算机代码之间的耦合度。其中最常见的方式叫做依赖注入(Dependency Injection,简称DI)。通过控制反转,对象在被创建的时候,由一个调控系统内所有对象的外界实体将其所依赖的对象的引用传递给它。也可以说,依赖被注入到对象中。简单地说就是拿到的对象的属性,已经被注入好相关值了,直接使用即可。
AOP
在软件业,AOP为Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术。
Spring是一个基于IOC和AOP的结构J2EE系统的框架
二、配置Spring
1、新建java类型项目
2、导入jar包
现在Spring是放在github上托管,下载步骤如下:
a、进入spring官网点击project
b、springfarmework
c、点右边github标志
d、底部的artifacts
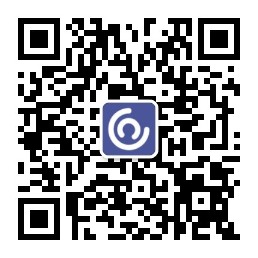
e、Spring Artifactory
f、三个版本,一般选第三个,稳定些
g、依次选择org/springfarmework/spring,然后选择需要的版本
3、将包导入到项目中
4、在src下建包,新建一个Category类
package com.yyt.pojo;
public class Category {
private int id; private String name; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
5、在src目录下新建applicationContext.xml文件
applicationContext.xml是Spring的核心配置文件,通过关键字c即可获取Category对象,该对象获取的时候,即被注入了字符串"category 1“到name属性中
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <bean name="c" class="com.yyt.pojo.Category"> <property name="name" value="category 1" /> </bean> </beans>
6、test类
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.how2java.pojo.Category; public class Test { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext( new String[] { "applicationContext.xml" }); Category c = (Category) context.getBean("c"); System.out.println(c.getName()); } }
三、原理
传统的方式:
通过new 关键字主动创建一个对象
IOC方式
对象的生命周期由Spring来管理,直接从Spring那里去获取一个对象。 IOC是反转控制 (Inversion Of Control)的缩写,就像控制权从本来在自己手里,交给了Spring。
注:基于框架的程序要成功运行,对于JAR包的版本,配置文件的正确性有着苛刻的要求,任何一个地方出错了,都会导致框架程序运行失败。
一、相关说明
IOC:
Inversion of Control,控制反转,是面向对象编程中的一种设计原则,可以用来减低计算机代码之间的耦合度。其中最常见的方式叫做依赖注入(Dependency Injection,简称DI)。通过控制反转,对象在被创建的时候,由一个调控系统内所有对象的外界实体将其所依赖的对象的引用传递给它。也可以说,依赖被注入到对象中。简单地说就是拿到的对象的属性,已经被注入好相关值了,直接使用即可。
AOP
在软件业,AOP为Aspect Oriented Programming的缩写,意为:面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术。
Spring是一个基于IOC和AOP的结构J2EE系统的框架
二、配置Spring
1、新建java类型项目
2、导入jar包
现在Spring是放在github上托管,下载步骤如下:
a、进入spring官网点击project
b、springfarmework
c、点右边github标志
d、底部的artifacts
e、Spring Artifactory
f、三个版本,一般选第三个,稳定些
g、依次选择org/springfarmework/spring,然后选择需要的版本
3、将包导入到项目中
4、在src下建包,新建一个Category类
package com.yyt.pojo;
public class Category {
private int id; private String name; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
5、在src目录下新建applicationContext.xml文件
applicationContext.xml是Spring的核心配置文件,通过关键字c即可获取Category对象,该对象获取的时候,即被注入了字符串"category 1“到name属性中
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation=" http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.0.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <bean name="c" class="com.yyt.pojo.Category"> <property name="name" value="category 1" /> </bean> </beans>
6、test类
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import com.how2java.pojo.Category; public class Test { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext( new String[] { "applicationContext.xml" }); Category c = (Category) context.getBean("c"); System.out.println(c.getName()); } }
三、原理
传统的方式:
通过new 关键字主动创建一个对象
IOC方式
对象的生命周期由Spring来管理,直接从Spring那里去获取一个对象。 IOC是反转控制 (Inversion Of Control)的缩写,就像控制权从本来在自己手里,交给了Spring。
注:基于框架的程序要成功运行,对于JAR包的版本,配置文件的正确性有着苛刻的要求,任何一个地方出错了,都会导致框架程序运行失败。