1,前面博文面的单链表实现中运用了大量的原生指针,这里能否使用智能指针(SmartPointer)替换单链表(LinkList)中的原生指针?
1,不能简单的替换,程序会崩溃;
2,问题在于:
1,SmartPointer 的设计方案:
1,指针生命周期结束时主动释放堆空间;
2,一片堆空间最多只能由一个指针标识;
1,这个就是问题所在,因为在 LinkList 中遍历的时候将多个指针指向同一个元素;
3,杜绝指针运算和指针比较;
3,新的设计方案:
扫描二维码关注公众号,回复:
6271990 查看本文章
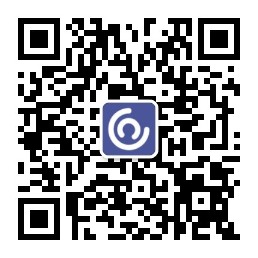
1,创建新的智能指针:
1,Pointer 是智能指针的抽象父类(模板):
1,纯虚析构函数 virtual ~Pointer() = 0;
1,因为 Pointer 是抽象父类,需要被继承产生正正的指针;
2,重载 operator->();
3,重载 operator*();
2,Pointer 的接口定义:
4,Pointer 的实现:
1 #ifndef POINTER_H 2 #define POINTER_H 3 4 #include "Object.h" 5 6 namespace DTLib 7 { 8 9 template <typename T> 10 class Pointer : public Object 11 { 12 protected: 13 T* m_pointer; 14 15 public: 16 Pointer( T* p = NULL ) 17 { 18 m_pointer = p; 19 } 20 21 T* operator-> () 22 { 23 return m_pointer; 24 } 25 26 T& operator* () 27 { 28 return *m_pointer; 29 } 30 31 const T* operator-> () const // 为了 const 函数调用而准备 32 { 33 return m_pointer; 34 } 35 36 const T& operator* () const // 为了 const 函数调用而准备 37 { 38 return *m_pointer; 39 } 40 41 bool isNull() const // 做成 const 函数是为了被 const 函数调用 42 { 43 return ( m_pointer == NULL ); 44 } 45 46 T* get() const // 做成 const 函数是为了被 const 函数调用 47 { 48 return m_pointer; 49 } 50 51 }; 52 53 }
6,SmartPointer 的实现(重新架构):
1 #ifndef SMARTPOINTER_H 2 #define SMARTPOINTER_H 3 4 #include "Pointer.h" 5 6 namespace DTLib 7 { 8 9 template <typename T> 10 class SmartPointer : public Pointer<T> 11 { 12 public: 13 SmartPointer(T* p = NULL) : Pointer<T>(p) 14 { 15 } 16 17 SmartPointer(const SmartPointer<T>& obj) // 这里为了一片堆空间只能由一个指//针标识; 18 { 19 this->m_pointer = obj.m_pointer; 20 const_cast<SmartPointer<T>&>(obj).m_pointer = NULL; // 去除常量属性、然后置空; 21 } 22 23 SmartPointer<T>& operator= (const SmartPointer<T>& obj) 24 { 25 if( this != &obj) 26 { 27 T* p = this->m_pointer; // 改成异常安全的,之前是 delete m_pointer; 28 this->m_pointer = obj.m_pointer; 29 const_cast<SmartPointer<T>&>(obj).m_pointer = NULL; 30 31 delete p; 32 } 33 34 return *this; 35 } 36 37 ~SmartPointer() // 必须要,否则这里是纯虚类; 38 { 39 delete this->m_pointer; 40 } 41 }; 42 43 } 44 45 #endif // SMARTPOINTER_H