Given a singly linked list, group all odd nodes together followed by the even nodes. Please note here we are talking about the node number and not the value in the nodes.
You should try to do it in place. The program should run in O(1) space complexity and O(nodes) time complexity.
Example 1:
Input:1->2->3->4->5->NULL
Output:1->3->5->2->4->NULL
Example 2:
Input: 2->1->3->5->6->4->7->NULL
Output:2->3->6->7->1->5->4->NULL
Note:
- The relative order inside both the even and odd groups should remain as it was in the input.
- The first node is considered odd, the second node even and so on ...
题目大意:
给定一个单链表,将奇数序号的节点连接在一起,将偶数序号的节点连接在一起,将偶数序号的节点放在奇数节点后面。
解法:
之前想的是将链表中的数字记录下来,但是这样空间复杂度就不是O(1)了,首先将链表奇数节点和偶数节点分成两个链表,再将这两个链表连接在一起。
java:
class Solution { public ListNode oddEvenList(ListNode head) { if (head==null||head.next==null||head.next.next==null) return head; ListNode pre=head,cur=head.next; ListNode node=head.next; while(cur!=null){ ListNode next=cur.next; pre.next=next; pre=cur; cur=next; } ListNode tail=head; while (tail.next!=null) tail=tail.next; tail.next=node; return head; } }
代码优化过后的java:
扫描二维码关注公众号,回复:
6263563 查看本文章
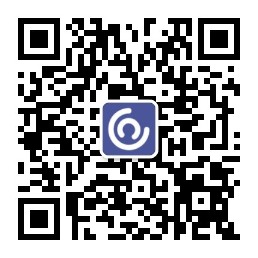
这里使用了两个指针将链表分成奇数节点链表和偶数节点链表。
class Solution { public ListNode oddEvenList(ListNode head) { if (head!=null){ ListNode odd=head,even=head.next,evenHead=head.next; while(even!=null && even.next!=null){ odd.next=odd.next.next; even.next=even.next.next; odd=odd.next; even=even.next; } odd.next=evenHead; } return head; } }