参数默认值
也就是说现在ES6对函数中的参数添加了默认值
我们在ES5种的处理
function Fn(a, b) {
b = b || "nodeing";
return a + b
}
console.log(Fn("hello"))
这样写有一个缺点就是当我传入b为一个空字符串的时候,返回的结果并不是我想要的结果,正确的输出结果应该是hello ,但是因为空字符串会被转换成false, b = '' "|| "nodeing",所以最终输出的结果会是“hellonodeing“,因此,我们还需要去判断b有没有值
function Fn(a, b) {
if(typeof b === "undefined"){
b = "nodeing"
}
return a + b
}
console.log(Fn("hello"))
在es6中就没怎么麻烦了,可以直接定义一下就OK了
function Fn(a, b = "nodeing"){
return "nodeing"
}
rest参数
n(...参数名),这种形式的参数叫做rest参数,作用就是获取函数多余的参数,这样就不需要arguments对象了
function Fn(...arg){
for(var i = 0; i < arg.length; i++){
console.log(arg[i])
}
}
Fn(1,3,4,5,6)
在ES6的数组中也有这个扩展运算符,但是他的作用是将数组转为相对应的数字,并且最不相同的点就是就是数组代表的是一个区间的,而函数参数和解析赋值的时候使用扩展运算符的时候子代表剩余,所以只可以放最后,而数组中的扩展扩展运算符可以放在任意位置
使用rest参数和普通参数混用的时候需要注意的是一一对应关系
function Fn(a,b,...arg){
console.log(a,b,arg)
}
Fn(1,2,4,5,6)// 1传给a 2 传给b, 4、6、6传给arg,arg是一个数组
还应该注意的一点是rest参数必须放在最后
function Fn(...arg,a,b){} //错误
.箭头函数
在es6中,定义函数可以使用箭头(=>)的形式
let f = n => n*2
这种写法等价于
let f = function(n){
return n * 2
}
上面函数只是传入了一个参数,如果有多个参数的时候,需要写成这样
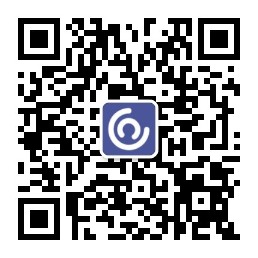
let f = (a, b) => a + b;
等价于
let f = function(a,b){return a + b}
如果函数体有多条语句的时候写成这样
let f = (a, b) => {
if(a>b){
console.log(a)
}else{
console.log(b)
}
}
如果没有参数的时候,括号不能省略
let f = () => console.log(1)
f()
//f = => console.log(1) 如果写成这样会报错
this问题
在ES5中this:执行函数的上下文
在ES6中this:定义函数的上下文
看例子
1)箭头函数没有自己的this,函数体内的this对象,就是定义时所在的对象,而不是使用时所在的对象。
document.onclick = ()=>{
console.log(this) // window
};
上面代码中调用后面箭头函数的是document对象 但是指向的却是window,因为箭头函数在window环境下定义的
document.onclick = function () {
console.log(this) //document
let f = ()=>{
console.log(this) // document
};
f();
}
上面代码中箭头函数里的this指向的是document,原因是箭头函数是在事件处理函数function(){}中调用的,在onclick后面的匿名函数function中this是指向document的,所以内层的箭头函数里的this和外层函数的this都会指向document
再举一个例子:
document.onclick = ()=>{
console.log(this) //window
};
function fn() {
setInterval(()=>{console.log(this)}, 1000) //document
}
// fn()
document.onclick = fn;
上面代码中,setInterval中的匿名函数是在外层的fn环境下定义的,外层fn中的this是指向document,所有箭头函数中的this也指向document (2)不可以当作构造函数,也就是说,不可以使用new命令,否则会抛出一个错误。
let F = ()=>{}
let f1 = new F() //报错
(3)不可以使用arguments对象,该对象在函数体内不存在。如果要用,可以用 rest 参数代替。
let f = ()=>{console.log(arguments)}
f() //报错
(4)不可以使用yield命令,因此箭头函数不能用作 Generator 函数